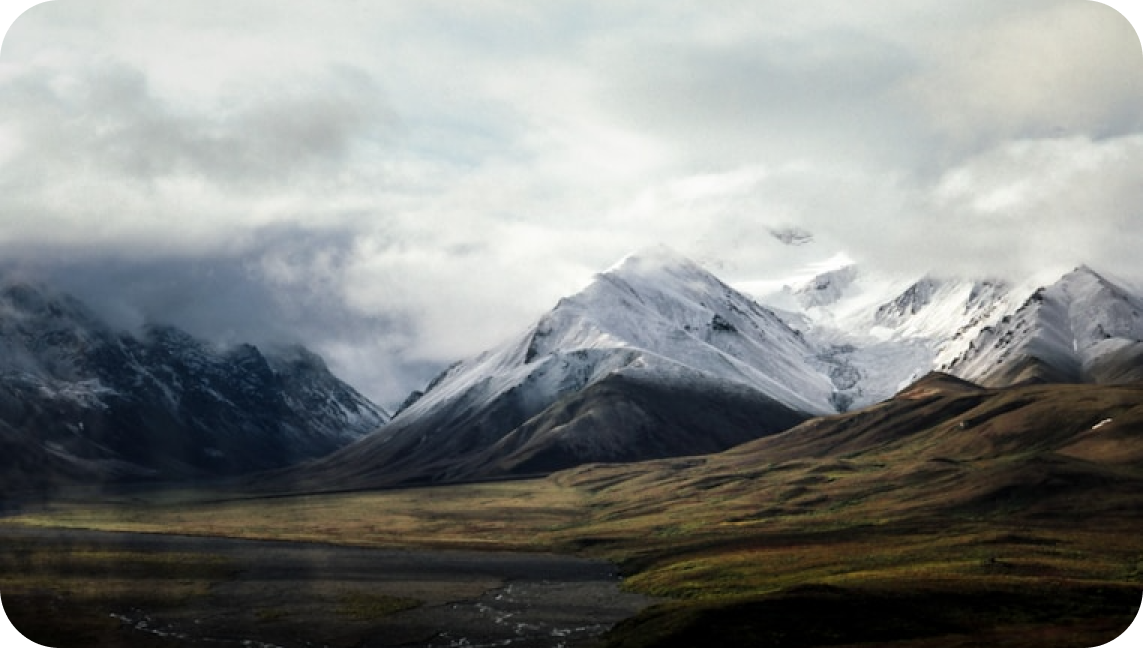
Hey, Python enthusiasts! Today, let's talk about a magical tool in the Python world - virtual environments. Are you often troubled by conflicting dependencies between different projects? Or unable to run code due to inconsistent environments during team collaboration? Don't worry, virtual environments were born to solve these problems! Let's dive deep into this powerful tool and see how it can make your Python development journey smoother.
What is a Virtual Environment
Simply put, a Python virtual environment is an independent, isolated Python runtime environment. It's like creating a dedicated room for your project, with its own Python interpreter and dependency libraries, completely isolated from other projects and the system environment. This way, you can use different versions of Python and dependency libraries in different projects without worrying about them interfering with each other.
Imagine you're a chef, and each project is a dish. A virtual environment is like preparing separate kitchens and ingredients for each dish. This way, you can use fresh tomatoes to make pasta in one kitchen, while using pickled tomatoes to make pizza in another kitchen, without interference. Doesn't that sound great?
Why Do We Need Virtual Environments
You might ask, why should I bother? Can't I just install all dependencies in the system environment? Let me give you an example:
Suppose you're simultaneously developing two projects: one using Django 3.2 and another using Django 4.1. If you install all dependencies in the system environment, these two projects won't run properly due to Django version conflicts. This is where virtual environments come in handy!
With virtual environments, you can create separate environments for each project, one installing Django 3.2 and the other installing Django 4.1. This way, the two projects can coexist peacefully without interference. Isn't that cool?
Besides this, virtual environments have many other advantages:
- Project dependency isolation: Each project has its own set of dependency libraries, avoiding version conflicts.
- Improved portability: You can easily package your project along with its virtual environment and run it on other machines without worrying about environmental differences.
- Enhanced reproducibility: By recording the dependency versions in the virtual environment, you can ensure that the project runs in the same way on different machines.
- Protecting the system environment: Avoid project dependencies polluting the system environment, keeping your system clean and stable.
How to Create and Use Virtual Environments
Alright, after talking about so many benefits, you must be eager to try it out, right? Don't rush, let me guide you step by step on how to create and use virtual environments.
Creating a Virtual Environment
Python 3.3 and above versions have the venv
module built-in, so we'll use it to create a virtual environment. Open the terminal and enter the following command:
python3 -m venv myproject_env
This command will create a folder named myproject_env
in the current directory, containing the Python interpreter, pip, and other tools.
Activating the Virtual Environment
After creating the virtual environment, we need to activate it. The activation method varies depending on the operating system:
-
On Linux or macOS:
bash source myproject_env/bin/activate
-
On Windows:
bash myproject_env\Scripts\activate
After activation, you'll see (myproject_env)
appear before the command prompt, indicating that you've entered the virtual environment.
Installing Dependency Libraries
Now you can install the dependency libraries needed for your project in the virtual environment. For example, to install Django 3.2, you can run:
pip install django==3.2
Saving the Dependency List
To facilitate project sharing and rebuilding, we can save all the dependency libraries and their versions in the current environment to a file:
pip freeze > requirements.txt
This command will create a requirements.txt
file listing all installed libraries and their versions.
Rebuilding the Virtual Environment
If you want to rebuild this environment on another machine, you just need to create a new virtual environment and then run:
pip install -r requirements.txt
This will install all the dependency libraries listed in requirements.txt
.
Exiting the Virtual Environment
When you've finished your work, you can exit the virtual environment with the following command:
deactivate
Best Practices for Virtual Environments
When using virtual environments, there are some best practices that can make your development process smoother:
- Create separate virtual environments for each project. This is the core principle of using virtual environments.
- Use meaningful names for virtual environments, like
project_name_env
, so you can easily distinguish between different project environments. - Regularly update the
requirements.txt
file to ensure it reflects the latest state of the current environment. - Don't add the virtual environment folder to the version control system. Only commit the
requirements.txt
file. - Use exact version numbers in
requirements.txt
, likedjango==3.2.4
, instead ofdjango>=3.2
. This ensures consistency in the environment. - Always make sure you've activated the correct virtual environment before performing project-related operations.
Common Problems and Solutions
Even experienced developers may encounter some problems when using virtual environments. Let's look at some common issues and their solutions:
1. Forgetting to Activate the Virtual Environment
This is a very common problem. If you forget to activate the virtual environment before starting work, you might end up installing dependency libraries in the global environment or using the wrong version of libraries.
Solution: Develop a habit of checking if the virtual environment is activated before starting work. You can check if the virtual environment name is included in the command prompt, or run which python
(on Unix systems) to confirm the location of the current Python interpreter.
2. Dependency Library Conflicts
Even when using virtual environments, you might still encounter dependency library conflicts, especially when you're using multiple interdependent libraries.
Solution: Carefully check the dependencies, try upgrading or downgrading certain library versions. Use the pip show <package_name>
command to view detailed information about a package, including its dependencies. If the problem persists, consider using tools like pipdeptree
to visualize the dependency tree, helping you find the source of the conflict.
3. Choosing Virtual Environment Management Tools
Besides venv
, there are other virtual environment management tools like virtualenv
, conda
, etc. Which one is the best to choose?
Solution: This depends on your specific needs. venv
is part of the Python standard library and is sufficient for most projects. If you need to manage different versions of Python interpreters or handle non-Python dependencies, then conda
might be a better choice. My suggestion is to start with venv
, and consider other tools when you find it can't meet your needs.
4. Migrating Projects Between Different Operating Systems
Sometimes, you might need to migrate your project between Windows and Linux/macOS. You might encounter issues like different path separators or inconsistent behavior of certain libraries on different systems.
Solution:
- Use Python's os.path
module to handle file paths, which can automatically handle path separators on different operating systems.
- Use platform_system
markers in requirements.txt
to specify that certain libraries should only be installed on specific operating systems. For example:
numpy==1.21.0
pywin32==301; sys_platform == 'win32'
- For code that only runs on specific operating systems, use conditional statements for encapsulation:
import sys
if sys.platform.startswith('win'):
# Windows-specific code
elif sys.platform.startswith('linux'):
# Linux-specific code
elif sys.platform.startswith('darwin'):
# macOS-specific code
5. Virtual Environments Occupying Too Much Disk Space
If you create many virtual environments, you might find that they occupy a large amount of disk space.
Solution:
- Regularly clean up virtual environments that are no longer in use.
- Use the --without-pip
option to create virtual environments, then manually install pip, which can save some space:
python3 -m venv --without-pip myenv
source myenv/bin/activate
curl https://bootstrap.pypa.io/get-pip.py | python
- Consider using tools like
pyenv
to manage Python versions, which can allow multiple virtual environments to share the same Python installation, thereby saving space.
Advanced Techniques for Virtual Environments
After mastering the basics, let's look at some advanced techniques that can make your use of virtual environments even more proficient:
1. Using virtualenvwrapper
virtualenvwrapper
is a very useful virtual environment management tool that provides a series of commands to simplify the creation, switching, and management of virtual environments.
Installation:
pip install virtualenvwrapper
Usage:
mkvirtualenv myproject # Create and activate a new virtual environment
workon myproject # Switch to the specified virtual environment
deactivate # Exit the current virtual environment
rmvirtualenv myproject # Delete the specified virtual environment
2. Using Virtual Environments in Jupyter Notebook
If you frequently use Jupyter Notebook, you might want to use virtual environments in notebooks. Here's a little trick:
- Activate your virtual environment
- Install ipykernel:
pip install ipykernel
- Create a kernel for the virtual environment:
python -m ipykernel install --user --name=myprojectenv
Now, when you open Jupyter Notebook, you can see and select your virtual environment in the kernel list.
3. Using .env Files to Manage Environment Variables
Sometimes, you might need to set some environment variables for your project. A good practice is to use .env
files to manage these variables.
- Install python-dotenv:
pip install python-dotenv
- Create a
.env
file in the project root directory and add environment variables:
DEBUG=True
DATABASE_URL=postgresql://user:pass@localhost/dbname
- In your Python code, you can load these environment variables like this:
from dotenv import load_dotenv
import os
load_dotenv()
debug = os.getenv('DEBUG')
database_url = os.getenv('DATABASE_URL')
Remember to add the .env
file to .gitignore
to avoid committing sensitive information to the version control system.
4. Using pip-tools to Manage Dependencies
pip-tools
is a powerful dependency management tool that can help you generate and synchronize requirements.txt
files.
Installation:
pip install pip-tools
Usage:
1. Create a requirements.in
file listing your top-level dependencies:
django
requests
- Run
pip-compile
to generaterequirements.txt
:
pip-compile requirements.in
- Use
pip-sync
to install/uninstall packages, making your environment exactly matchrequirements.txt
:
pip-sync
This tool can help you better manage dependencies, especially when dealing with complex dependency relationships.
5. Using tox for Multi-Environment Testing
If you need to test your project under multiple Python versions or different environment configurations, tox
is a very useful tool.
Installation:
pip install tox
Usage:
1. Create a tox.ini
file in the project root directory:
[tox]
envlist = py36,py37,py38
[testenv]
deps = pytest
commands = pytest
- Run tox:
tox
This will create virtual environments under different Python versions, install dependencies, and run tests.
Summary
Virtual environments are an indispensable tool in Python development, effectively managing project dependencies and improving project portability and reproducibility. Through this article, we've deeply explored the concept of virtual environments, methods of creation and use, best practices, and some advanced techniques.
Remember, the key to using virtual environments is to create separate environments for each project and develop the habit of activating the correct environment. At the same time, make good use of the requirements.txt
file to manage and share dependencies, which will greatly improve your development efficiency and project maintainability.
Using virtual environments may take some time to get used to, but once mastered, you'll find it so powerful and convenient. It not only helps you avoid the troubles of dependency conflicts but also keeps your development environment clean and orderly.
Finally, I want to say that technology is constantly advancing, and tools and best practices related to virtual environments are constantly evolving. Maintain an attitude of learning and exploration, constantly try new tools and methods, and you'll find that the path of Python development will become smoother and smoother.
So, are you ready to start your virtual environment journey? Come on, create your first virtual environment and start a brand new Python development experience!
Next
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.
Next
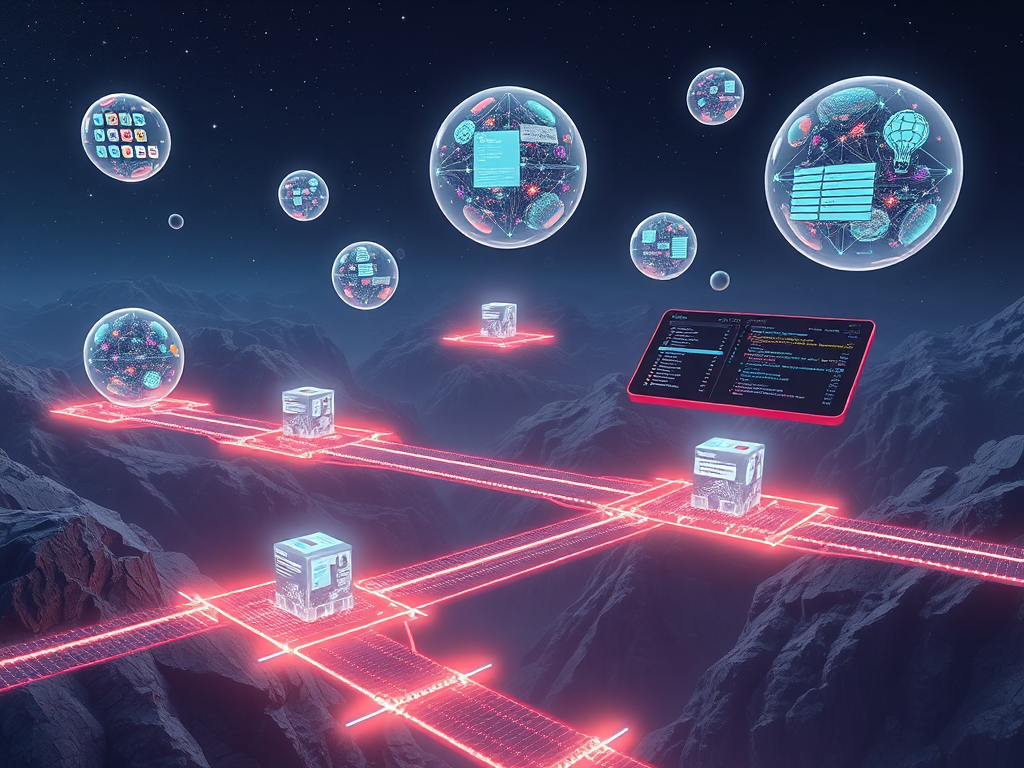
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
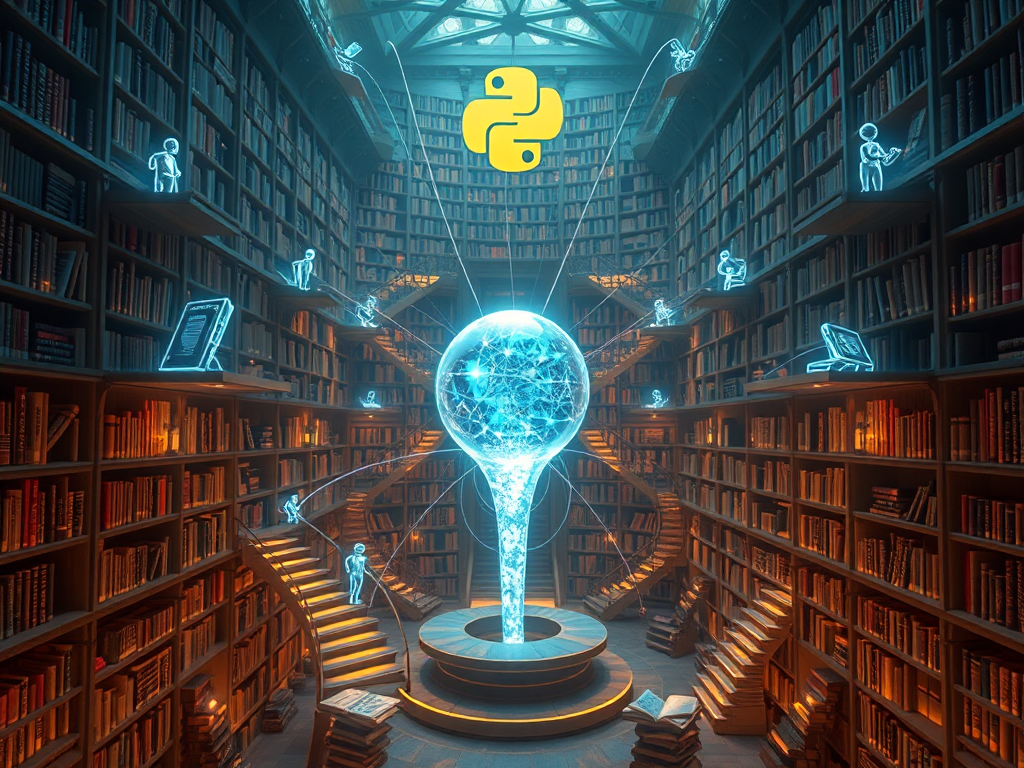
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
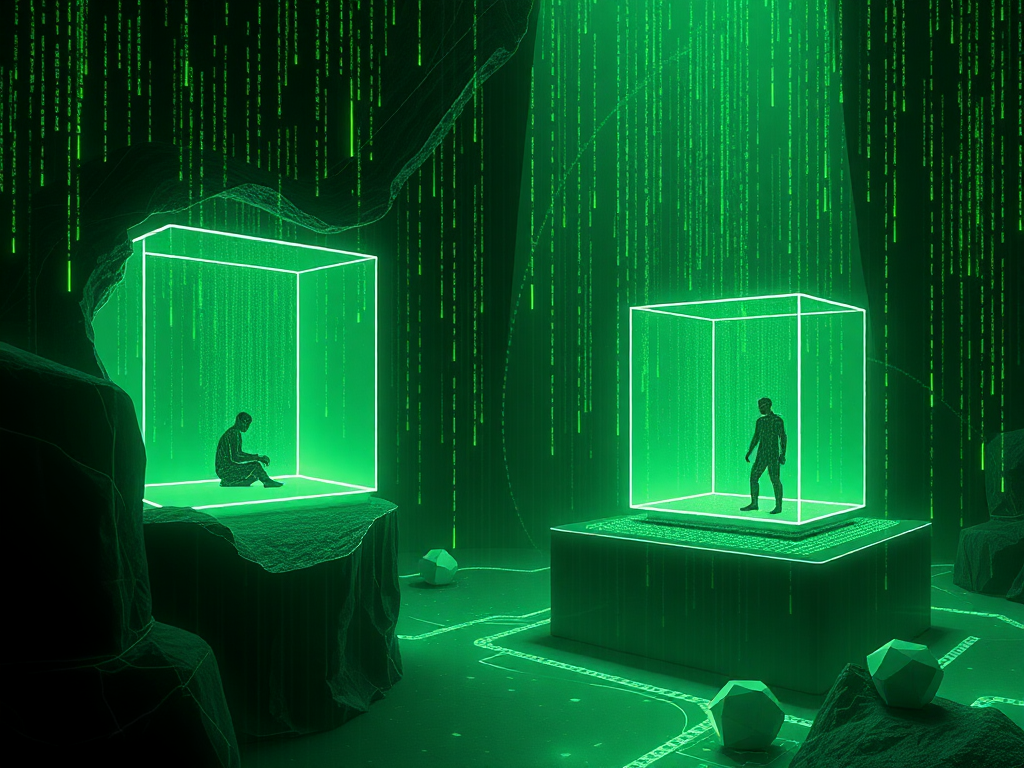
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.