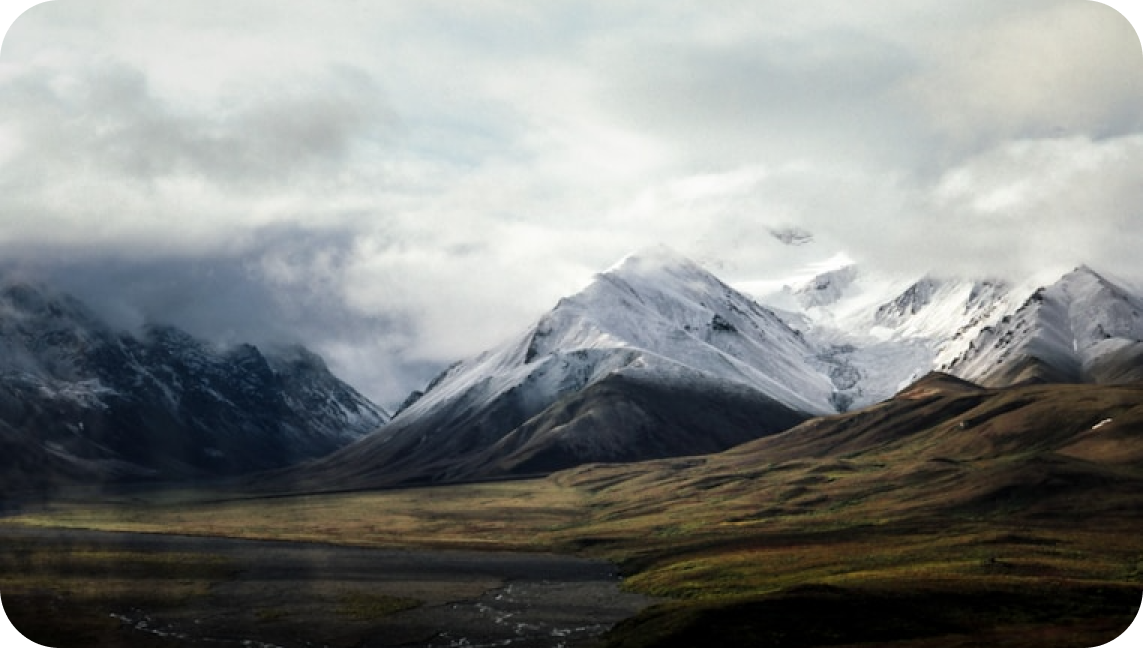
Hello, Python enthusiasts! Today we're going to talk about a very practical topic—Python virtual environments. Many of you might have faced dependency conflicts while developing Python projects, or encountered environment inconsistencies when deploying projects on different machines. Is there a way to make our project development smoother? The answer is: virtual environments!
What is a Virtual Environment?
Simply put, a virtual environment creates a standalone mini-world for your Python project. In this mini-world, you can install, use, and manage Python packages specific to that project without affecting the system's Python environment or other projects.
Imagine you're developing two projects: one requires Django 2.2, and the other needs Django 3.0. Without virtual environments, you'd have to constantly switch between these versions—how troublesome! But with virtual environments, you can create a separate environment for each project, and they won't interfere with each other. How wonderful!
Why Use Virtual Environments?
You might ask, why bother? Can't we just install everything in the system Python environment? Let me give you a few reasons to use virtual environments:
-
Isolation: Each project has its own space, without interference. You can use Django 2.2 in one project and Django 3.0 in another without worrying about version conflicts.
-
Portability: You can easily package and transfer the entire development environment. For instance, if you developed a project on your own computer and want to run it on a colleague's computer, just copy the virtual environment—it’s super convenient!
-
Cleanliness: When you no longer need a project, just delete the corresponding virtual environment without leaving useless packages in the system.
-
Version Control: You can precisely control the Python and package versions used by each project, which is crucial for ensuring long-term stability.
-
Team Collaboration: By sharing the configuration file of a virtual environment, team members can quickly set up a consistent development environment, greatly reducing the "it works on my machine" scenarios.
I remember once developing a data analysis project using pandas 1.0.3 while maintaining an old project that used pandas 0.25.3. Without virtual environments, I might have had to constantly install and uninstall different versions of pandas—a nightmare! But with virtual environments, I can easily switch between these projects, making the development experience fantastic!
How to Create a Virtual Environment?
Now that you know the benefits, are you eager to try it out? Let’s see how you can create and use virtual environments!
In Python 3.3 and later, we can use the built-in venv
module to create virtual environments. This module is simple and one of my favorite ways to create virtual environments.
First, let's create a new project directory:
mkdir my_awesome_project
cd my_awesome_project
Then, we can create a virtual environment using the following command:
python3 -m venv myenv
This command will create a folder named myenv
in the current directory, containing an independent Python interpreter and some basic tools.
After creating the virtual environment, we need to activate it. Activation methods vary by operating system:
-
On Windows:
myenv\Scripts\activate
-
On macOS and Linux:
source myenv/bin/activate
After activation, you'll see (myenv)
appear before the command line prompt, indicating that you have entered the virtual environment. Now, you can start installing the packages needed for the project!
Managing Packages in a Virtual Environment
In a virtual environment, we can use pip
to install and manage packages, just like in the system Python environment. The difference is that packages installed here will only affect the current virtual environment.
For example, you can install Django like this:
pip install django
If you need to install a specific version of a package, you can do it like this:
pip install django==3.2.4
You can even specify a version range:
pip install 'django>=3.2,<4.0'
This command will install Django version 3.2 and above, but below version 4.0.
After installing packages, you can use the pip list
command to see all packages installed in the current environment:
pip list
This command will list all installed packages and their versions.
Sharing Your Virtual Environment
When you finish project development and want to share it with others or deploy it to a server, you don’t need to copy the entire virtual environment folder. Instead, you can create a requirements.txt
file listing all the needed packages and their versions.
Creating a requirements.txt
file is simple:
pip freeze > requirements.txt
This command writes all installed packages and their versions in the current environment to the requirements.txt
file.
When others get your project code and this requirements.txt
file, they can easily recreate the same environment:
python3 -m venv new_env
source new_env/bin/activate # Use new_env\Scripts\activate on Windows
pip install -r requirements.txt
This way, on your colleague's computer or a production server, you can quickly set up an environment identical to your development environment, greatly reducing problems caused by environment inconsistencies and making team collaboration and project deployment smoother.
Exiting and Deleting a Virtual Environment
When you're done working and want to exit the virtual environment, just type:
deactivate
This command will return you to the system's Python environment.
If you no longer need a virtual environment, you can simply delete its folder. For example:
rm -rf myenv
This command deletes the virtual environment named myenv
. Note that this operation is irreversible, so make sure you really don't need the environment before deleting it.
Best Practices for Virtual Environments
I've summarized some best practices for using virtual environments that might help you:
-
Create a Separate Virtual Environment for Each Project: This avoids dependency conflicts between different projects.
-
Add the Virtual Environment Folder to .gitignore: Virtual environments usually don't need version control, so add them to .gitignore to avoid unnecessary file submissions.
-
Update requirements.txt Regularly: Update the requirements.txt file after adding or updating dependencies to ensure team members always use the latest dependency configuration.
-
Use Virtual Environment Management Tools: Tools like pyenv-virtualenv can help you manage multiple Python versions and virtual environments more easily.
-
Run pip After Activating the Virtual Environment: This ensures that the packages you install only affect the current virtual environment.
-
Use Version Control: Specify the exact package versions in requirements.txt to ensure consistency across different environments.
-
Clean Regularly: Regularly check and delete unused virtual environments to save disk space.
Advanced Tips for Virtual Environments
Once you've mastered the basics, let's explore some advanced tips to make your development work more efficient:
1. Use Environment Variables
In a virtual environment, you can set environment variables to store sensitive information or configuration details. For example, set database connection info like this:
export DATABASE_URL="postgresql://user:password@localhost/dbname"
Then, in your Python code, read this environment variable like this:
import os
database_url = os.environ.get('DATABASE_URL')
This allows you to use different configurations in different environments (development, testing, production) without modifying the code.
2. Use a Virtual Environment Wrapper
Though the venv
module is great, there are even more powerful tools like virtualenvwrapper
. It provides convenient commands to create, delete, and switch virtual environments.
Install virtualenvwrapper
:
pip install virtualenvwrapper
Then, use the following commands to manage virtual environments:
- Create a new virtual environment:
mkvirtualenv myenv
- Switch to a virtual environment:
workon myenv
- Exit the current virtual environment:
deactivate
- Delete a virtual environment:
rmvirtualenv myenv
3. Use Virtual Environments in Jupyter Notebook
If you frequently use Jupyter Notebook, you might wonder how to use virtual environments in it. It’s simple—just install ipykernel
within the virtual environment:
pip install ipykernel
python -m ipykernel install --user --name=myenv
This allows you to select this virtual environment in Jupyter Notebook.
4. Use pyenv to Manage Multiple Python Versions
If you need to use multiple Python versions on the same machine, pyenv
is a great tool. It allows you to easily switch between different Python versions.
Install pyenv (on macOS):
brew install pyenv
Then use pyenv like this:
- Install a specific Python version:
pyenv install 3.8.5
- Set the global Python version:
pyenv global 3.8.5
- Set the Python version for the current directory:
pyenv local 3.7.9
Combine with the pyenv-virtualenv
plugin to create virtual environments for different Python versions.
5. Use Docker Containers
If you want more thorough isolation, consider using Docker containers. Docker provides a completely independent runtime environment for your application, including the operating system, Python interpreter, and all dependencies.
A simple Dockerfile might look like this:
FROM python:3.8
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
With this Dockerfile, you can build a Docker image containing your app and all its dependencies. This allows your app to run in any Docker-supported environment without worrying about environment differences.
Common Issues and Solutions with Virtual Environments
You might encounter some issues while using virtual environments. Here are some common problems and their solutions:
1. Activation of Virtual Environment Fails
Issue: When trying to activate a virtual environment, you might encounter an error saying "cannot load file xxx because running scripts is disabled on this system."
Solution: This is usually due to Windows execution policy settings. You can resolve it by:
- Opening PowerShell as an administrator
- Running the command:
Set-ExecutionPolicy RemoteSigned
- Selecting 'Y' to confirm the change
2. pip Installation Fails
Issue: pip fails to install packages in a virtual environment.
Solution:
- Ensure pip is up to date: pip install --upgrade pip
- If it's a network issue, try using a mirror source: pip install package-name -i https://pypi.tuna.tsinghua.edu.cn/simple
- If it's a permission issue, try adding the --user
option: pip install package-name --user
3. Python Version in Virtual Environment Differs from System Python Version
Issue: The Python version used by your virtual environment is different from what you expected.
Solution: Specify the Python version explicitly when creating the virtual environment:
python3.8 -m venv myenv
Or use pyenv to manage Python versions.
4. Using Virtual Environment in PyCharm
Issue: Uncertain how to use a virtual environment in PyCharm.
Solution: 1. Open PyCharm settings (File > Settings) 2. Go to Project > Python Interpreter 3. Click the gear icon and select "Add" 4. Choose "Existing environment," then select the path to your virtual environment's Python interpreter
5. Virtual Environment Takes Up Too Much Disk Space
Issue: Over time, your virtual environment might take up a lot of disk space.
Solution:
- Regularly clean up unused virtual environments
- Use the pip uninstall
command to remove unnecessary packages
- Consider using pipx
to install and manage Python applications, creating a separate virtual environment for each
Conclusion
Our journey through Python virtual environments ends here. Let’s recap the main points:
- Virtual environments are powerful tools for isolating project dependencies and enhancing development efficiency.
- The
venv
module makes it easy to create and manage virtual environments. - In a virtual environment, you can freely install and manage packages without affecting the system environment.
- With a
requirements.txt
file, you can easily share and replicate project environments. - There are many advanced tips and tools to make virtual environments more efficient, such as using environment variables and virtual environment wrappers.
- While using virtual environments, you may encounter issues, but most have corresponding solutions.
Virtual environments may seem like a small tool, but they play a crucial role in Python development. Mastering virtual environments can make your development work smoother and project management more efficient.
Have you encountered interesting issues or discovered useful tips while using virtual environments? Feel free to share your experiences in the comments!
Remember, in the world of Python, virtual environments are like your personal mini-universe. Here, you can experiment and create freely without worrying about affecting other projects or system environments. So, explore boldly, and you will discover more joys in Python development!
Lastly, I hope this article helps you. If you have any questions, feel free to ask. The world of Python is vast and boundless; let’s continue exploring it together!
Next
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.
Next
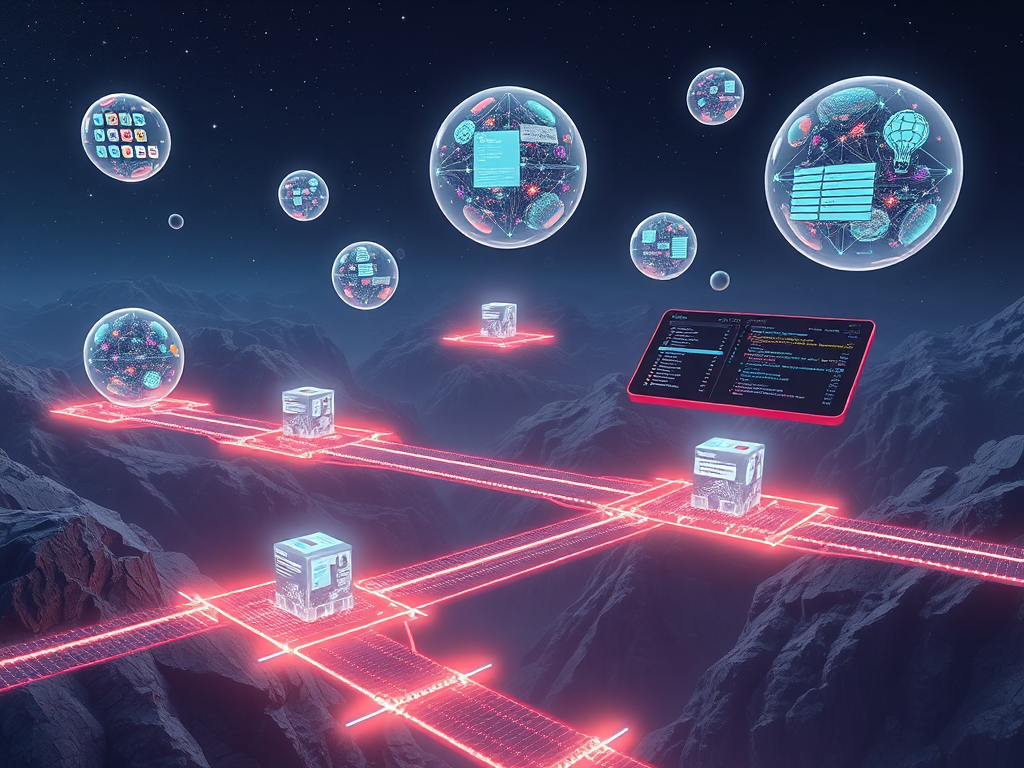
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
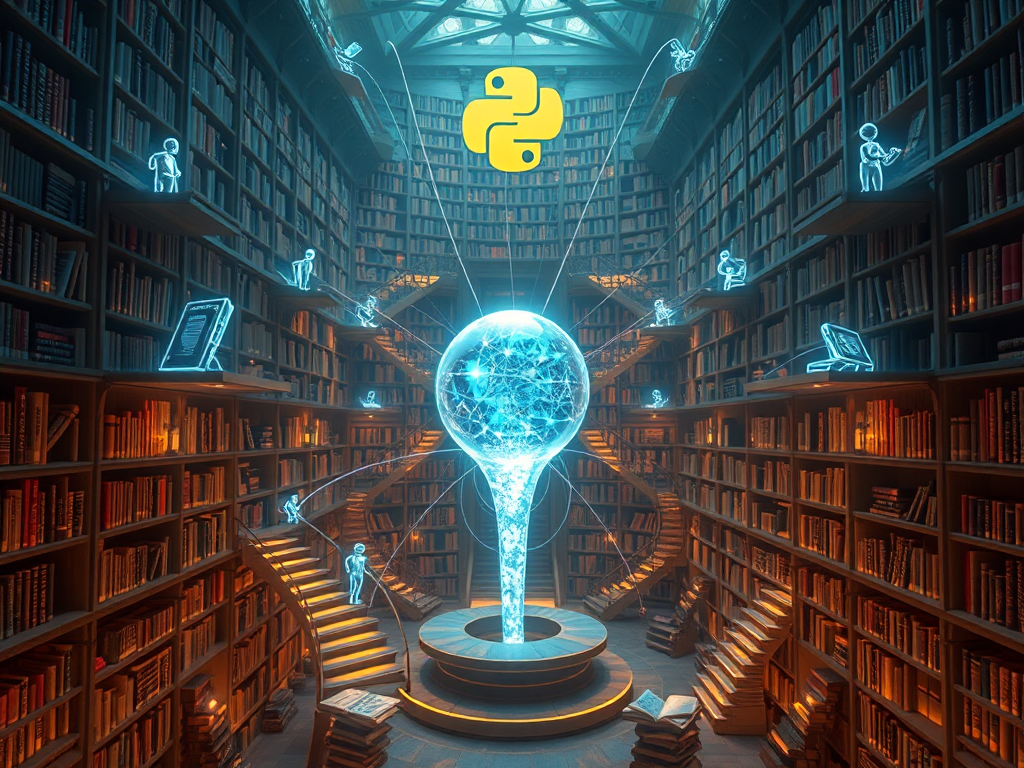
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
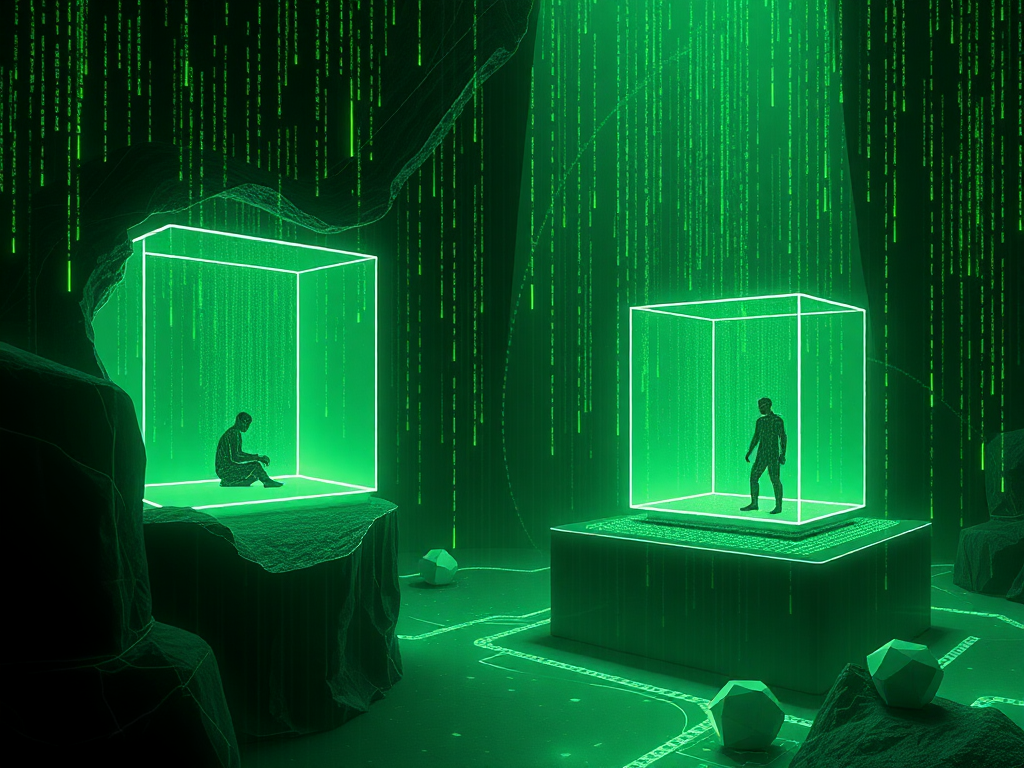
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.