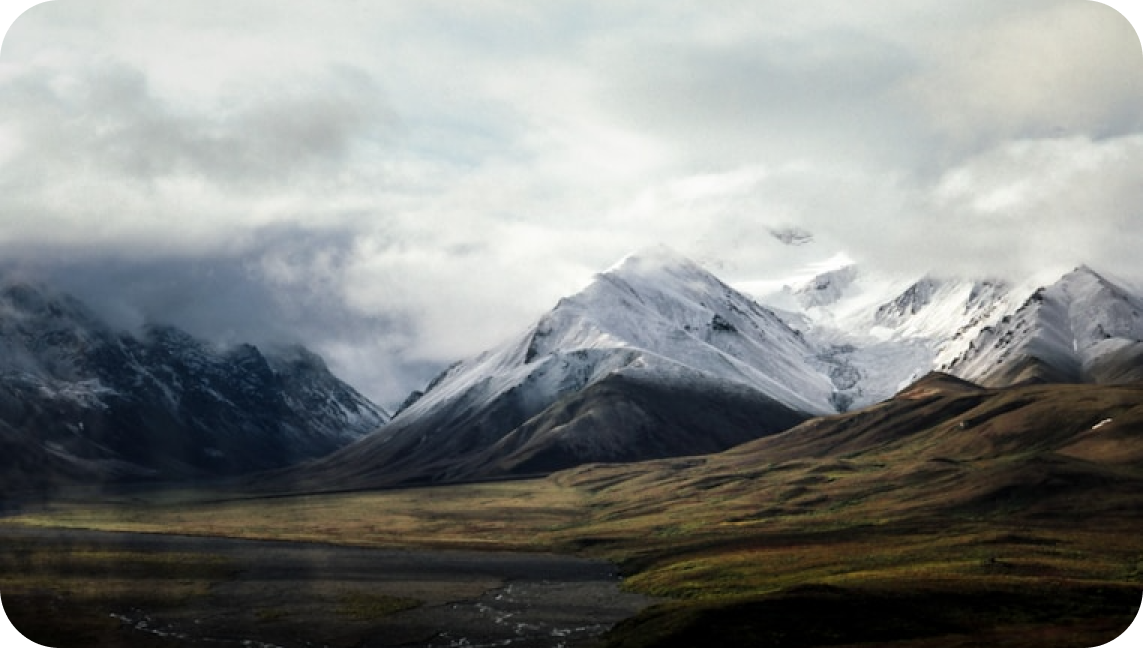
Hello, dear Python enthusiasts! Today, we will discuss a very important but often overlooked topic in Python development - virtual environments. Many beginners have likely encountered this frustration: the code is exactly the same, but why does it run well on others' computers and encounter all kinds of errors on your own? Or one project uses Django 2.x version, while another project requires Django 3.x, how should this be managed? Don't worry, these problems can be easily solved with the help of virtual environments. So, what is a virtual environment? Why do we need it? How do we use it correctly? Let's explore the answers to these questions together!
Introduction to Virtual Environments
What is a Virtual Environment?
Imagine that you have a magical toolbox, and every time you start a new project, you can create a brand new, independent Python environment from this toolbox. This environment has its own Python interpreter, its own libraries and tools, completely unaffected by other projects or system environments. Doesn't that sound great? This is the magic of virtual environments!
A virtual environment is like creating an "isolated space" for your Python project. In this space, you can install, upgrade, and remove various packages without worrying about affecting other projects or the system environment. Each virtual environment is independent and does not interfere with each other. This means you can use Django 2.2 in one project and Django 3.1 in another project, and they can coexist harmoniously without any conflicts.
Why Do We Need Virtual Environments?
You may ask, "Can't I just install all the packages I need directly in the system environment? Why bother with this extra step?" That's a good question! Let me give you a vivid example.
Imagine you are a chef, and your kitchen is your Python environment. If you only have one kitchen (system environment) and need to prepare Chinese, Western, and dessert dishes at the same time, what would that scenario be like? Seasonings and tools would be everywhere, interfering with each other, and you might accidentally put sugar into a savory dish. This is the predicament you may encounter if you don't use virtual environments.
By using virtual environments, it's like having a separate kitchen for each cuisine. Chinese dishes have a Chinese kitchen, Western dishes have a Western kitchen, and desserts have a dessert kitchen. Each kitchen has its own dedicated tools and seasonings, without interference. This way, you can easily handle various "cuisines" (projects) without worrying about "seasoning" (dependency package) mixing issues.
Specifically, using virtual environments has the following important benefits:
-
Avoid Dependency Conflicts: Different projects may depend on different versions of the same library. For example, Project A needs Django 2.2, while Project B needs Django 3.1. If both are installed in the system environment, a conflict will occur. Using virtual environments, each project has its own Django version, solving the problem effortlessly.
-
Improve Portability: By packaging the project and all its dependencies into a virtual environment, you can easily migrate the entire project to other machines. You don't have to worry about missing dependencies on the new machine because everything needed is in the virtual environment.
-
Enhance Reproducibility: Reproducibility is very important in software development. Using virtual environments, you can ensure that the project runs in exactly the same way on different machines. This is especially important for team collaboration and continuous integration.
-
Keep the System Environment Clean: If all projects directly use the system environment, the system environment will become cluttered over time. Using virtual environments, you can keep the system environment clean, with each project's dependencies isolated in its own virtual environment.
-
Facilitate Experimentation: Want to try a new library but are unsure if it will be used? Install and test it in a virtual environment, and if you're not satisfied, you can simply delete the entire environment without affecting the system environment or other projects.
After seeing this, do you have a deeper understanding of the importance of virtual environments? Next, let's learn how to create and use virtual environments together!
Creating Virtual Environments
Okay, now that we know why we need virtual environments, let's create one! In Python, there are multiple ways to create virtual environments. We will mainly introduce two of the most common methods: venv
and virtualenv
.
Using venv to Create Virtual Environments
venv
is the built-in virtual environment creation tool in Python 3.3 and later versions. If you are using Python 3.3+, I strongly recommend using venv
because it is simple, convenient, and does not require installing additional packages.
The command to create a virtual environment is:
python3 -m venv <virtual_environment_name>
For example, if we want to create a virtual environment named .venv
, we can do:
python3 -m venv .venv
This command will create a folder named .venv
in the current directory, containing the Python interpreter, standard library, and various support files.
You may wonder, "Why use .venv
as the name?" This is a good question! Using .venv
as the name of the virtual environment is a common convention. Folders starting with a dot (.) are hidden in Unix systems, which avoids the virtual environment folder interfering with your project files. Of course, you can also use other names, such as env
, venv
, or project-related names, depending entirely on your preference.
Using virtualenv to Create Virtual Environments
virtualenv
is another popular virtual environment creation tool. One of its advantages is that it can be used in both Python 2 and Python 3, so if you are still using Python 2 (although I strongly recommend upgrading to Python 3), or need to switch between different Python versions, virtualenv
would be a good choice.
First, you need to install virtualenv
:
pip install virtualenv
Then, the command to create a virtual environment is:
virtualenv <virtual_environment_name>
For example:
virtualenv .venv
This command has the same effect as using venv
, creating a new folder containing the Python environment.
Specifying Python Version
Regardless of whether you use venv
or virtualenv
, you can specify the Python version when creating the virtual environment. This is particularly useful when you have multiple Python versions installed on your system.
Using venv
:
python3.8 -m venv .venv # Use Python 3.8 to create the virtual environment
Using virtualenv
:
virtualenv -p python3.8 .venv # Use Python 3.8 to create the virtual environment
This way, you can create virtual environments using different Python versions for different projects. Isn't that convenient?
Activating the Virtual Environment
After creating the virtual environment, the next step is to activate it. After activating the virtual environment, your command line prompt will usually change to show the name of the currently activated virtual environment. At this point, any packages you install will be installed into this virtual environment, not the system environment.
The command to activate the virtual environment is slightly different on different operating systems:
-
On Linux or macOS:
bash source .venv/bin/activate
-
On Windows:
bash .venv\Scripts\activate
After activation, you will see the command prompt change to something like this:
(.venv) $
This indicates that you have successfully entered the virtual environment. Now you can start installing packages and running Python scripts in this independent environment!
Deactivating the Virtual Environment
When you have finished your work, you can use the following command to exit the virtual environment:
deactivate
This command is universal across all operating systems. After executing it, you will return to the system's global Python environment.
Okay, now you have learned how to create, activate, and deactivate virtual environments. Doesn't it feel simple? Don't rush, next we will learn some more advanced usages to make you a true virtual environment master!
Advanced Virtual Environment Usage
Managing Dependencies
In the process of using virtual environments, managing project dependencies is a very important topic. Imagine if you need to share your project with others, or set up the environment on another machine, how do you ensure that all necessary packages are correctly installed? This is the problem that dependency management aims to solve.
Generating a Dependency List
In an activated virtual environment, you can use the following command to generate a file containing all installed packages and their versions:
pip freeze > requirements.txt
This command will create (or overwrite) a file named requirements.txt
, listing all packages installed in the current environment and their exact versions. For example:
Django==3.1.7
requests==2.25.1
numpy==1.20.1
Installing from a Dependency List
With the requirements.txt
file, it becomes very simple to reinstall all dependencies in a new environment:
pip install -r requirements.txt
This command will read the requirements.txt
file and install all packages listed in it.
This method is very suitable for team collaboration and project deployment. You can commit the requirements.txt
file to a version control system (like Git), so other developers can easily reproduce your development environment.
Using Different Package Management Tools
Although pip
is Python's default package management tool, there are some other tools that can provide more advanced features. Here, I want to introduce two commonly used alternative tools: Pipenv and Poetry.
Pipenv
Pipenv is a more modern dependency management tool that combines the functionality of pip
and virtualenv
, and provides a simpler workflow.
Install Pipenv:
pip install pipenv
Use Pipenv to create a virtual environment and install packages:
pipenv install requests
This command will create a new virtual environment (if one doesn't exist yet) and install the requests package in it.
Pipenv uses Pipfile
and Pipfile.lock
to track dependencies instead of requirements.txt
. Pipfile
contains high-level dependency information, while Pipfile.lock
contains exact version information, ensuring environment reproducibility.
Poetry
Poetry is another popular dependency management tool that provides a range of features including dependency resolution, building, and publishing.
Install Poetry:
pip install poetry
Use Poetry to create a new project:
poetry new my-project
Add dependencies:
poetry add requests
Poetry uses the pyproject.toml
file to manage project configuration and dependencies.
These tools all have their advantages, and which one to choose mainly depends on your project requirements and personal preferences. Personally, I prefer using Poetry because it provides more comprehensive project management features, but for simple projects, the combination of venv
+ pip
is completely sufficient.
Using Virtual Environments in IDEs
Many Python developers prefer to use an Integrated Development Environment (IDE) for development. Most modern IDEs have good support for virtual environments. Here, I'll use PyCharm as an example to introduce how to use virtual environments in an IDE.
- When creating a new project, PyCharm will prompt you to create a new virtual environment or use an existing one.
- For existing projects, you can select or create a virtual environment in "File" > "Settings" > "Project: YourProjectName" > "Python Interpreter".
- After selecting the virtual environment, PyCharm will automatically use this environment to run your code and install packages.
The benefit of using an IDE to manage virtual environments is that you can directly view and manage installed packages in the IDE, and when running code, it will automatically use the correct environment.
Virtual Environment Best Practices
In the process of using virtual environments, I have summarized some best practices that I hope will be helpful to you:
-
Create an Independent Virtual Environment for Each Project: This is the core principle of using virtual environments. Even for seemingly related projects, it's best to use separate environments.
-
Use Meaningful Virtual Environment Names: Although
.venv
is a common name, for cases with multiple related projects, using more descriptive names likemyproject-env
may be better. -
Exclude Virtual Environments from Version Control: Add the virtual environment folder (e.g.,
.venv
) to the.gitignore
file. Virtual environments are generated locally and do not need to be committed to the repository. -
Keep
requirements.txt
(or Equivalent File) Updated: Update the dependency file whenever you add or update dependencies. This ensures that others (including your future self) can accurately reproduce your environment. -
Update Dependencies Regularly: Regularly check and update your dependencies to get bug fixes and new features. However, be aware that updates may introduce incompatible changes, so thorough testing is required after updating.
-
Consider Using Dependency Locking: Tools like Pipenv and Poetry provide dependency locking features, which ensure that exactly the same package versions are installed across different environments.
-
Be Mindful of Python Versions: Make sure to use the correct Python version when creating virtual environments. Different Python versions may lead to different behaviors.
-
Use Virtual Environment Wrappers: Consider using tools like
virtualenvwrapper
, which can make virtual environment management more convenient.
Following these best practices will make your development process smoother and help you better organize and manage your projects.
Common Virtual Environment Issues and Solutions
In the process of using virtual environments, you may encounter some common problems. Don't worry, here I have summarized some common issues and their solutions, which hopefully will help you avoid some detours.
1. Failed to Activate Virtual Environment
Problem: An error occurs when trying to activate the virtual environment.
Solution:
- Make sure you are in the correct directory. You should be in the parent directory of the virtual environment folder.
- Check if the virtual environment folder exists.
- On Windows, you may need to change the PowerShell execution policy. Try running:
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned -Scope CurrentUser
2. Packages Installed in the Wrong Environment
Problem: When installing packages, they are installed in the global environment instead of the virtual environment.
Solution:
- Make sure you have activated the virtual environment. The command prompt should show the name of the virtual environment.
- Use which python
(on Unix systems) or where python
(on Windows) to check the path of the currently used Python interpreter.
3. Missing System Packages in Virtual Environment
Problem: Unable to use certain system-level packages in the virtual environment.
Solution:
- When creating the virtual environment, use the --system-site-packages
option to allow the virtual environment to access system packages. For example:
python3 -m venv --system-site-packages .venv
- Or, reinstall the required packages in the virtual environment.
4. IDE Unable to Recognize Virtual Environment
Problem: The IDE (such as PyCharm or VSCode) cannot correctly recognize or use the virtual environment.
Solution: - Manually select the Python interpreter of the virtual environment in the IDE settings. - Make sure the virtual environment folder is inside the project directory, as many IDEs can automatically detect virtual environments within the project.
5. Dependency Conflicts
Problem: Dependency conflicts occur when installing a new package.
Solution:
- Try upgrading the conflicting package with pip install <package_name> --upgrade
.
- If the problem persists, you may need to create a new virtual environment and carefully select compatible package versions.
- Consider using tools like pip-tools
or Poetry to manage dependencies, as they can better handle dependency conflicts.
6. Virtual Environments Take Up Too Much Disk Space
Problem: Multiple virtual environments take up a lot of disk space.
Solution:
- Regularly clean up unused virtual environments.
- Use tools like pyenv
to manage Python versions, which allows multiple virtual environments to share the same base files.
7. Using Virtual Environments in Jupyter Notebook
Problem: Unsure how to use a specific virtual environment in Jupyter Notebook.
Solution:
- In the activated virtual environment, install ipykernel:
pip install ipykernel
- Then, create a new kernel:
python -m ipykernel install --user --name=myenv
- In Jupyter Notebook, you can then select this newly created kernel.
Remember, don't get discouraged if you encounter problems! The process of solving these problems is also an opportunity to learn and improve. By solving these common issues, you will gain a deeper understanding of Python environments and package management.
Conclusion
Our journey through Python virtual environments has come to an end. Let's review the important knowledge points we have learned:
-
Virtual environments are a powerful tool that can help us create independent Python environments for each project, avoid dependency conflicts, and improve project portability and reproducibility.
-
Virtual environments can be created using Python's built-in
venv
module or third-party tools likevirtualenv
. -
Managing dependencies is a key part of using virtual environments. We learned how to generate and use
requirements.txt
files, as well as some more advanced dependency management tools like Pipenv and Poetry. -
In practice, we need to pay attention to some best practices, such as creating an independent virtual environment for each project, keeping dependency files up-to-date, and more.
-
In the process of using virtual environments, we may encounter some common problems, but as long as we understand how virtual environments work, these problems can be solved.
Virtual environments may seem like a small tool, but they play a crucial role in Python development. By mastering virtual environments, you have mastered an important skill in managing Python projects. It not only makes your development process smoother but also helps you better organize and manage your projects.
Remember, using virtual environments is a good habit that can make your Python journey more enjoyable. Whether you are developing a small script or building a large application, virtual environments are your powerful assistants.
Finally, I want to say, don't be afraid to try and make mistakes. Every Python developer has encountered problems in the process of using virtual environments, which is an inevitable part of the learning process. What's important is that we learn from these experiences and continuously improve our skills.
I hope this article has helped you better understand and use Python virtual environments. If you have any questions or thoughts, feel free to leave a comment for discussion. Wishing you a smooth sailing Python journey!
Do you have any other questions about virtual environments? Or do you have any unique experiences to share in the process of using virtual environments? Let's discuss together in the comments and make progress together!
Next
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.
Next
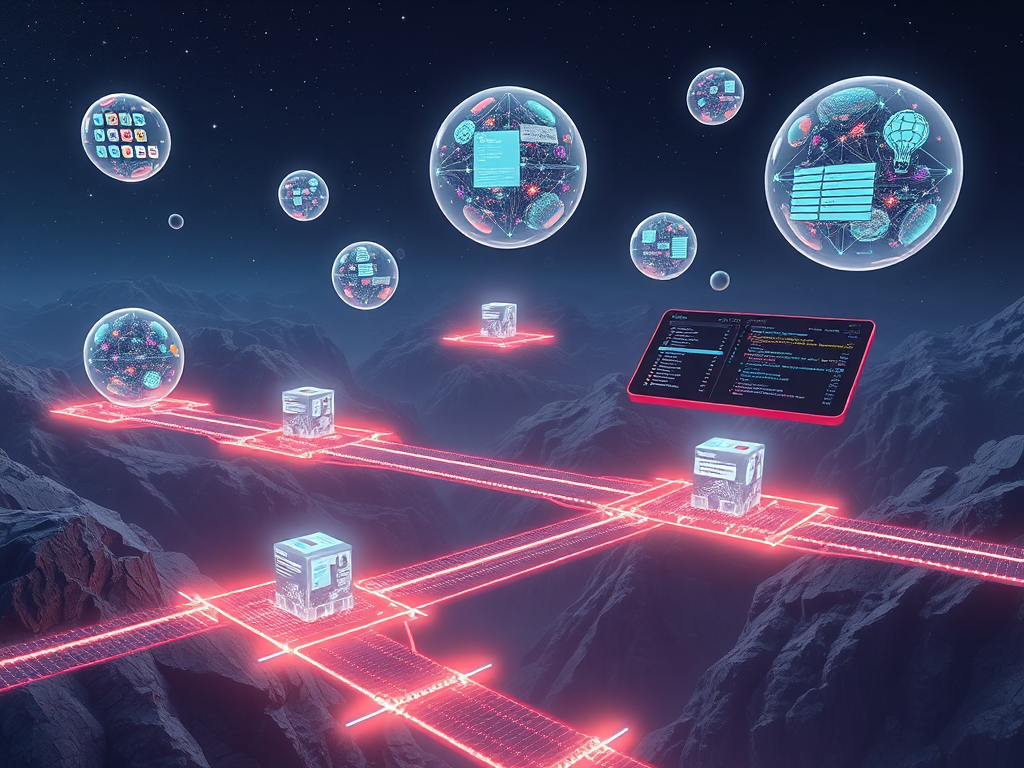
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
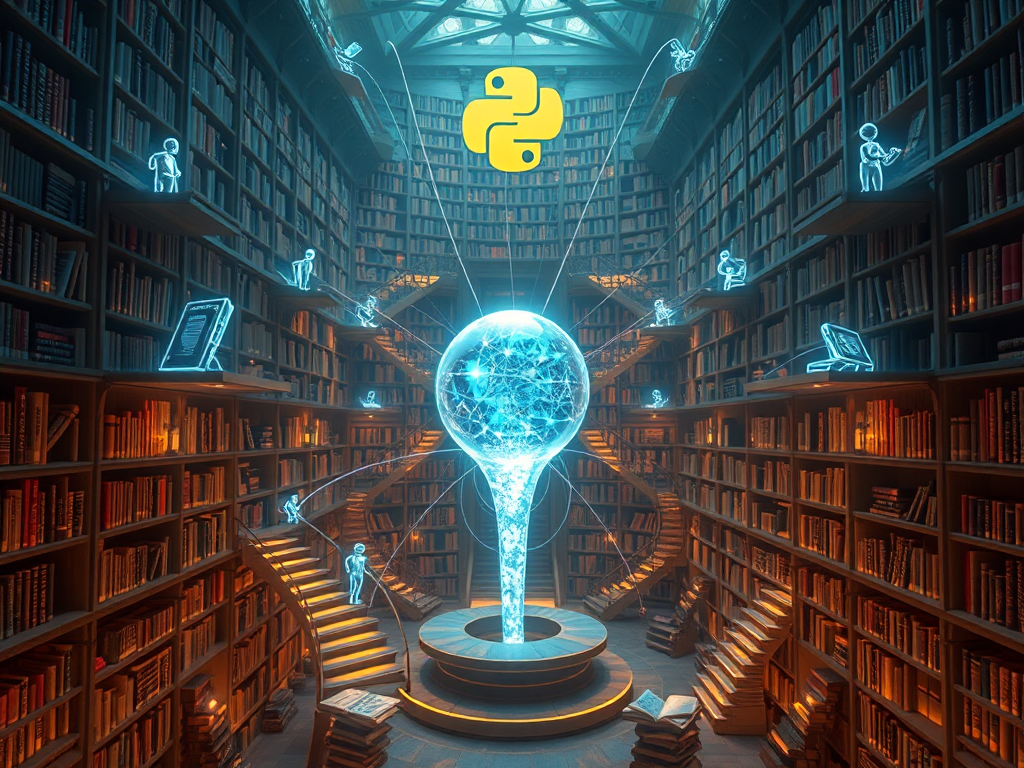
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
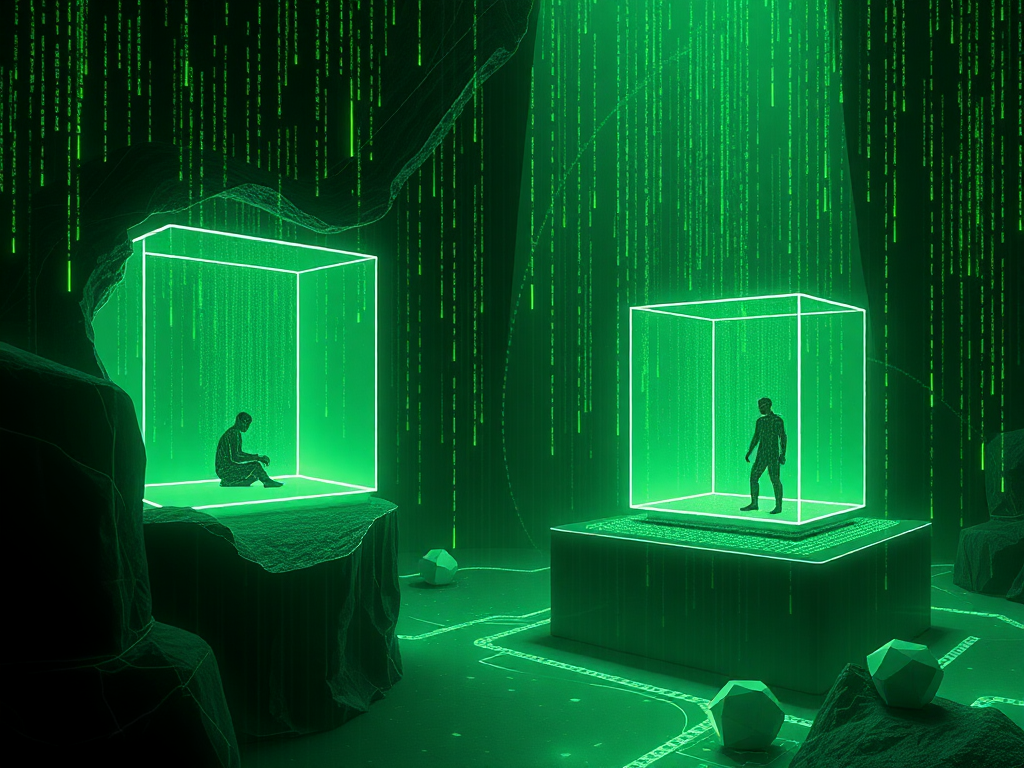
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.