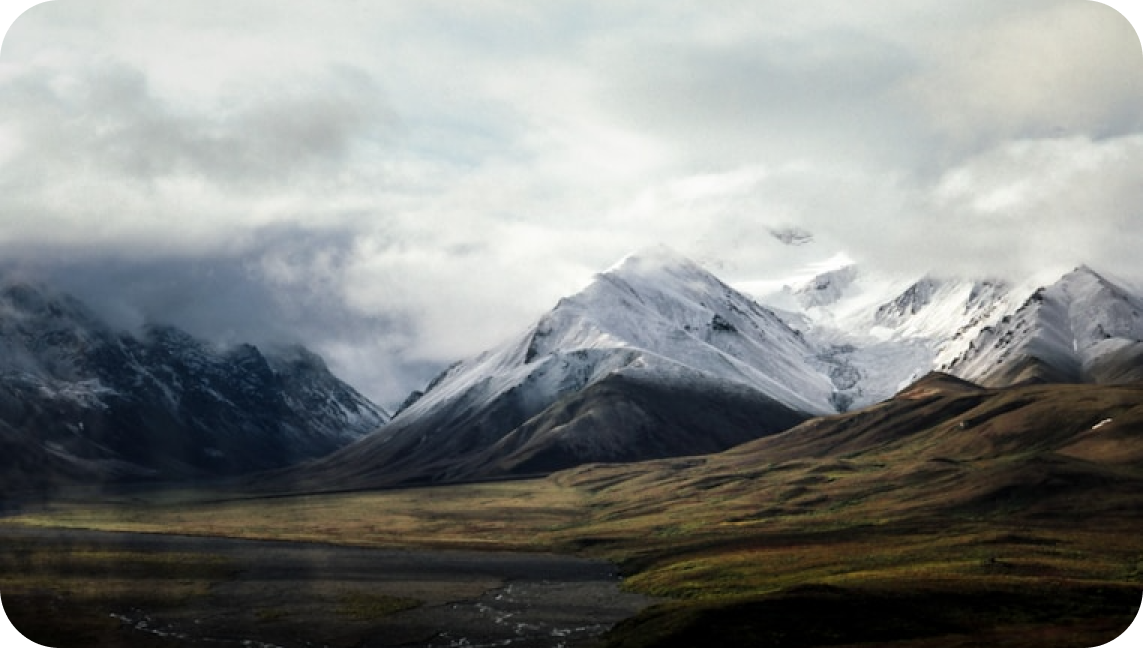
Hello everyone! Today let's talk about an important topic in Python programming - virtual environments. Have you been tortured by various package dependency issues? Or do you always mix up environments when switching between different projects? Don't worry, virtual environments were born to solve these troubles! Let's dive deep into all aspects of this powerful tool together.
Introduction
First, what is a virtual environment? Simply put, it's an isolated Python runtime environment. Imagine you have a magic box containing a specific version of the Python interpreter and all necessary packages. Every time you develop a new project, you can create a brand new magic box that only contains what this project needs. Isn't that cool?
So, why do we need this "magic box"? There are several main reasons:
- Project isolation: Different projects may require different versions of packages, and virtual environments allow them to coexist harmoniously.
- Dependency management: Easily manage dependencies for each project without worrying about polluting the global environment.
- Version control: Precisely control the versions of Python interpreter and various packages.
- Team collaboration: Ensure team members use the same development environment, reducing embarrassing situations of "it works on my computer".
Sounds useful, right? Now, let's see how to create and use this magical tool.
Creation
Creating a virtual environment is actually very simple. In Python 3.3 and above, we can use the built-in venv
module. Open the terminal and enter the following command:
python -m venv myenv
Here, myenv
is the name you want to give to the virtual environment, which can be changed freely. After execution, the system will create a folder named myenv
in the current directory, containing an independent Python environment.
Activation
After creating the virtual environment, we need to activate it. Once activated, our terminal will switch to this isolated environment. The activation method varies depending on the operating system:
-
Windows:
myenv\Scripts\activate
-
macOS/Linux:
source myenv/bin/activate
After successful activation, you'll see (myenv)
appear in front of the command prompt, indicating that you've entered the virtual environment.
Usage
After activating the virtual environment, you can start installing the packages needed for your project. For example:
pip install numpy pandas matplotlib
These packages will only be installed in the virtual environment and won't affect your global Python environment.
When you're done working, you can use the following command to exit the virtual environment:
deactivate
Isn't it simple? But wait, you might encounter some problems in actual use. Let's look at how to solve these common troubles.
Common Issues
Environment Selection in VSCode
Sometimes, even if you've selected the correct virtual environment in VSCode, scripts still use the base environment. What's going on?
Solution: 1. Open the command palette (Ctrl+Shift+P) 2. Type "Python: Select Interpreter" 3. Choose the correct virtual environment
If the problem persists, try restarting VSCode and make sure the virtual environment is activated in the terminal.
In my personal experience, sometimes VSCode's cache can cause this issue. Clearing the cache or reopening the project usually solves it.
Package Import Issues
After creating a virtual environment, sometimes you might find that you can't import installed modules. This could be because the package wasn't correctly installed in the virtual environment.
Solution:
1. Make sure the virtual environment is activated
2. Run pip list
to check installed packages
3. If a package is missing, use pip install <package_name>
to install it
I've encountered this problem before. Once I clearly remembered installing numpy, but the code reported an error saying it couldn't find the module. Later I realized I forgot to activate the virtual environment and installed the package in the global environment. The problem was solved after activating the correct environment.
Module Import Errors
Sometimes, even if the package is installed, you might still encounter import errors. For example, encountering a "No module named 'sqlalchemy'" error when using FastAPI.
Solution:
1. Make sure SQLAlchemy is installed in the virtual environment: pip install sqlalchemy
2. Check if the correct virtual environment is selected as the interpreter
I've encountered this problem many times. Sometimes it's because I forgot to install packages in the new virtual environment, sometimes it's because I accidentally used the global environment. Developing the habit of creating a new virtual environment for each new project can greatly reduce such problems.
Multiple Environment Conflicts
Sometimes, you might find that Python is sourcing from multiple environments, causing various strange issues.
Solution:
1. Use separate virtual environments for each project
2. Make sure to activate the correct environment before running any commands
3. Use the which python
and which pip
commands to check the current Python and pip paths
I once spent an entire day debugging because of environment confusion and couldn't find the problem. Later, upon careful inspection, I realized that different packages were coming from different environments. Since then, I've always been particularly careful about environment isolation and activation.
Best Practices
After talking about so many problems, how can we avoid these pitfalls? Here are some best practices I've summarized, hope they're helpful:
-
Project isolation: Create separate virtual environments for each project. This avoids dependency conflicts between projects.
-
Use requirements.txt: Record project dependencies in a requirements.txt file. You can generate this file using the
pip freeze > requirements.txt
command. -
Version control: Add the virtual environment folder (like venv/) to the .gitignore file, but include requirements.txt.
-
Environment consistency: When setting up a project on a new machine, first create a virtual environment, then use
pip install -r requirements.txt
to install dependencies. -
Regular updates: Regularly update packages in the virtual environment, but be sure to test compatibility.
-
Use virtual environment wrappers: Consider using tools like pyenv-virtualenv, which can more conveniently manage multiple Python versions and virtual environments.
-
Documentation: Explain how to set up and activate the virtual environment in the project README, this is very helpful for new team members.
These practices might seem a bit troublesome, but trust me, they will make your development process much smoother. It was only after experiencing countless times of "why does it run on his computer but not mine" that I deeply understood the importance of these practices.
Summary
Virtual environments are a powerful tool in Python development that can help us better manage project dependencies and avoid environment conflicts. Although you may encounter some problems during use, as long as you master the correct usage methods and solutions to some common problems, you can fully leverage its power.
Remember, one virtual environment per project, install packages after activating the environment, use requirements.txt to manage dependencies. These simple habits will take you further on your Python development journey.
So, do you have any insights on using virtual environments? Feel free to share your experiences and tips in the comments section. Let's learn and progress together!
On the programming journey, we are all lifelong learners. I hope this article has been helpful to you. See you next time!
Next
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.
Next
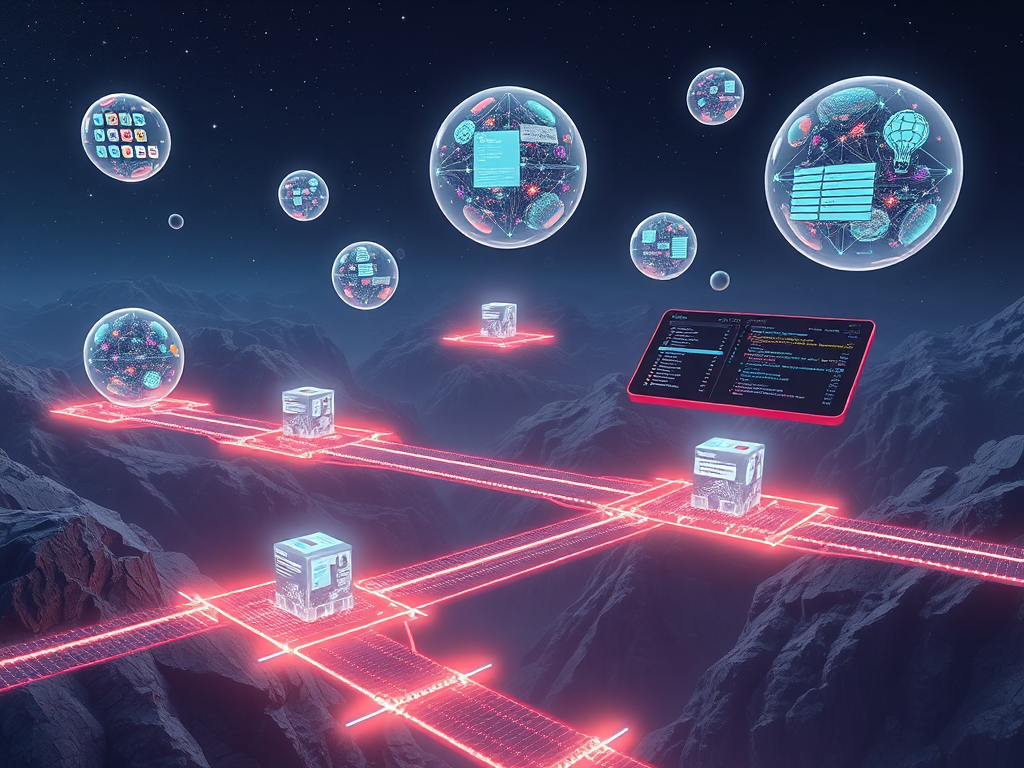
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
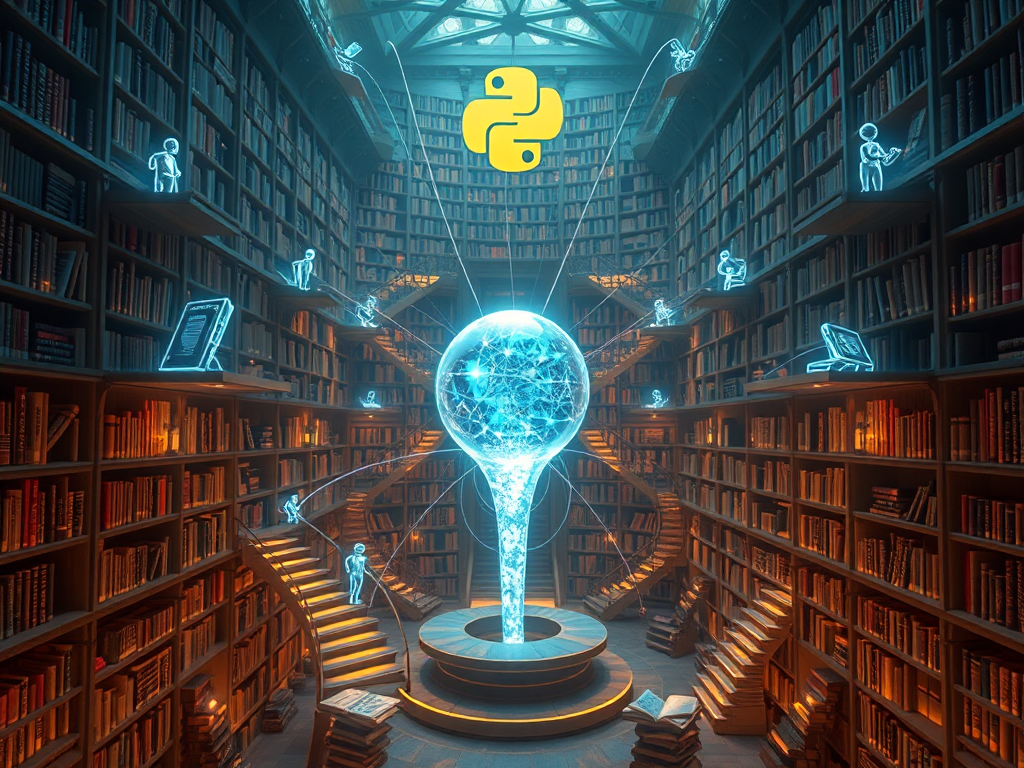
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
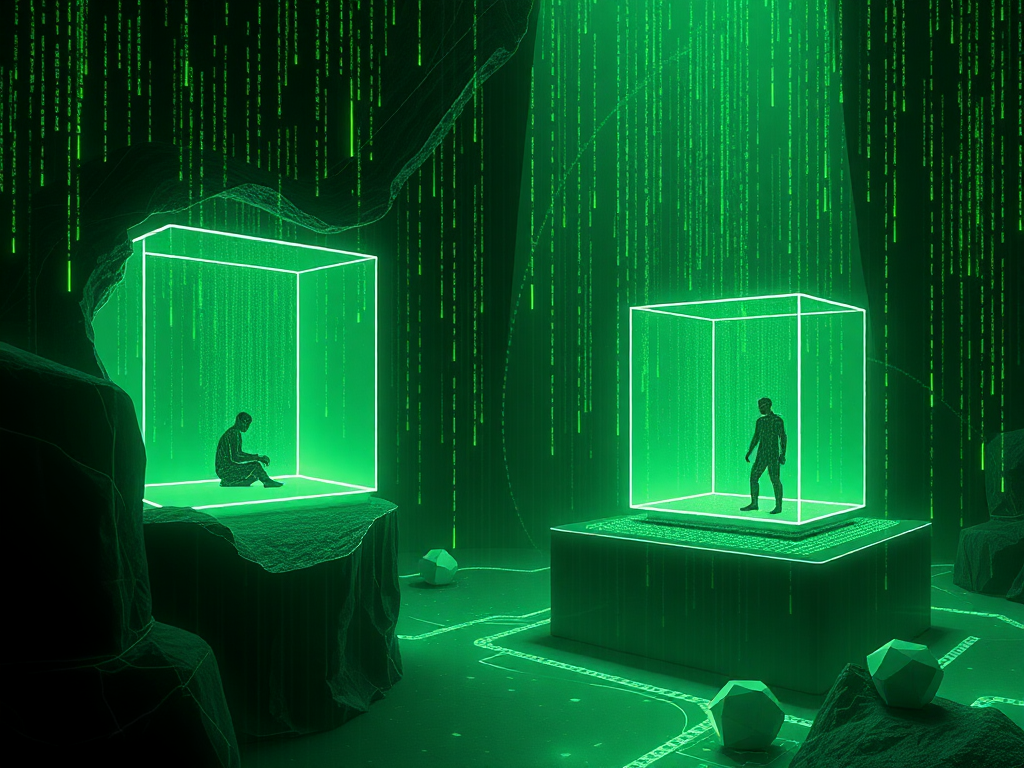
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.