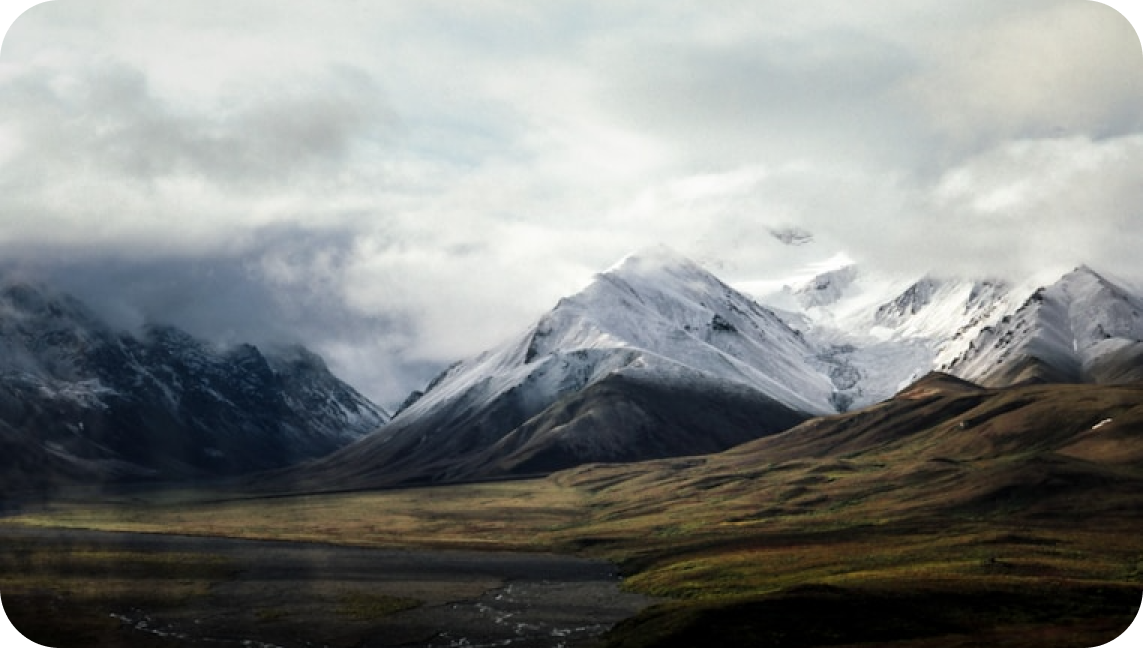
Hey, Python enthusiasts! Today, let's talk about Python virtual environments. Are you often troubled by dependency conflicts between different projects? Or confused by various package version issues? Don't worry, virtual environments were born to solve these problems. Let's explore the mysteries of this powerful tool together!
Unveiling the Mystery
First, we need to understand what a virtual environment actually is. Simply put, a virtual environment is like creating an independent small world for your Python project. In this world, you can install, use, and manage specific versions of Python packages needed for that project without affecting other projects or system-level Python environments.
Imagine you have two projects: one needs Django 2.2, while the other requires Django 3.0. Without virtual environments, you'd have to switch versions back and forth in the global environment, which is a nightmare! But with virtual environments, each project can have its own Django version, without interfering with each other. How wonderful is that!
Why Use Virtual Environments?
You might ask, "This sounds good, but do I really need to use virtual environments?" Let me tell you, there are so many benefits to using virtual environments:
-
Dependency Isolation: Each project has its own set of dependencies, without affecting each other. No more worrying about updates to Project A breaking Project B!
-
Version Control: You can use different versions of Python and packages in different virtual environments. Want to try the latest Python 3.9 features? Just create a new virtual environment, without affecting your other projects.
-
Clearer Project Structure: Virtual environments make your project structure clearer. All dependencies are in one place, easy to manage and share.
-
Easier Environment Reproduction: By recording the dependencies in the virtual environment, you can easily reproduce the same development environment on other machines. This is a blessing for team collaboration!
-
Avoid Permission Issues: Installing packages in a virtual environment usually doesn't require administrator privileges, making the development process safer and more convenient.
At this point, are you eager to start using virtual environments? Don't rush, next we'll look at how to apply this powerful tool in practical work.
Virtual Environment Magic in VS Code
As a Python developer, I guess you probably use Visual Studio Code (VS Code) as your main IDE. The good news is, VS Code has excellent support for Python virtual environments! Let's see how to harness the power of virtual environments in VS Code.
Activating a Virtual Environment
First, we need to learn how to activate a virtual environment. In VS Code, this process is actually very simple:
- Open your project folder.
- Press
Ctrl+`` (or
Cmd+`` if you're on a Mac) to open the integrated terminal. - Enter the following command (depending on your operating system):
- Windows:
.venv\Scripts\activate
- Linux/Mac:
source .venv/bin/activate
Done! You should see (.venv)
or a similar marker appear before the terminal prompt, indicating that the virtual environment has been activated.
But wait, if you find that the virtual environment isn't working as expected, don't panic! Let's look at possible reasons and solutions.
Solving Virtual Environment Ineffectiveness Issues
Sometimes, even if you've selected the correct virtual environment, VS Code might still report errors about not finding certain modules. What's going on? Usually, there are several possible reasons:
-
Path Recognition Issue: VS Code might not have correctly recognized the path to the virtual environment. The solution is to manually set the Python interpreter path.
-
Settings Not Updated: Sometimes VS Code settings might not update in time. Try restarting VS Code or manually refreshing the window.
-
Virtual Environment Not Correctly Created: Make sure your virtual environment is correctly created and contains all necessary files and directories.
If you encounter these problems, don't worry, let's look at specific solution steps:
- Open the VS Code command palette (Windows/Linux:
Ctrl+Shift+P
, Mac:Cmd+Shift+P
). - Type "Python: Select Interpreter" and select it.
- In the pop-up list, select your virtual environment path. If you don't see it, you can click "Enter interpreter path" to manually input it.
- The path should be similar to
.venv/bin/python
(Linux/Mac) or.venv\Scripts\python.exe
(Windows).
After setting this up, reopen your Python file, and VS Code should correctly recognize the virtual environment.
Package Management: "Supermarket Shopping" in Virtual Environments
Now that we've successfully activated the virtual environment, here comes the most exciting part - installing and managing packages! Imagine your virtual environment as an exclusive supermarket where you can freely choose various Python packages without worrying about affecting other projects. Isn't that cool?
Installing Packages with pip
Installing packages in a virtual environment is very simple, just like in a regular Python environment. Make sure your virtual environment is activated, then use the pip command:
pip install package_name
For example, if you want to install Django, just do:
pip install django
It's that simple! pip will automatically install Django and its dependencies in your virtual environment.
Viewing Installed Packages
Want to know what "products" are in your "supermarket"? Use the following command to list all installed packages:
pip list
This command will display all packages installed in the current virtual environment and their versions. Very convenient, right?
However, sometimes you might encounter some permission issues, especially when installing certain special packages. Don't worry, let's look at how to solve these annoying permission problems.
Solving Installation Permission Issues
When using virtual environments, you might sometimes encounter insufficient permissions issues, especially when trying to install certain packages. In this case, there are several methods you can try:
Using the --user Option
If you encounter permission issues, you can try using the --user
option:
pip install --user package_name
This will install the package in your user directory instead of the system directory, which can usually avoid permission issues.
Using Administrator Privileges
If the --user
option doesn't work, you might need to use administrator privileges:
- On Windows, run the command prompt as an administrator.
- On Linux/Mac, use
sudo
:
sudo pip install package_name
But note that using sudo
to install packages might affect the system Python environment, so use it cautiously.
My personal advice is to avoid using administrator privileges to install packages as much as possible. One of the main advantages of virtual environments is being able to manage packages without administrator privileges, so if you often need to use sudo
, you might need to check if your virtual environment setup is correct.
The Ultimate Weapon for Project Management
Now that you've mastered the basic usage of virtual environments, let's look at how to apply them to actual project management. Trust me, this is definitely the ultimate weapon to improve your work efficiency!
Creating Separate Virtual Environments for Different Projects
It's a good habit for each project to have its own virtual environment. Suppose you have two projects, one is a web application and the other is a data analysis project, you can do this:
-
Create a virtual environment for the web application:
python -m venv web_project_env
-
Create a virtual environment for the data analysis project:
python -m venv data_analysis_env
This way, each project has its own "small world" where you can install the specific packages and versions needed.
I remember once, I was working on two projects simultaneously on the same machine: one using TensorFlow 1.x and the other needing TensorFlow 2.x. Without virtual environments, this would have been an almost impossible task. But with virtual environments, I could easily switch between the two projects without worrying about version conflicts. This was really a lifesaver!
Conclusion
Alright, my friends, today we've delved deep into the powerful tool of Python virtual environments. From basic concepts to practical applications, we've mastered how to use virtual environments to simplify project management, resolve dependency conflicts, and improve development efficiency.
Remember, using virtual environments is not only a good habit but also an indispensable skill in modern Python development. It can make your development process smoother, your project structure clearer, and also provide convenience for team collaboration.
So, are you ready to try using virtual environments in your next project? Or if you're already using virtual environments, do you have any insights or experiences you'd like to share? I'd love to hear your thoughts and experiences!
Finally, I want to say that technology is constantly advancing, and new tools and methods are constantly emerging. Maintaining enthusiasm for learning and being brave to try new things is the key to becoming an excellent developer. Let's continue to explore together in the world of Python and create more exciting code!
Do you have any more questions about virtual environments? Or do you have any unique tips for using virtual environments that you'd like to share? Feel free to leave a comment, let's discuss and progress together!
Next
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.
Next
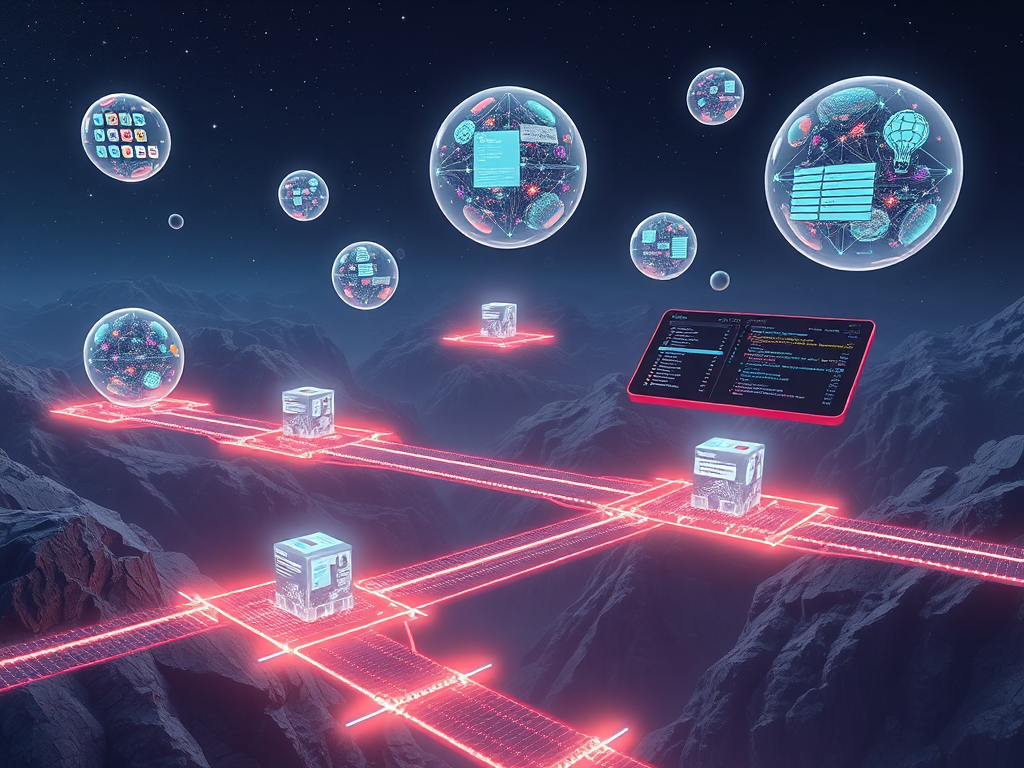
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
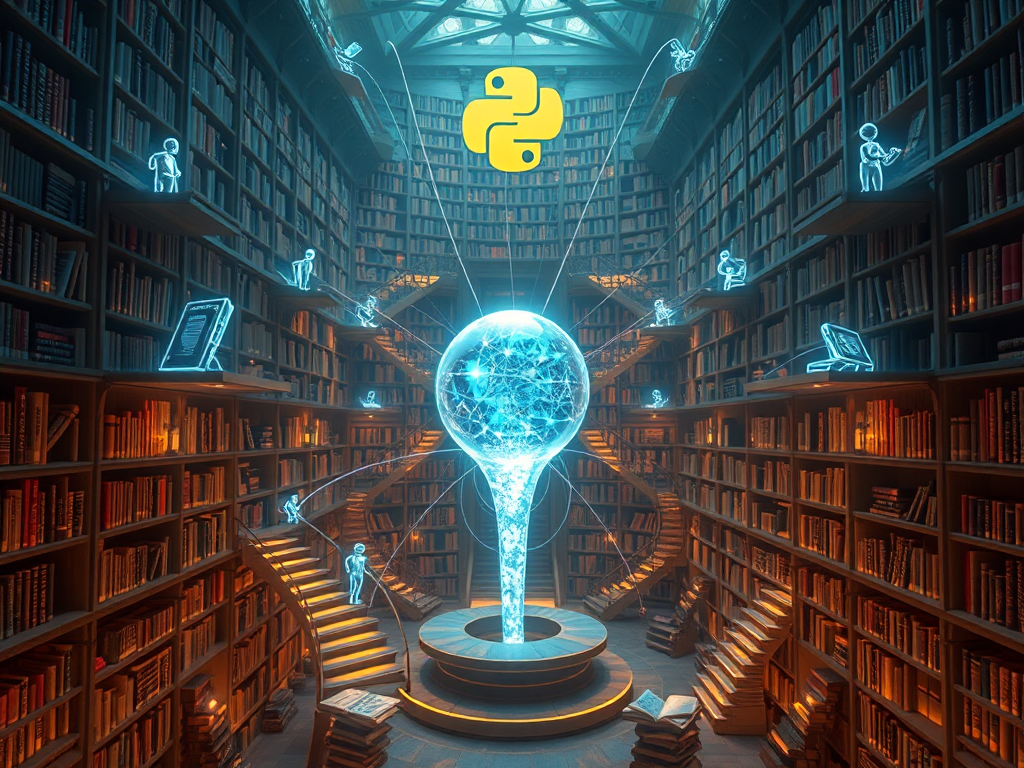
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
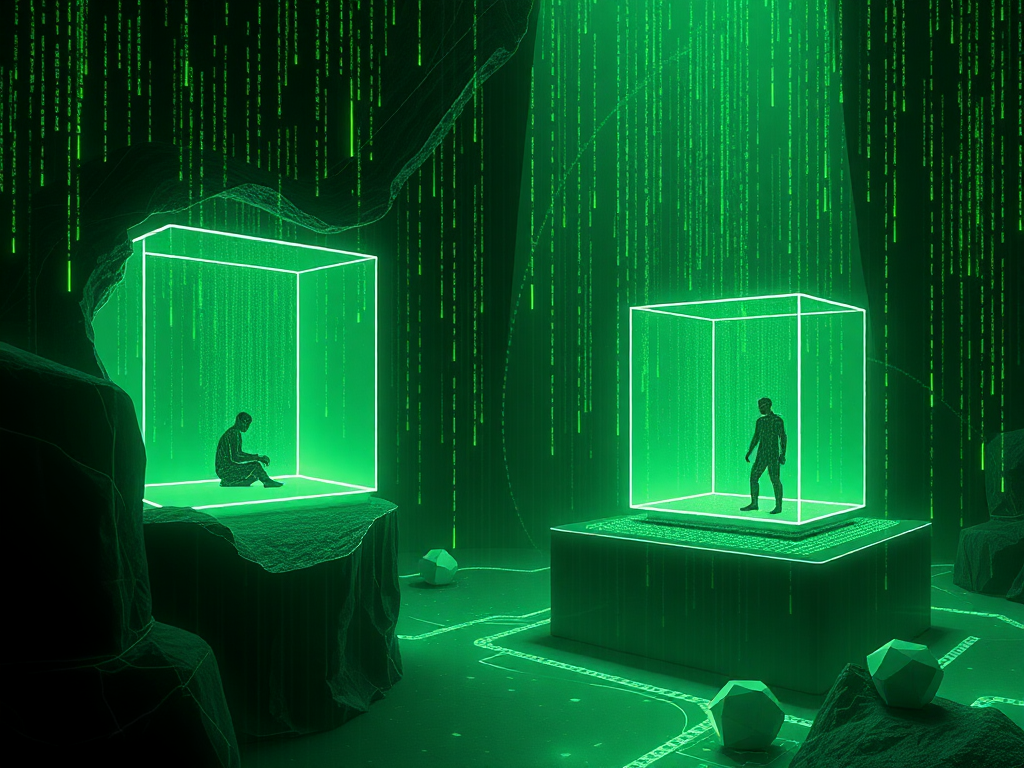
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.