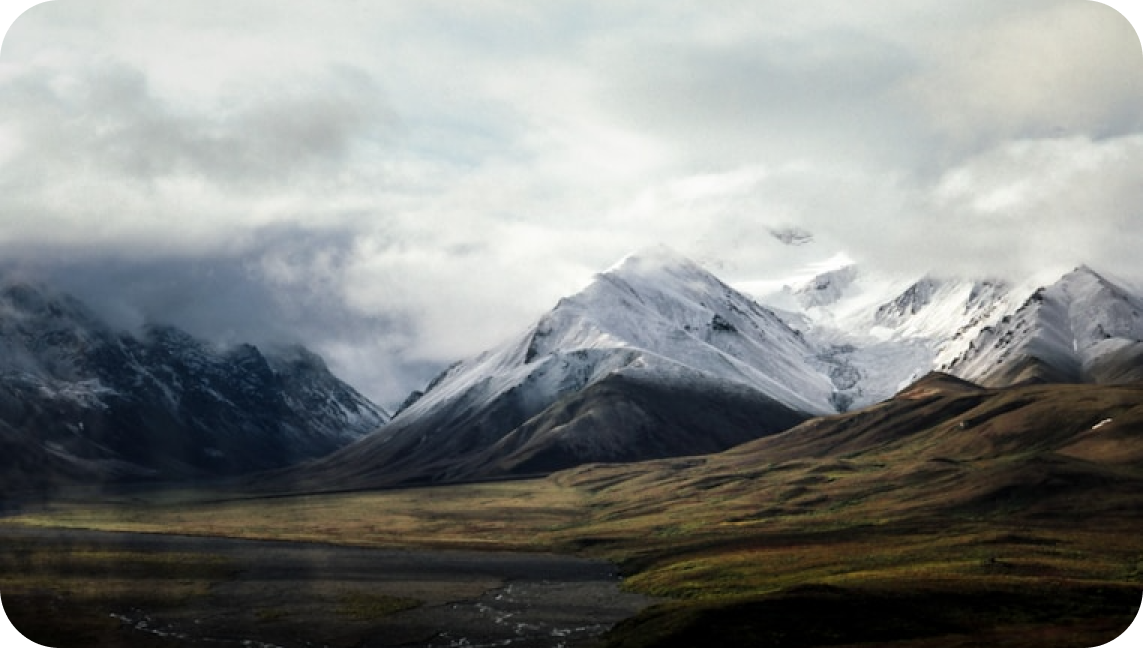
Introduction
Have you ever encountered situations where a Python project runs perfectly on your computer but throws errors on someone else's? Or installed a new package only to find that previously working projects suddenly stop functioning? These issues occur due to not using virtual environments.
As a Python developer, I deeply understand the importance of virtual environments. I remember once when developing a data analysis project, I needed TensorFlow 1.x, but another machine learning project required TensorFlow 2.x. Without virtual environments, these two projects couldn't coexist harmoniously on the same computer.
Today, I'll discuss Python virtual environments in detail. After reading this article, you'll understand why virtual environments are indispensable tools in Python development.
Getting Started
Think of a virtual environment as an independent room. In this room, you can install various Python packages without affecting other rooms or the entire house. It's like decorating your own room without impacting others' rooms.
Here's a vivid example: Imagine you're developing three projects: - A website using Django 2.2 - An API service requiring Django 3.2 - A new project needing Django 4.0
Without virtual environments, you could only install one Django version globally, which clearly wouldn't meet the requirements. But with virtual environments, you can create independent environments for each project, avoiding interference.
Principles
To deeply understand virtual environments, we need to discuss Python's package management mechanism. When you install Python packages, they're typically installed in the global site-packages directory. On Windows, this directory is usually at:
C:\Users\<username>\AppData\Local\Programs\Python\Python3x\Lib\site-packages
On Linux/macOS, it might be at:
/usr/lib/python3.x/site-packages
The core principle of virtual environments is: they create an independent copy of the Python interpreter and maintain a separate package directory. When you activate a virtual environment, the system temporarily modifies environment variables, making the Python interpreter prioritize packages in the virtual environment over the global environment.
Practice
After discussing theory, let's get hands-on. Since Python 3.3, the virtual environment tool venv has been integrated into the standard library, meaning you can use it without additional installation.
First, let's create a virtual environment:
python -m venv myproject_env
This command creates a folder named myproject_env in the current directory, containing a Python interpreter copy and necessary files.
Next, we need to activate this virtual environment. The activation command differs by operating system:
On Windows:
myproject_env\Scripts\activate
On Linux/macOS:
source myproject_env/bin/activate
After activation, you'll see (myproject_env) prefix in your command prompt, indicating you're in the virtual environment.
Let's do an experiment. First, install a specific package version:
pip install requests==2.25.1
Then create a simple test script test.py:
import requests
print(requests.__version__)
response = requests.get('https://api.github.com')
print(response.status_code)
Running this script will show version 2.25.1. Now, let's deactivate the virtual environment:
deactivate
If you have a different version of requests installed globally, running the script again will show a different version number. This demonstrates the isolation effect of virtual environments.
Advanced Usage
In real development, we often need to record project dependencies and their versions. This is where requirements.txt comes in handy:
pip freeze > requirements.txt
This command exports version information of all packages in the current environment to requirements.txt. When other developers need to recreate your environment, they just need:
pip install -r requirements.txt
I've found a useful tip in daily development: creating different requirements files for different development stages. For example:
- requirements-dev.txt: packages needed for development
- requirements-test.txt: packages needed for testing
- requirements-prod.txt: packages needed for production
This helps better manage dependencies across different environments.
Common Issues
You might encounter some issues when using virtual environments. Here are some common problems and solutions I've summarized:
- Virtual Environment Activation Fails Usually due to permission or path issues. Solutions:
- Run command prompt as administrator on Windows
-
Check file permissions on Linux/macOS: chmod +x activate
-
Pip Package Installation Fails Could be network issues or version conflicts. Solutions:
- Use domestic mirrors: pip install -i https://pypi.tuna.tsinghua.edu.cn/simple package_name
-
Clear pip cache: pip cache purge
-
Virtual Environment Too Large Might be due to too many unnecessary packages. Solutions:
- Regularly clean unused packages: pip uninstall package_name
- Use pipdeptree to view dependency tree, remove unused dependencies
Tools
Besides built-in venv, there are other excellent virtual environment management tools:
- virtualenv The earliest virtual environment tool with more features:
pip install virtualenv
virtualenv myenv
- conda Especially suitable for data science projects:
conda create -n myenv python=3.8
conda activate myenv
- pipenv Combines pip and virtualenv functionality:
pip install pipenv
pipenv install requests
- poetry Modern Python project dependency management tool:
pip install poetry
poetry new myproject
Each tool has its characteristics. I suggest choosing based on project requirements. For example, conda is good for data science projects; if you want modern dependency management, poetry is worth trying.
Recommendations
Based on my years of Python development experience, here are some recommendations for using virtual environments:
- Project Naming Conventions Use meaningful virtual environment names, like:
- project_name-py38
-
project_name-dev This clearly indicates the environment's purpose and Python version.
-
Environment Isolation Strategy
- Development environment: includes all development tools
- Testing environment: only test-required packages
-
Production environment: minimized dependency set
-
Dependency Management
- Regular package updates
- Use version locking (pip freeze)
-
Frequent security update checks
-
Workflow Suggestions
- Create virtual environment immediately for new projects
- Include requirements.txt in code repository
- Use .gitignore to exclude virtual environment directory
Future Outlook
Virtual environment technology continues evolving. We might see:
-
Smarter Dependency Resolution Current dependency resolution sometimes encounters version conflicts; future tools might provide smarter solutions.
-
Better Container Integration Virtual environments will integrate more tightly with container technologies like Docker.
-
Cross-language Support Tools might emerge that can manage dependencies for multiple programming languages simultaneously.
-
Cloud-Native Support Virtual environments might better support cloud development environments.
Conclusion
Looking back at this article, we've thoroughly explored Python virtual environments. From basic concepts to practical applications, from common issues to advanced techniques, you should now have a comprehensive understanding of virtual environments.
Remember, using virtual environments isn't just good practice; it's an essential skill in Python development. It helps build reliable, reproducible development environments, making your Python journey smoother.
How do virtual environments help your development work? Feel free to share your experiences and thoughts in the comments. If you have any questions, feel free to discuss.
Let's continue exploring the Python world together. See you in the next article.
Next
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.
Next
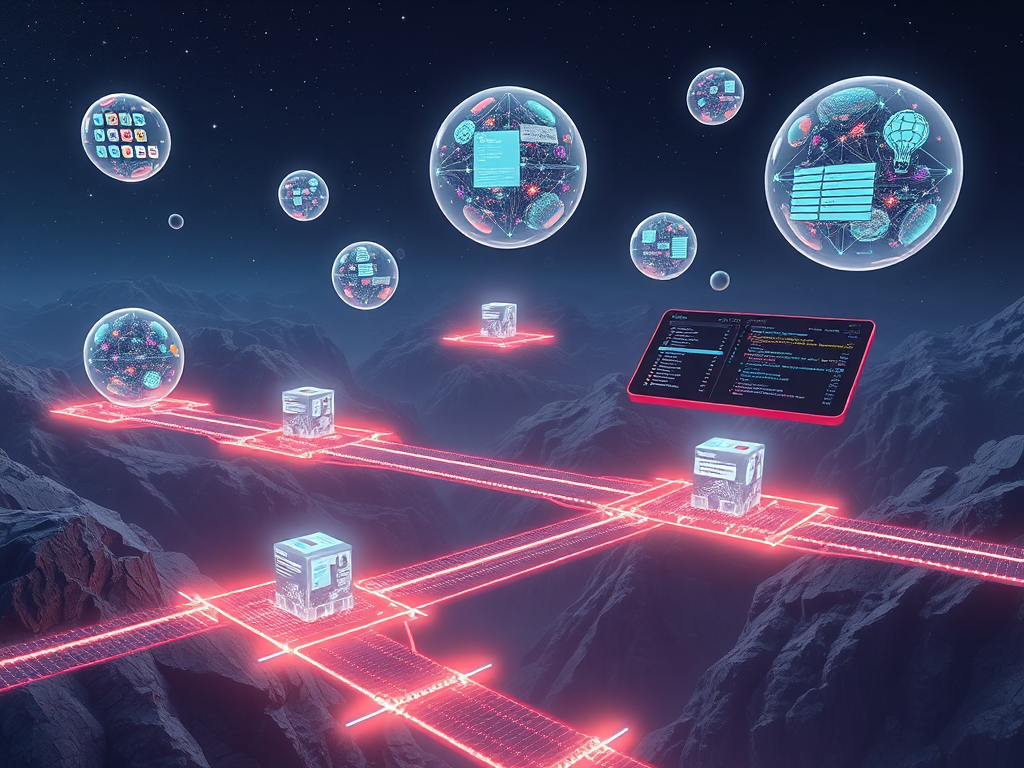
Python Virtual Environments: Your Secret Weapon for Project Development
An in-depth exploration of Python virtual environments, covering concepts, creation methods, usage techniques, and management strategies. Includes venv module setup, pip package management, environment replication and sharing, as well as exiting and deleting virtual environments.
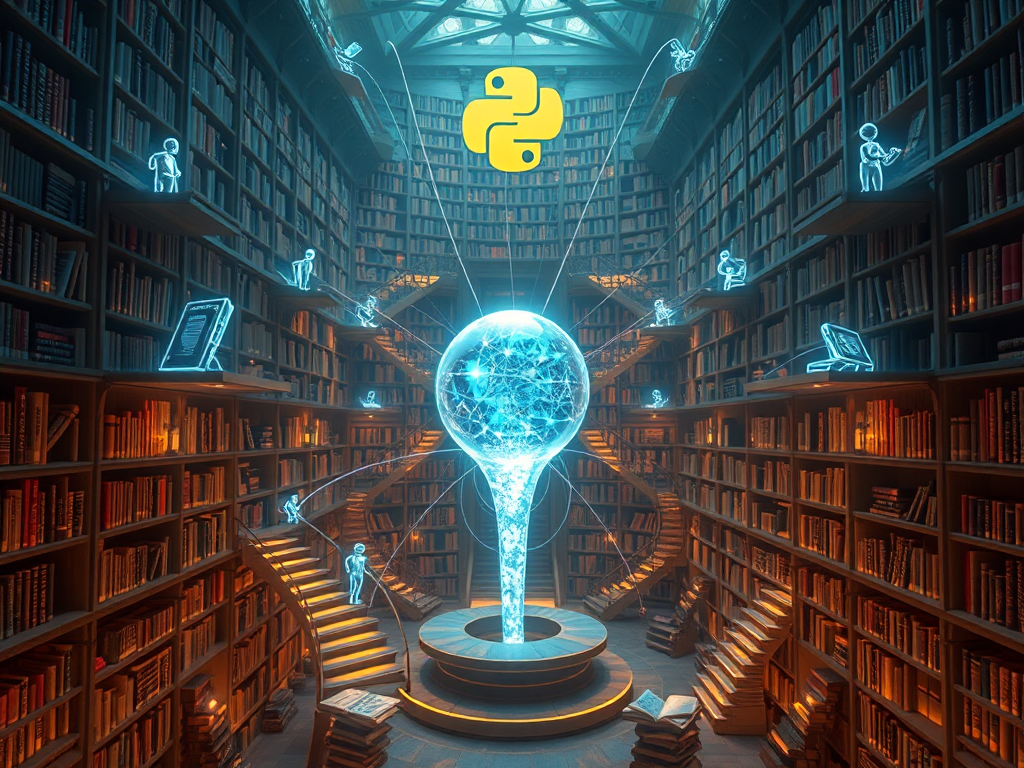
Basic Concepts of Python Virtual Environments
Python virtual environment is an isolation technique that creates independent Python environments for different projects, avoiding package version conflicts. Th
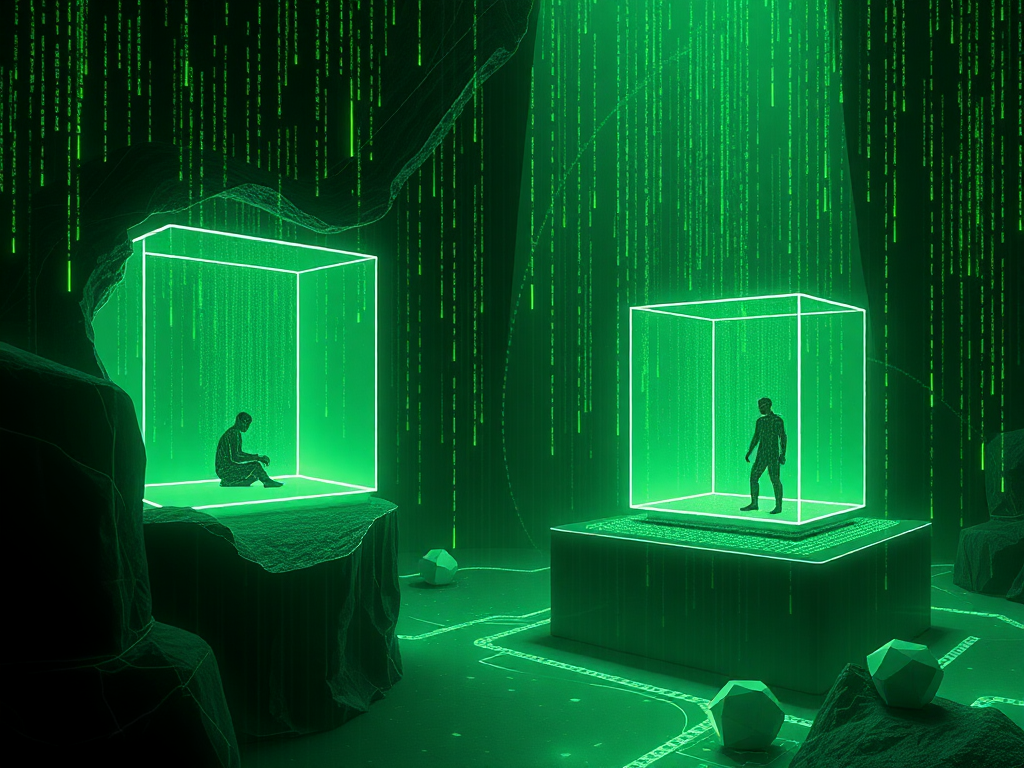
Python Virtual Environments: Making Your Project Dependency Management Easier and More Flexible
An in-depth guide to Python virtual environments, covering concepts, benefits, and usage. Learn techniques for creating and managing virtual environments, along with strategies for solving common issues to enhance Python project portability and maintainability.