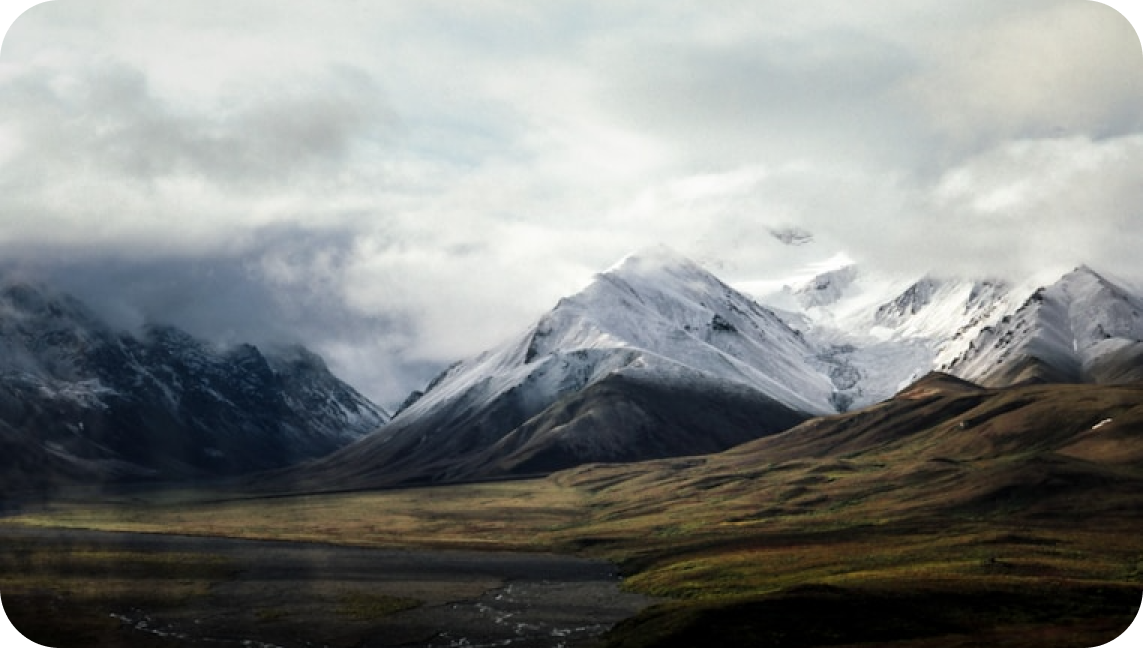
Hello, Python enthusiasts! Today, let’s talk about a fascinating and powerful feature in Python—decorators. Have you ever wondered how to add new functionality to a function without modifying its original code? Or how to elegantly handle some repetitive code logic? If these questions intrigue you, then decorators are the Python "magic" you can’t miss!
What is a Decorator?
As the name suggests, a decorator is like putting a beautiful outfit on your function. It allows you to add extra functionality without changing the original function code. Sounds magical, right? Let’s gradually unveil the mystery of decorators.
First, we need to understand an important concept: in Python, functions are objects. This means we can pass functions as parameters and return them as values. This characteristic lays the foundation for implementing decorators.
Here’s a simple example:
def greet(name):
return f"Hello, {name}!"
def shout(func):
def wrapper(name):
return func(name).upper()
return wrapper
greet = shout(greet)
print(greet("Alice")) # Output: HELLO, ALICE!
In this example, the shout
function is a decorator. It takes a function as a parameter and returns a new function. The new function (wrapper
) calls the original function and processes its result (in this case, converting it to uppercase).
Decorator Syntax Sugar
Python offers a more elegant way to use decorators, which is by using the @
symbol. Let’s rewrite the example above:
def shout(func):
def wrapper(name):
return func(name).upper()
return wrapper
@shout
def greet(name):
return f"Hello, {name}!"
print(greet("Bob")) # Output: HELLO, BOB!
See? By using @shout
, we can apply the decorator directly before the function definition. This approach is more concise and clear, isn’t it cool?
Practical Applications of Decorators
After discussing so much theory, you might ask: what’s the use of decorators in actual development? Let me give you a few examples.
1. Timer Decorator
Suppose we want to know the execution time of a function; we can create a timer decorator:
import time
def timer(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} execution time: {end_time - start_time:.5f} seconds")
return result
return wrapper
@timer
def slow_function():
time.sleep(2)
print("Function completed")
slow_function()
This decorator can help us easily measure the execution time of any function without adding timing code to each function. Isn’t it convenient?
2. Logging Decorator
During development, we often need to log function call information. Using decorators can simplify this process:
import logging
logging.basicConfig(level=logging.INFO)
def log_function_call(func):
def wrapper(*args, **kwargs):
logging.info(f"Calling function: {func.__name__}")
return func(*args, **kwargs)
return wrapper
@log_function_call
def add(a, b):
return a + b
result = add(3, 5)
print(f"Result: {result}")
With this decorator, we can easily add logging functionality to any function without modifying its code.
3. Cache Decorator
For some computationally intensive functions, we can use caching to improve performance:
def memoize(func):
cache = {}
def wrapper(*args):
if args in cache:
return cache[args]
result = func(*args)
cache[args] = result
return result
return wrapper
@memoize
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(100)) # This calculation will be fast
This decorator will cache the function’s results, allowing us to return cached results directly when the function is called with the same parameters again, without recalculating.
Decorators with Parameters
Sometimes, we may want the decorator itself to accept parameters. This requires us to wrap another layer of functions:
def repeat(times):
def decorator(func):
def wrapper(*args, **kwargs):
for _ in range(times):
result = func(*args, **kwargs)
return result
return wrapper
return decorator
@repeat(3)
def greet(name):
print(f"Hello, {name}!")
greet("Charlie") # Prints "Hello, Charlie!" three times
In this example, repeat
is a decorator that can accept parameters. It returns a decorator that makes the decorated function execute the specified number of times.
Class Decorators
In addition to function decorators, Python also supports class decorators. Class decorators can be used to modify class behavior:
def singleton(cls):
instances = {}
def get_instance(*args, **kwargs):
if cls not in instances:
instances[cls] = cls(*args, **kwargs)
return instances[cls]
return get_instance
@singleton
class Database:
def __init__(self):
print("Database connection initialized")
db1 = Database()
db2 = Database() # Will not initialize again
print(db1 is db2) # Output: True
This example shows how to use a class decorator to implement the singleton pattern. No matter how many times we create a Database
instance, it always returns the same object.
Considerations for Decorators
While decorators are powerful, there are some issues to be aware of:
-
Function Metadata: Decorators change the metadata of the decorated function (such as function name, docstring, etc.). You can use
functools.wraps
to preserve the original function metadata. -
Performance Impact: Overuse of decorators may affect program performance, as each function call adds a layer of indirection.
-
Debugging Difficulty: Using decorators can make debugging more difficult, as the actual executing code is wrapped in the decorator.
-
Readability: Although decorators can make the code more concise, overuse may reduce code readability.
Conclusion
Decorators are a powerful and flexible feature in Python. They can help us write more concise and maintainable code. By using decorators wisely, we can achieve code reuse, separation of concerns, and functionality enhancement.
Do you find decorators interesting? Have you thought about where you can apply decorators in your projects? I hope this article inspires your creativity and helps you go further on your Python programming journey.
Remember, programming is like magic, and decorators are one of the powerful spells. Master it well, and you can become an outstanding Python wizard!
Let’s continue exploring the wonderful world of Python together! If you have any questions or ideas, feel free to leave a comment for discussion. The joy of programming lies in continuous learning and sharing, doesn’t it?
Next
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit
Next
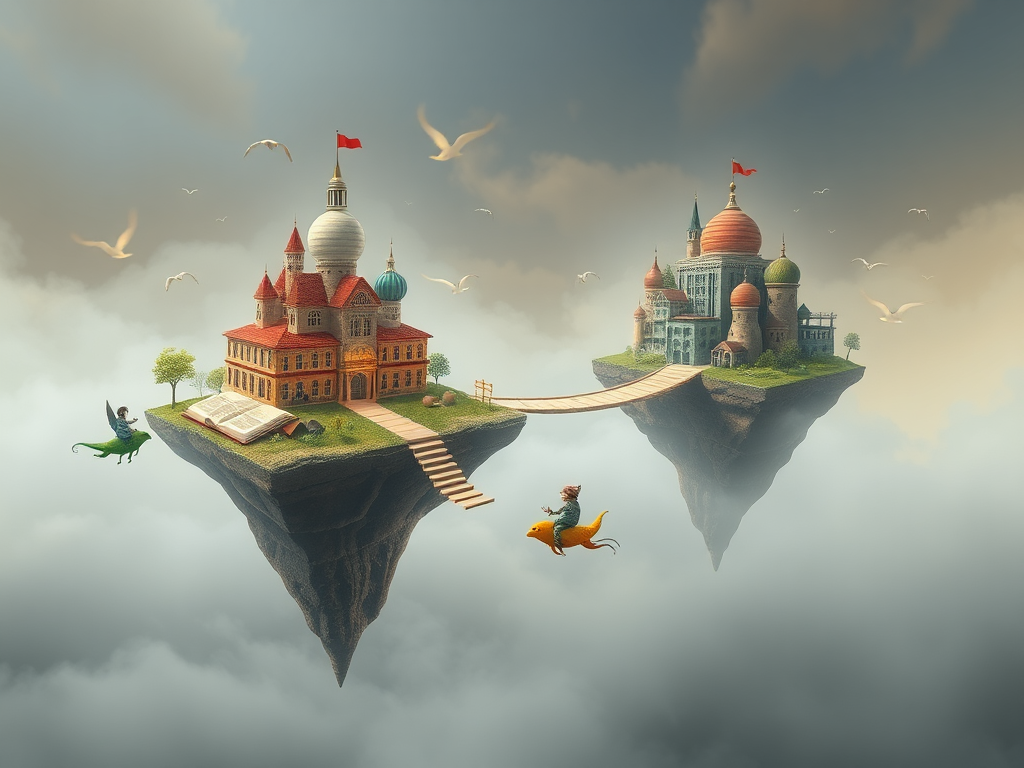
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
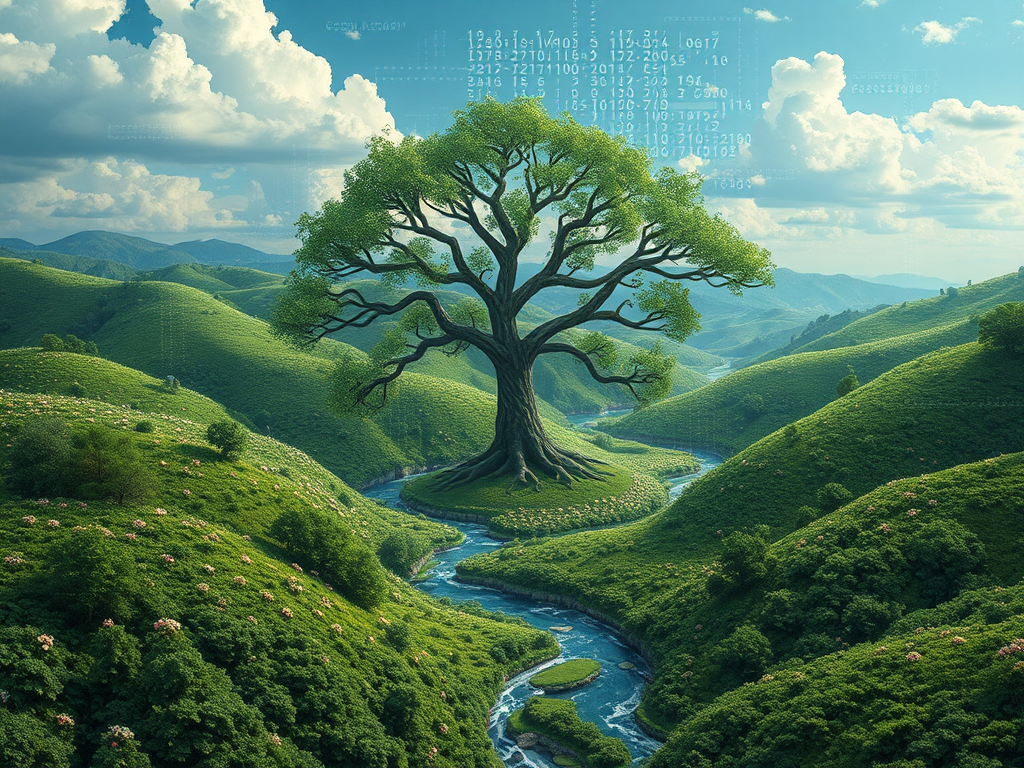
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
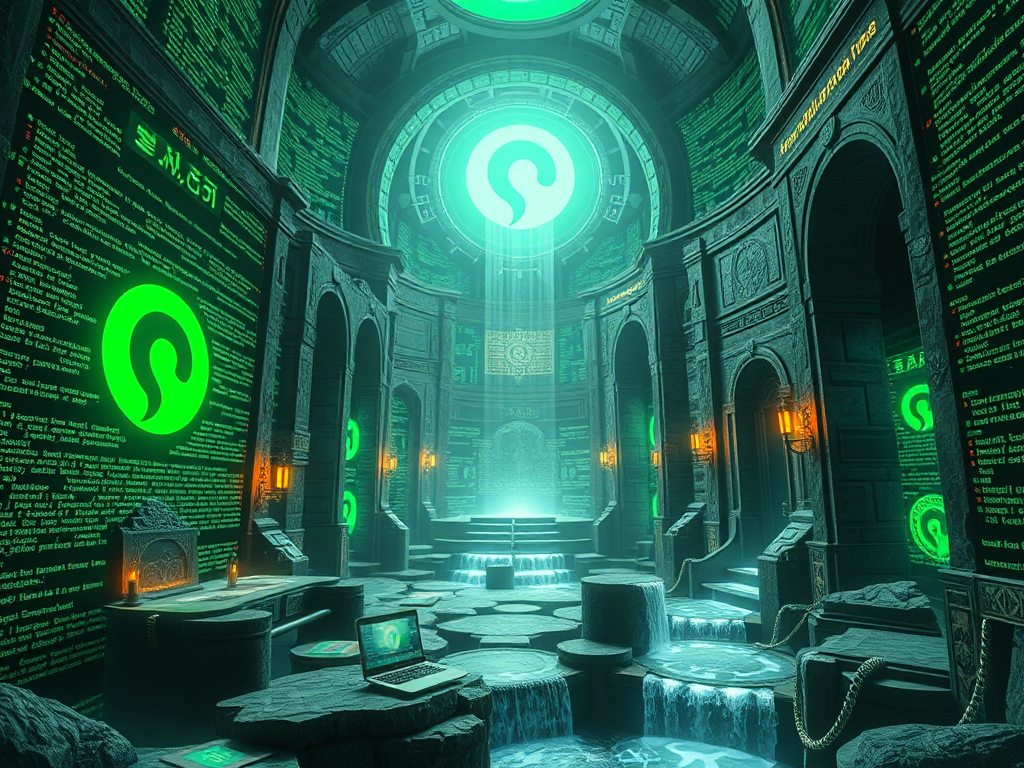
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit