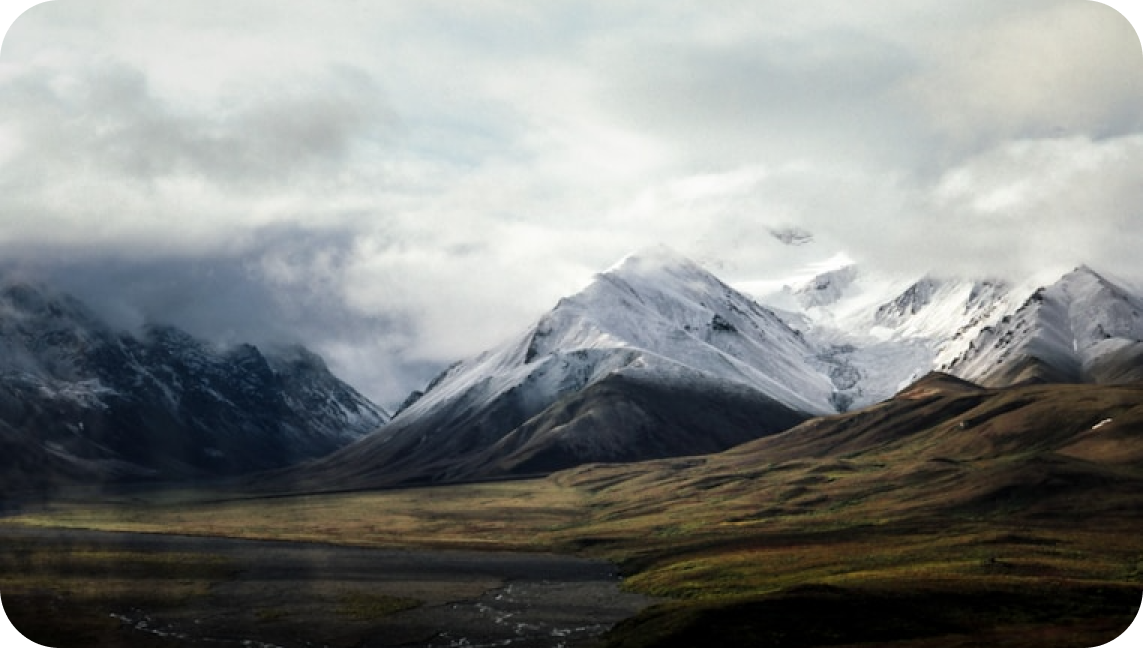
Hello everyone, today let's talk about some practical tips for Python Web development! As a Python blogger, I often search online for various development issues and solutions, and share my experiences and insights with everyone. Today, we'll review several common problems you might encounter in the web development process, and how to cleverly solve them using Python. Are you ready? Let's begin!
Image Upload and Storage in FastAPI
Let's first look at how to handle image upload and storage issues in FastAPI projects. I saw a question like this on Stack Overflow:
"Users encountered problems when uploading images to S3 using FastAPI and SQLAlchemy ORM, and storing the URL in a PostgreSQL database."
For this situation, I suggest using the boto3
library to handle S3 upload operations, while ensuring that the image URL is correctly stored in the database. The specific steps are as follows:
- Create an image upload endpoint in the FastAPI application;
- Use the
boto3
library to upload the image to the S3 bucket; - Store the image URL returned by S3 in the PostgreSQL database.
Here's a sample code for your reference:
from fastapi import UploadFile, File
import boto3
@app.post("/upload")
async def upload_image(file: UploadFile = File(...)):
s3 = boto3.client('s3')
bucket_name = 'my-bucket'
key = f'images/{file.filename}'
s3.upload_fileobj(file.file, bucket_name, key)
url = f'https://{bucket_name}.s3.amazonaws.com/{key}'
# Store URL in the database
image = Image(url=url)
db.session.add(image)
db.session.commit()
return {"url": url}
In this way, we can elegantly solve the problem of image upload and storage. You see, using Python and the right libraries, handling common tasks in web development is so simple and efficient!
Complex Queries in SQLAlchemy
Next, let's look at how to implement complex SQL queries in SQLAlchemy. I found a question like this on Stack Overflow:
"The user wants to perform a left join in SQLAlchemy and use a subquery in the ON clause."
For this situation, we can use aliased
and subquery
to implement complex join operations. Here's a sample code:
from sqlalchemy.orm import aliased
subquery = session.query(OtherModel).filter(OtherModel.some_field == value).subquery()
result = session.query(MainModel).outerjoin(subquery, MainModel.id == subquery.c.id).all()
In this code, we first create a subquery subquery
, then left join it with the main query. In this way, we can use subqueries in the ON clause.
Isn't it cool? I personally think SQLAlchemy is really powerful in handling complex queries. However, if you're not very familiar with SQL, you might find it a bit challenging at first. So, my advice is to practice more, practice makes perfect!
Solving Data Type Conversion Problems
Speaking of database operations, we inevitably encounter data type conversion problems. For example, I saw a question like this on Stack Overflow:
"Users encountered difficulties when trying to convert integers to boolean values in SQLite."
For this situation, I suggest using a CASE statement to perform type conversion. Here's the code:
SELECT CASE WHEN integer_column = 1 THEN TRUE ELSE FALSE END AS boolean_column
FROM table_name;
In this way, we can convert an integer column to a boolean type. Isn't it simple and practical?
Of course, different databases may have different support for data type conversion. So, when performing type conversion operations, it's best to consult the relevant documentation first to understand the specific approach.
AWS Service Integration Tips
In the web development process, we often need to integrate with various cloud services. For example, when uploading images, we may need to store the images in an AWS S3 bucket. At this time, we can use the boto3
library to interact with S3.
In addition, if you encounter a 404 error when reading files from an S3 bucket in an AWS Lambda function, you can check if the bucket name and object key are correct, and ensure that the Lambda function has the appropriate permissions to access S3. You can also use the head_object
method of boto3
to verify if the object exists.
Besides S3, we sometimes need to integrate with other AWS services, such as the SES email service. If you encounter a connection timeout error when sending emails through Gmail, you can check the firewall settings and network connection to ensure that the SMTP port is not blocked. Also, make sure to use the correct Gmail SMTP settings and consider using an application-specific password for authentication.
By mastering these techniques, I believe you'll be better equipped to solve various problems that may arise during the AWS service integration process.
Summary
Alright, that's all for today. Through the above sharing, I believe you now have a deeper understanding of some common issues in Python Web development. However, this is just the tip of the iceberg. In actual development, we will encounter all kinds of challenges.
So, my advice is to maintain curiosity and be diligent in hands-on practice. When you encounter problems, don't be discouraged. Learn to search for solutions online, such as on Stack Overflow. I believe that as long as you persist, you can definitely become an excellent Python Web developer!
Finally, if you have any other questions or ideas, feel free to share and discuss with me anytime. Looking forward to chatting with you about Python development in the next issue!
Next
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit
Next
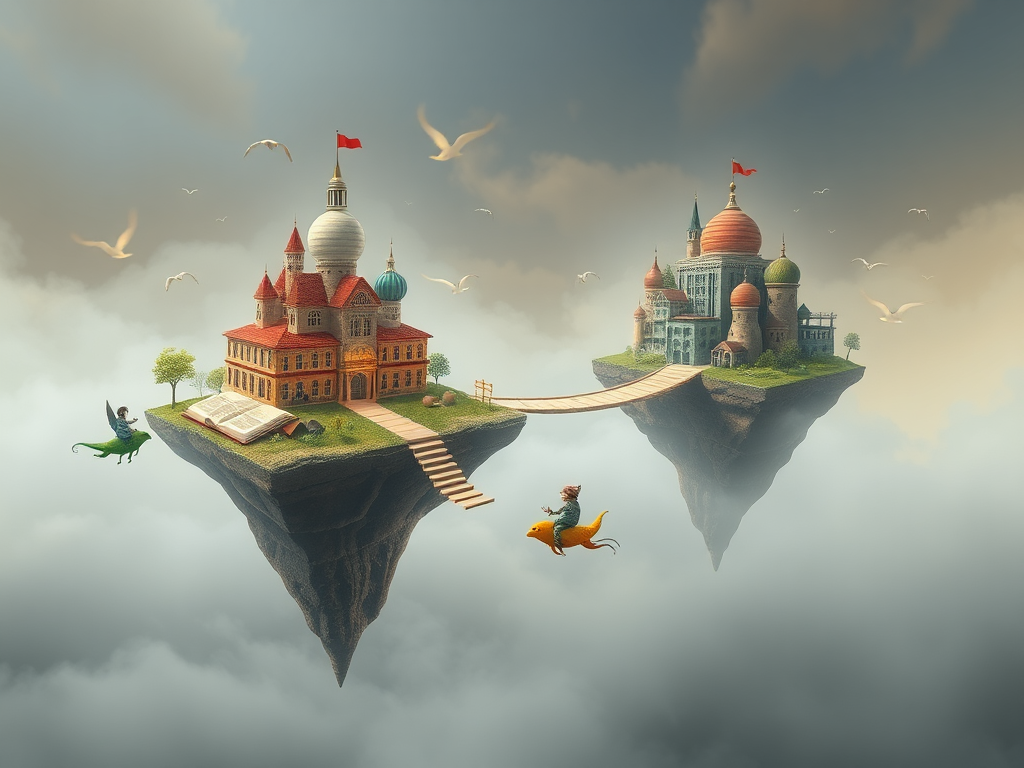
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
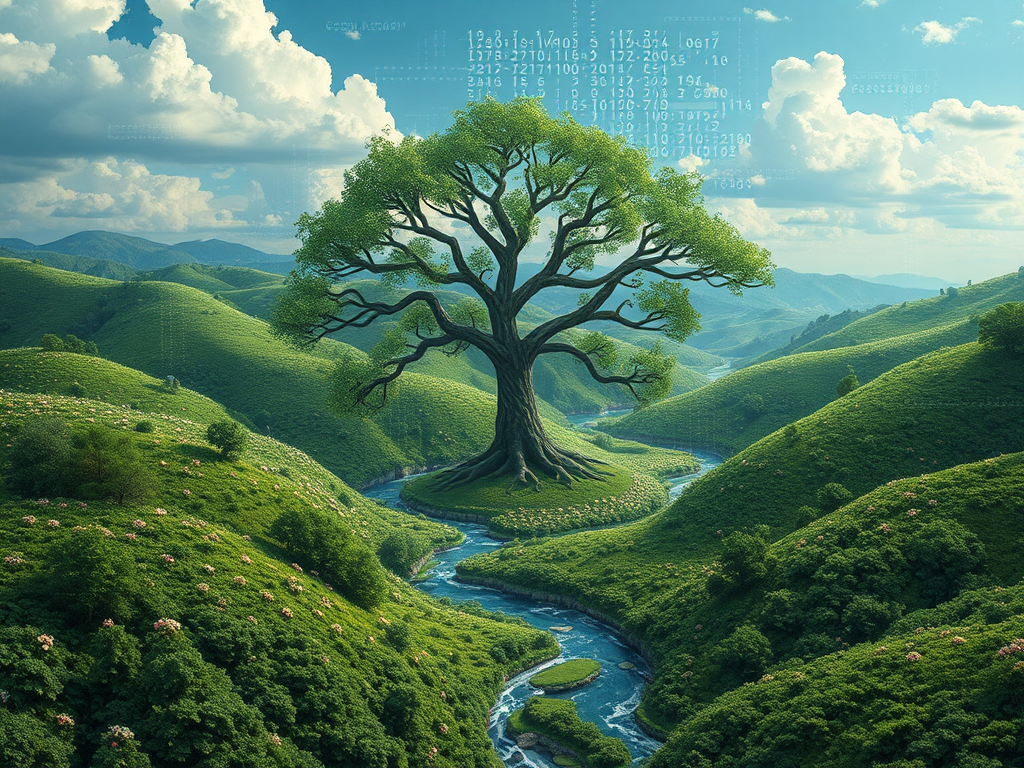
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
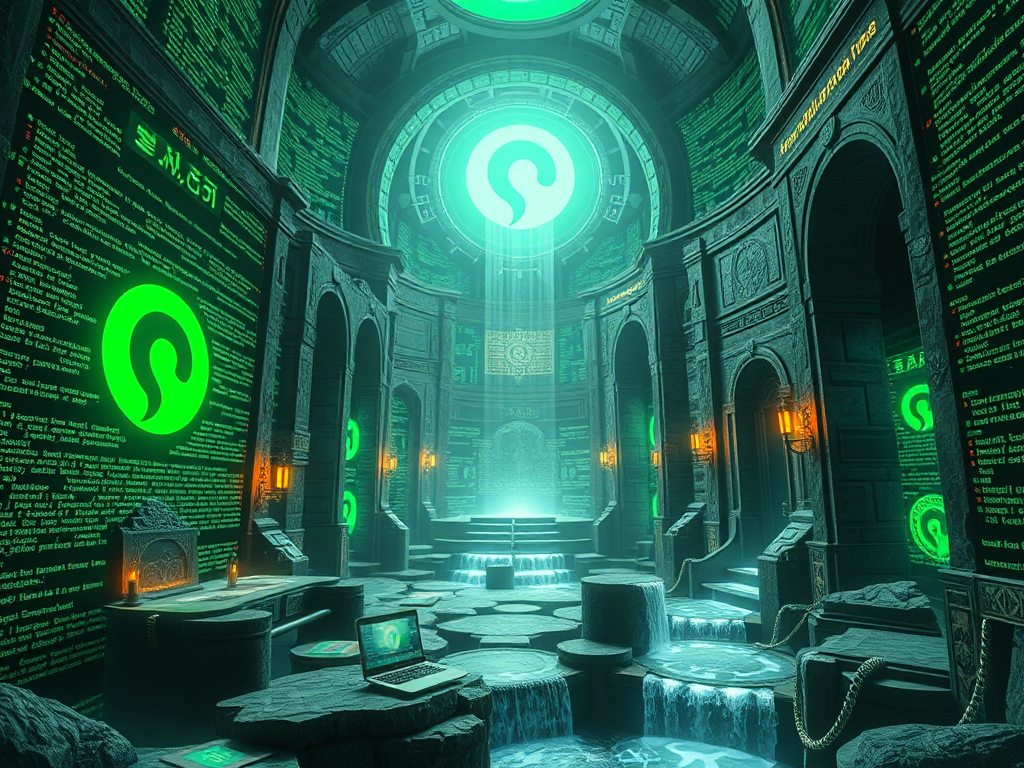
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit