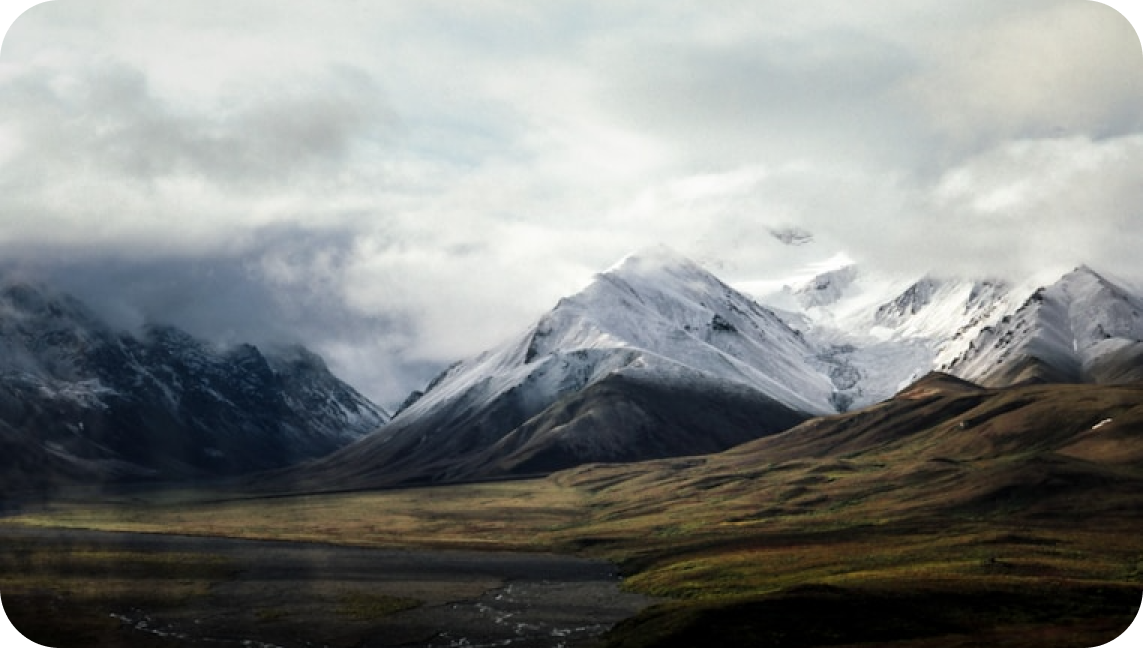
As a Python developer, have you encountered situations where database connections become increasingly slow as concurrent requests grow, or even experience connection timeouts? Today, I want to share with you a powerful tool that can thoroughly solve this problem — database connection pools. Through this article, you will gain a deep understanding of how connection pools work and master various techniques for using connection pools in real projects.
Understanding Connection Pools
Remember the first time I encountered database connection pools? It was in an e-commerce project where database access became exceptionally slow as the user base grew. Later, by introducing connection pools, we not only solved the performance issues but also gave me a new perspective on database access.
Let me explain connection pools with a vivid example. Imagine you're running a restaurant where you hire a new chef every time a customer arrives - this would obviously be wasteful and inefficient. A better approach, like connection pools, is to prepare a certain number of chefs (database connections) in advance and then coordinate and reuse them.
Working Principle
The core concept of connection pools is connection reuse. Let's look at a simple example:
import asyncpg
import asyncio
async def create_connection_pool():
pool = await asyncpg.create_pool(
user='postgres',
password='password',
database='testdb',
host='localhost',
port=5432,
min_size=5,
max_size=20
)
return pool
This code creates a connection pool with a minimum of 5 connections and a maximum of 20 connections. When requests come in, the pool allocates from existing connections rather than creating new ones. This is like a chef scheduling system in a restaurant, flexibly adjusting the number of working chefs based on customer flow.
Performance Improvement
Before using connection pools, each database operation required establishing a new connection:
async def without_pool():
conn = await asyncpg.connect(
user='postgres',
password='password',
database='testdb',
host='localhost'
)
try:
await conn.execute("SELECT * FROM users")
finally:
await conn.close()
Code using connection pool:
async def with_pool(pool):
async with pool.acquire() as connection:
await connection.execute("SELECT * FROM users")
Comparing these two code snippets, you'll find the version using connection pools is more concise. Moreover, it avoids the overhead of frequently establishing and closing connections, which is particularly important in high-concurrency scenarios.
Practical Application
How should we properly use connection pools in real projects? I've summarized several key points:
Connection Pool Configuration
First is the connection pool configuration, which is the most basic and important part:
import asyncpg
import logging
from typing import Dict, List
class DatabasePool:
def __init__(self):
self._pool = None
self._config = {
'user': 'postgres',
'password': 'password',
'database': 'testdb',
'host': 'localhost',
'port': 5432,
'min_size': 5,
'max_size': 20,
'command_timeout': 60,
'max_queries': 50000,
'max_inactive_connection_lifetime': 300.0
}
async def initialize(self):
try:
self._pool = await asyncpg.create_pool(**self._config)
logging.info("Database pool initialized successfully")
except Exception as e:
logging.error(f"Failed to initialize database pool: {str(e)}")
raise
This configuration includes several important parameters: - min_size: minimum number of connections to maintain - max_size: maximum number of connections allowed - command_timeout: command execution timeout - max_queries: maximum number of queries per connection - max_inactive_connection_lifetime: maximum lifetime of idle connections
Connection Management
Good connection management is key to avoiding resource leaks:
class DatabaseManager:
def __init__(self, pool):
self.pool = pool
async def execute_query(self, query: str, *args) -> List[Dict]:
async with self.pool.acquire() as connection:
try:
async with connection.transaction():
result = await connection.fetch(query, *args)
return [dict(record) for record in result]
except Exception as e:
logging.error(f"Query execution failed: {str(e)}")
raise
Here we use context managers (async with) to ensure connections are properly released. We've also included transaction management and error handling.
Performance Monitoring
Monitoring connection pool status is crucial in production environments:
class PoolMonitor:
def __init__(self, pool):
self.pool = pool
async def get_pool_status(self) -> Dict:
return {
'size': self.pool.get_size(),
'free_size': self.pool.get_free_size(),
'active_connections': self.pool.get_size() - self.pool.get_free_size()
}
async def monitor_pool(self, interval: int = 60):
while True:
status = await self.get_pool_status()
logging.info(f"Pool status: {status}")
await asyncio.sleep(interval)
Practical Example
Let's look at a complete application example:
async def main():
# Initialize database pool
db_pool = DatabasePool()
await db_pool.initialize()
# Create database manager
db_manager = DatabaseManager(db_pool._pool)
# Create monitor
monitor = PoolMonitor(db_pool._pool)
# Start monitoring task
asyncio.create_task(monitor.monitor_pool())
# Execute query
users = await db_manager.execute_query(
"SELECT * FROM users WHERE age > $1",
18
)
# Process query results
for user in users:
print(f"Found user: {user['name']}, age: {user['age']}")
if __name__ == "__main__":
asyncio.run(main())
Performance Optimization
During my experience with connection pools, I discovered some performance improvement techniques:
Batch Operations
When executing multiple queries, using batch operations can improve efficiency:
async def batch_insert(pool, records: List[Dict]):
async with pool.acquire() as conn:
# Prepare batch insert statement
stmt = await conn.prepare(
"INSERT INTO users(name, age) VALUES($1, $2)"
)
# Execute batch operations within transaction
async with conn.transaction():
await asyncio.gather(
*[stmt.execute(record['name'], record['age'])
for record in records]
)
Connection Pool Optimization
Adjust connection pool parameters based on actual load:
async def optimize_pool(pool, load_factor: float):
current_size = pool.get_size()
active_connections = current_size - pool.get_free_size()
if active_connections / current_size > load_factor:
# Increase pool size
new_size = min(current_size * 2, pool._config['max_size'])
await pool.set_size(new_size)
logging.info(f"Pool size increased to {new_size}")
Summary and Future Outlook
Through this article, we've deeply explored the implementation and optimization of Python async database connection pools. From basic concepts to practical applications, from simple usage to performance tuning, I believe you now have a comprehensive understanding of connection pools.
Remember, choosing appropriate connection pool configurations is an ongoing optimization process. You need to adjust parameters based on your actual application scenarios, server performance, and concurrency requirements. Also, remember to monitor connection pool status to promptly identify and resolve issues.
What role do you think connection pools can play in your projects? Feel free to share your thoughts and experiences in the comments.
Finally, let's look forward to further developments in Python async database access technology. As technology evolves, we'll surely have more efficient data access solutions.
Next
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit
Next
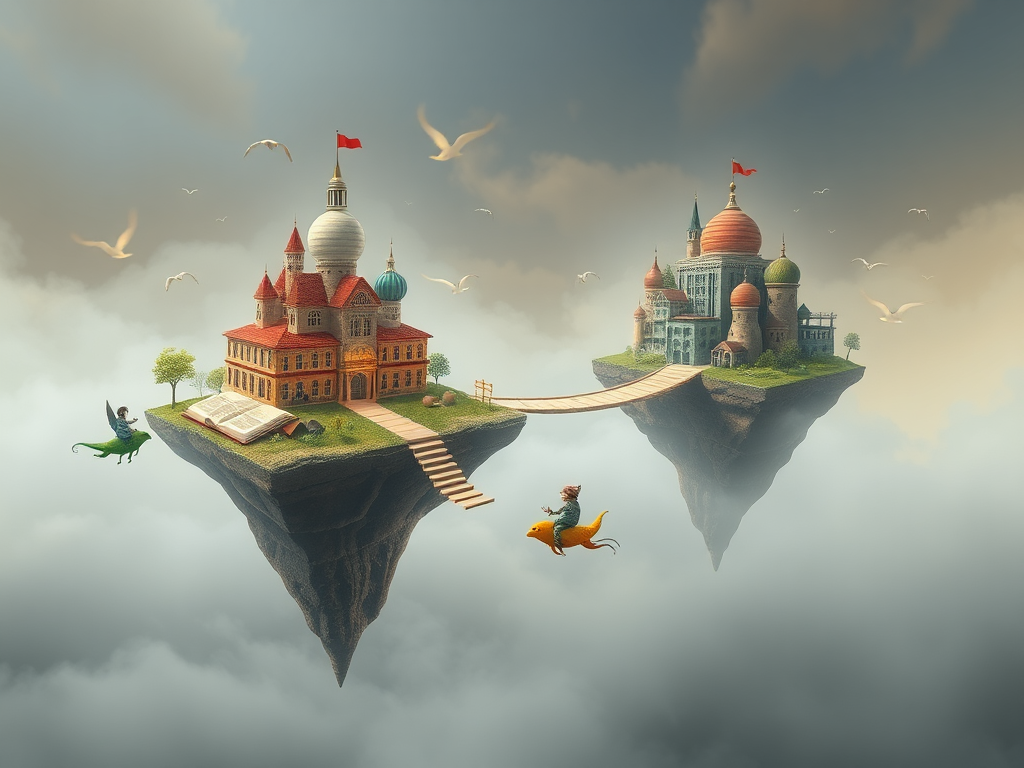
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
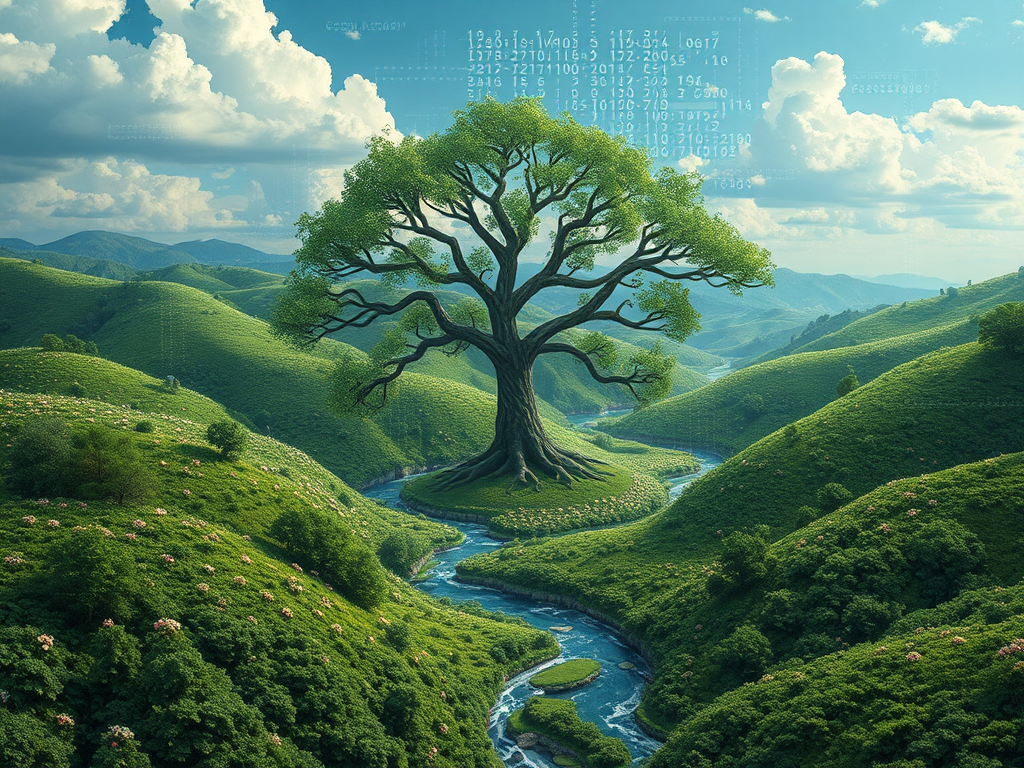
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
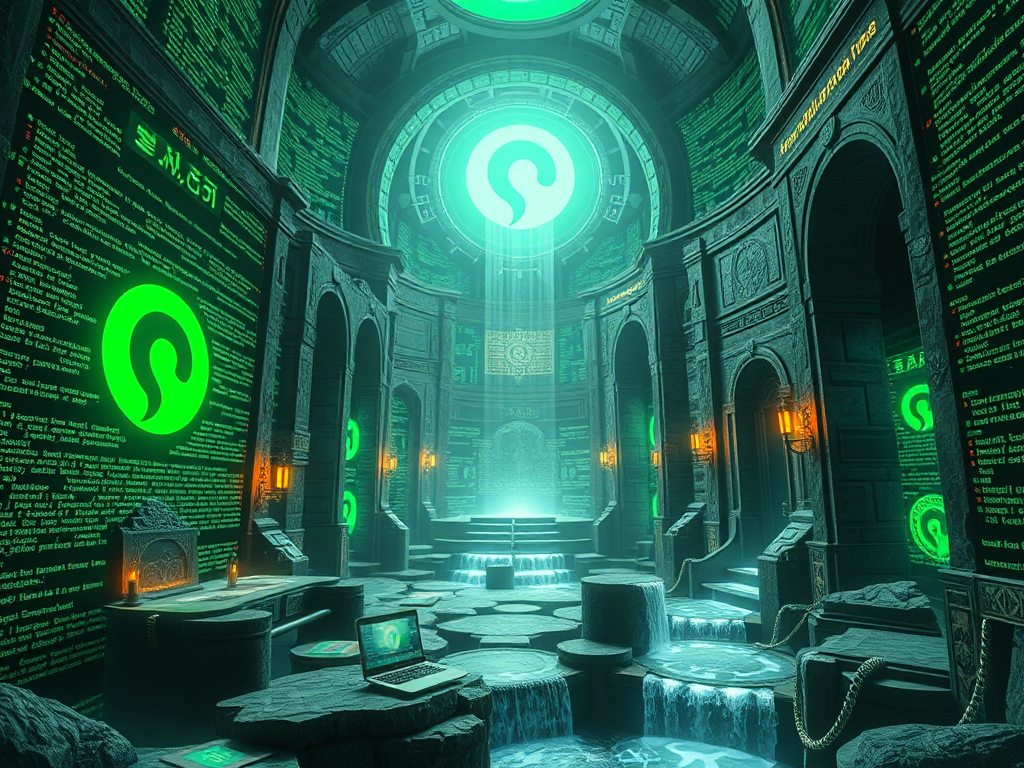
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit