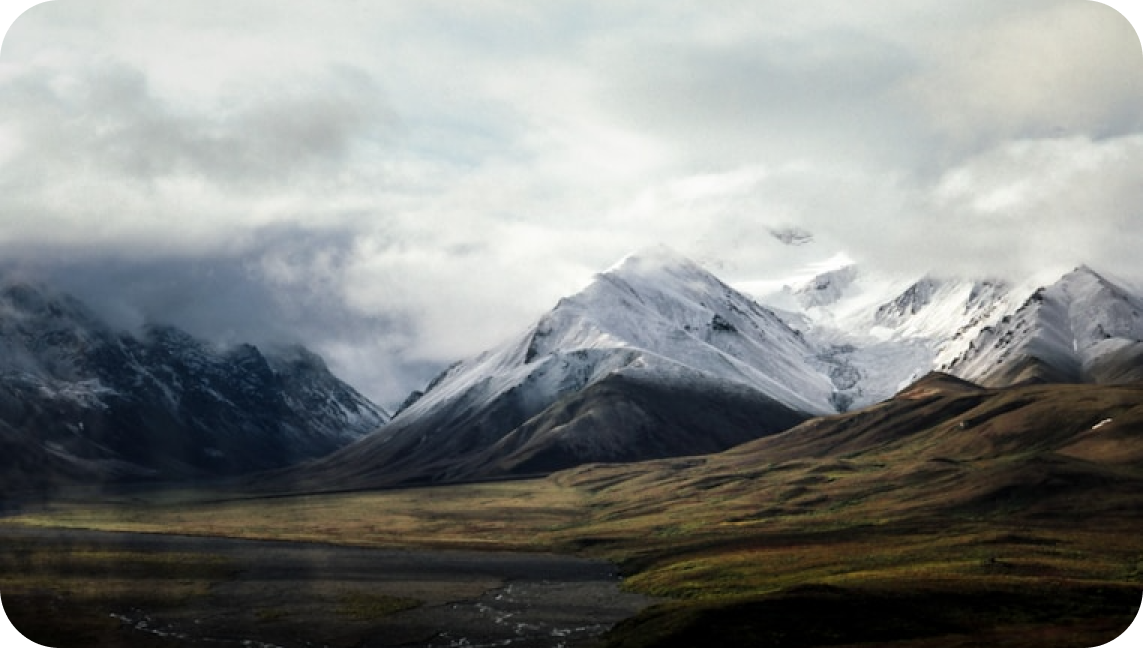
Background
As a Python programmer, have you ever wondered what else you can do besides data analysis and machine learning? Today I want to share an exciting field - Python Web Development. To be honest, when I first encountered web development, I was also confused. But after years of practice, I've deeply experienced Python's powerful advantages in web development.
Fundamentals
Before building web applications, we need to understand some basic concepts. Python, as a high-level programming language, has very friendly features. Do you know why Python is particularly suitable for web development? Let's take a look.
First is Python's dynamic typing system. This means we don't need to declare variable types in advance - Python handles this automatically at runtime. This feature allows us to focus more on implementing business logic rather than being bothered by tedious type declarations. I remember when developing with Java, I often had to write a lot of boilerplate code, while Python makes this process much more concise.
String name = "Python Developer";
Integer age = 25;
name = "Python Developer"
age = 25
Additionally, Python's automatic memory management is a major highlight. You don't need to manually manage memory like in C++ - Python's garbage collection mechanism handles these things automatically. This not only reduces the risk of memory leaks but also greatly improves development efficiency.
Frameworks
When it comes to web development, we must discuss framework choices. Django and Flask are Python's most popular web frameworks, each with its own characteristics.
Django is an all-in-one framework providing a complete web development solution. Its philosophy is "batteries included," with many common features built-in. For example, Django's Admin backend management system can generate a fully functional management interface with just a few lines of code, which can save a lot of time in development.
from django.contrib import admin
from .models import Article
@admin.register(Article)
class ArticleAdmin(admin.ModelAdmin):
list_display = ('title', 'author', 'publish_date')
search_fields = ('title', 'content')
Flask takes a different approach - it's a "micro-framework" with very minimal core functionality but can add various features through extensions. I particularly like Flask's design philosophy because it allows us to flexibly choose components based on project requirements.
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, Web Developer!'
Architecture
In actual development, a complete web application usually contains both frontend and backend components. The frontend handles the user interface, while the backend handles business logic and data storage. Python is mainly used for backend development, but through modern frameworks, we can also easily handle frontend tasks.
Let me share a real case. I once participated in developing an online education platform using Django as the backend framework. The architecture was like this:
from rest_framework import viewsets
from .models import Course
from .serializers import CourseSerializer
class CourseViewSet(viewsets.ModelViewSet):
queryset = Course.objects.all()
serializer_class = CourseSerializer
The frontend was built using Vue.js, interacting with the backend through APIs:
// Frontend code example
async function getCourses() {
try {
const response = await fetch('/api/courses/');
const courses = await response.json();
return courses;
} catch (error) {
console.error('Failed to get course list:', error);
}
}
Security
Security in web development is an eternal topic. I remember encountering SQL injection attacks in one project. Fortunately, Django provides good protection mechanisms.
Here are some common security threats and protection measures:
- SQL Injection Attacks: Use ORM or parameterized queries
query = "SELECT * FROM users WHERE username = '" + username + "'"
from django.db import models
class User(models.Model):
username = models.CharField(max_length=100)
User.objects.filter(username=username)
- XSS Attacks: Use template engine's automatic escaping
{{ user_input|escape }}
- CSRF Attacks: Enable CSRF middleware
MIDDLEWARE = [
'django.middleware.csrf.CsrfViewMiddleware',
]
Practice
After discussing so much theory, let's practice together. Let me guide you through implementing a simple blog system. This project will involve core features like user authentication, article management, and commenting functionality.
First, we need to design the data models:
from django.db import models
from django.contrib.auth.models import User
class Article(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
author = models.ForeignKey(User, on_delete=models.CASCADE)
created_at = models.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
class Comment(models.Model):
article = models.ForeignKey(Article, on_delete=models.CASCADE)
author = models.ForeignKey(User, on_delete=models.CASCADE)
content = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
Then, let's implement the view functions:
from django.shortcuts import render, get_object_or_404
from django.contrib.auth.decorators import login_required
from .models import Article, Comment
@login_required
def article_create(request):
if request.method == 'POST':
title = request.POST.get('title')
content = request.POST.get('content')
Article.objects.create(
title=title,
content=content,
author=request.user
)
return redirect('article_list')
return render(request, 'article_create.html')
Finally, set up URL routing:
from django.urls import path
from . import views
urlpatterns = [
path('', views.article_list, name='article_list'),
path('article/create/', views.article_create, name='article_create'),
path('article/<int:pk>/', views.article_detail, name='article_detail'),
]
Future Outlook
Web development is a constantly evolving field. We've seen Python's advantages in web development, but technology stack choices should still be based on specific project requirements. For beginners, I recommend starting with Django because it has excellent documentation and strong community support.
Did you know? According to Stack Overflow's 2023 Developer Survey, Python has consistently ranked among the most popular programming languages for years, and Django also ranks high among web frameworks. This indicates that Python web development has a future full of opportunities.
Afterword
At this point, I wonder if you've developed an interest in Python web development? If you want to learn more, I suggest mastering Python's basic syntax first, then choosing a framework to start practicing. Remember, the best way to learn programming is by working on projects.
Do you have any thoughts or questions? Feel free to discuss in the comments section. If this article has been helpful, please share it with other friends interested in Python web development.
Next
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit
Next
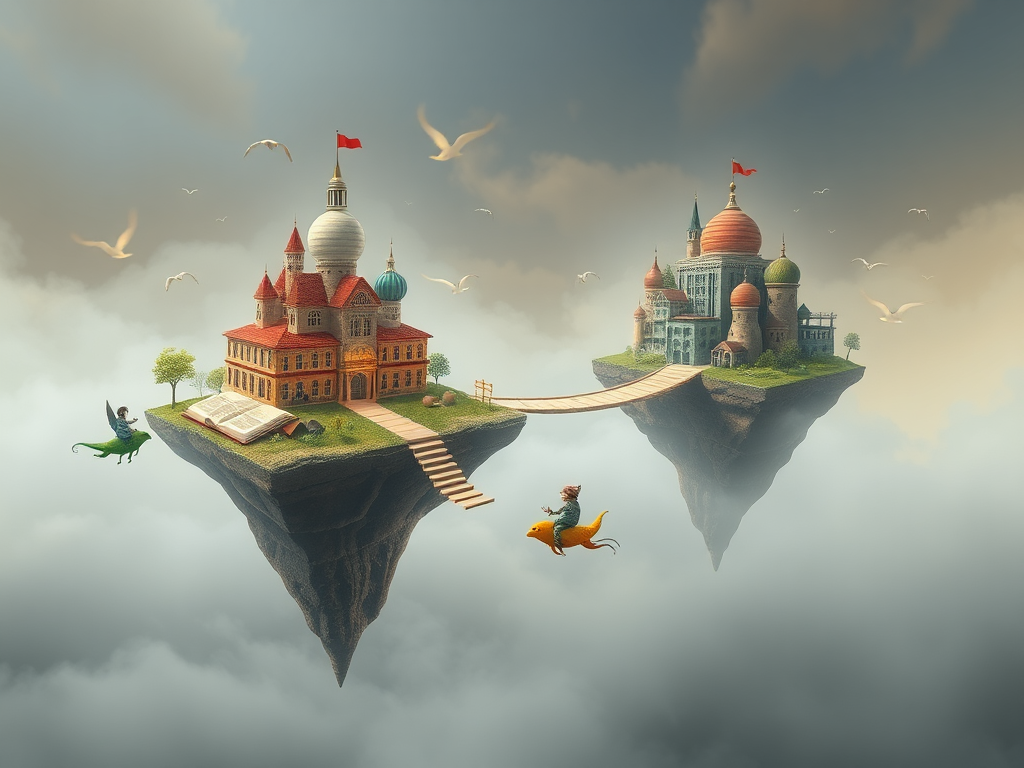
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
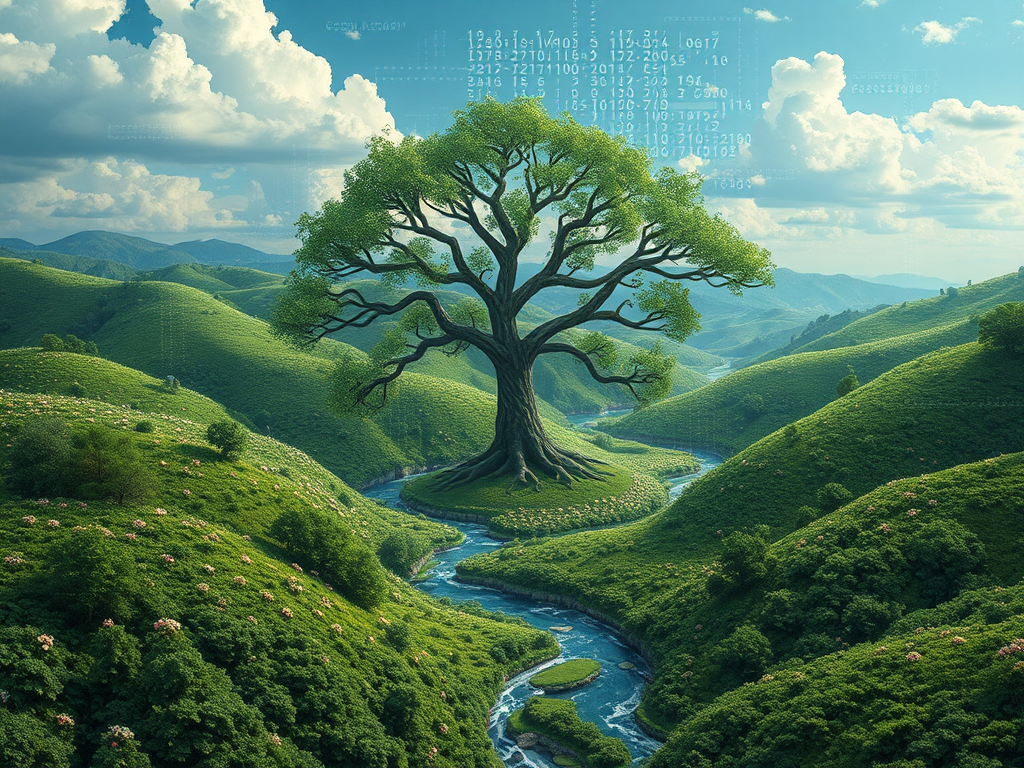
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
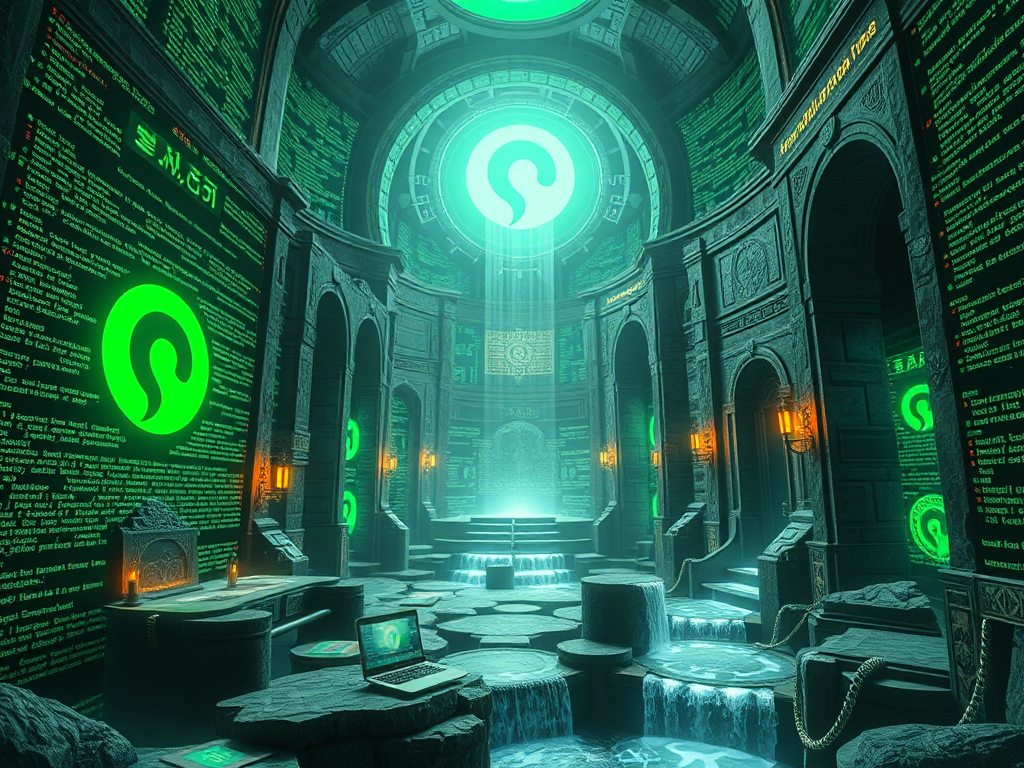
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit