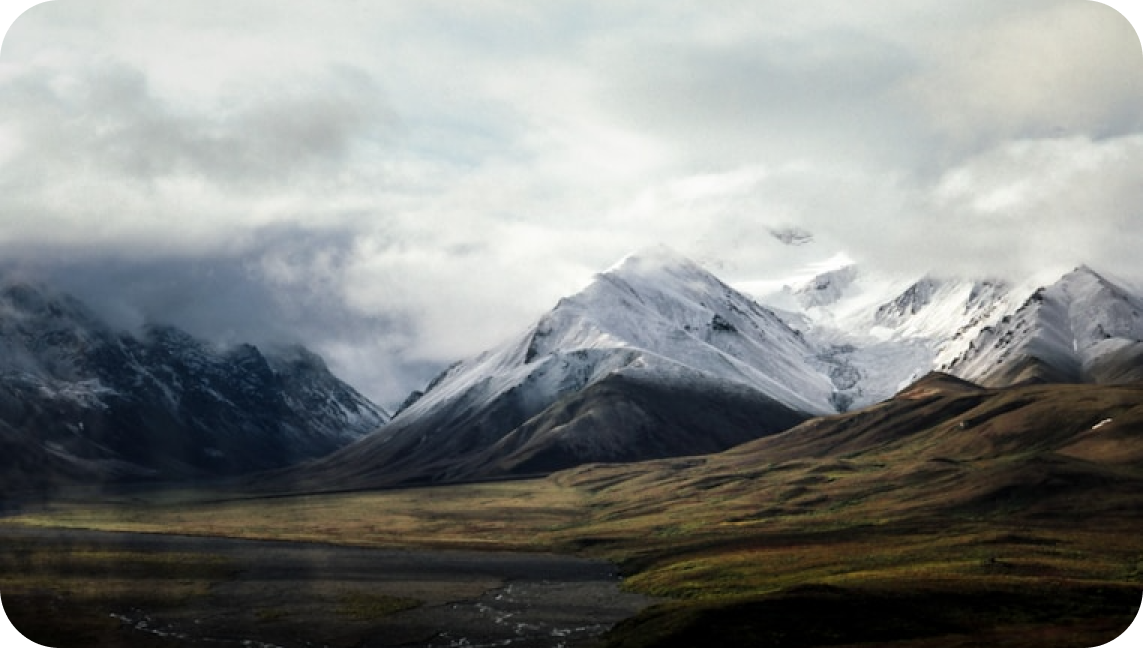
Are you often frustrated by large datasets? Is Python processing as slow as a snail crawling? Don't worry, today we're going to discuss how to make your Python data analysis code fly. I've stumbled into quite a few pitfalls and accumulated a lot of experience, so today I'll share my insights with you. Are you ready? Let's embark on this data analysis adventure!
Introduction
Remember the first time you dealt with a large dataset? Were you like me, full of anticipation when running the code, then faced with a long wait, and possibly even a crash due to insufficient memory? Haha, I bet you've been through that too. But don't be discouraged, these problems can all be solved. Today, I'm going to share with you some secrets to make Python data analysis more efficient.
The Big Guns
When it comes to handling large datasets, we can't help but mention some "big guns". Have you heard of the libraries dask and modin? They're powerful tools for handling big data!
dask is a flexible parallel computing library that allows you to easily handle datasets larger than your memory size. Imagine you have a 100GB CSV file to process, but your computer only has 16GB of memory. Regular pandas certainly won't cut it, but with dask, you can handle it with ease.
Let's see how dask works:
import dask.dataframe as dd
df = dd.read_csv('huge_file.csv')
result = df.groupby('column').mean().compute()
Isn't it simple? dask's API is very similar to pandas, so if you're familiar with pandas, using dask requires almost no additional learning.
modin is another powerful tool. Its goal is to speed up pandas operations, and the best part is, you hardly need to change your code! Just replace pandas with modin.pandas when importing:
import modin.pandas as pd
df = pd.read_csv('huge_file.csv')
result = df.groupby('column').mean()
See? The code is exactly the same as using pandas, but the speed could be many times faster!
I remember once I needed to process a 20GB log file. With regular pandas, my computer just froze. But after switching to modin, the whole process was unbelievably smooth. This experience really made me feel like I had gained superpowers!
Smart Tricks
Besides these "big guns", there are some small tricks that can greatly improve your data processing efficiency. For example, do you know about the categorical data type? It can help you save a lot of memory.
Imagine you have a dataset with millions of rows, and one of the columns is "country". If you store it as regular strings, it will certainly occupy a lot of memory. But if we convert it to categorical type, the memory usage will be greatly reduced:
import pandas as pd
df = pd.read_csv('large_file.csv')
df['country'] = df['country'].astype('category')
I once dealt with a large dataset containing global user data. After using the categorical type, memory usage decreased by nearly 50%! This not only gave my computer a breather but also sped up the processing significantly.
Also, have you heard of the chunking technique? When the data is really too big for even dask and modin to handle, we can consider processing the data in batches:
import pandas as pd
chunk_size = 100000
for chunk in pd.read_csv('enormous_file.csv', chunksize=chunk_size):
# Process each batch of data
process_chunk(chunk)
This method may seem a bit "clumsy", but it's very effective when dealing with extremely large datasets. I once successfully processed a 200GB dataset using this method, and the entire process didn't encounter any memory issues.
Efficiency King
After talking about techniques for handling big data, let's discuss how to improve efficiency in daily data analysis. Do you know about the groupby and agg methods? They're the kings of efficiency in data analysis!
Many people like to use loops when doing data aggregation. But actually, using groupby and agg can make your code more concise and faster:
import pandas as pd
df = pd.DataFrame({
'date': pd.date_range(start='2023-01-01', periods=1000),
'product': ['A', 'B', 'C'] * 333 + ['A'],
'sales': np.random.randint(1, 100, 1000)
})
result = df.groupby('product').agg({
'sales': ['sum', 'mean', 'max', 'min']
})
Look, with just a few lines of code, we've completed grouping by product and calculating the sum, average, maximum, and minimum of sales. If we were to implement this using loops, the amount of code might be several times more!
I remember once I needed to analyze a year's worth of sales data for an e-commerce platform. The data volume was huge, and various statistical indicators needed to be calculated. Initially, I used loops to implement it, and it ran for over half an hour without results. Later, when I switched to using groupby and agg methods, the entire process took less than a minute to complete! This improvement in efficiency really shocked me.
Also, when merging data, the merge and join methods are very powerful. But note that proper use of indexes can greatly increase the speed of merging:
import pandas as pd
df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'], 'value1': [1, 2, 3, 4]})
df2 = pd.DataFrame({'key': ['B', 'D', 'E', 'F'], 'value2': [5, 6, 7, 8]})
df1 = df1.set_index('key')
df2 = df2.set_index('key')
result = df1.join(df2, how='outer')
Using indexes for merging can significantly increase speed, especially when dealing with large datasets. I once needed to merge two datasets, each with several million rows. After using indexes, the merging time was reduced from half an hour to just a few minutes!
Cleaning Techniques
In data analysis, data cleaning might be the most time-consuming and annoying part. But with some techniques, this process can become much easier.
Handling missing values is a common problem in data cleaning. pandas provides two methods, fillna() and dropna(), to handle missing values:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': [1, 2, np.nan, 4],
'B': [5, np.nan, np.nan, 8],
'C': [9, 10, 11, 12]
})
df_filled = df.fillna(method='ffill') # Use forward fill
df_dropped = df.dropna()
Whether to fill or delete depends on your specific needs. I remember once when I was dealing with time series data, I encountered many missing values. Initially, I wanted to delete these missing values directly, but later found that this would lose a lot of important information. In the end, I chose to use forward fill, which preserved the continuity of the data without introducing too much bias.
Also, when dealing with outliers, we can use the describe() method to quickly understand the distribution of the data, and then handle it according to the specific situation:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'value': [1, 2, 3, 1000, 5, 6, 7, 8, 9, 10]
})
print(df.describe())
Q1 = df['value'].quantile(0.25)
Q3 = df['value'].quantile(0.75)
IQR = Q3 - Q1
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
df_cleaned = df[(df['value'] >= lower_bound) & (df['value'] <= upper_bound)]
This method can help us quickly identify and handle outliers. I once used this method when analyzing a set of sensor data. There were some obviously unreasonable extreme values in the data. After using the IQR method, I successfully eliminated these outliers, making the subsequent analysis results more reliable.
The Final Touch
The last step in data analysis is usually data visualization. Good visualization can make your analysis results more intuitive and convincing. In Python, matplotlib and seaborn are two very powerful visualization libraries.
Let's first look at how to create a simple line chart using matplotlib:
import pandas as pd
import matplotlib.pyplot as plt
df = pd.DataFrame({
'date': pd.date_range(start='2023-01-01', periods=100),
'value': np.random.randn(100).cumsum()
})
plt.figure(figsize=(12, 6))
plt.plot(df['date'], df['value'])
plt.title('Time Series Plot')
plt.xlabel('Date')
plt.ylabel('Value')
plt.grid(True)
plt.show()
This code will create a simple time series line chart. However, if you want to create more complex and beautiful charts, seaborn might be a better choice:
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
df = pd.DataFrame({
'x': np.random.randn(100),
'y': np.random.randn(100),
'category': np.random.choice(['A', 'B', 'C'], 100)
})
plt.figure(figsize=(12, 8))
sns.scatterplot(data=df, x='x', y='y', hue='category', style='category')
plt.title('Scatter Plot with Multiple Categories')
plt.show()
This code will create a scatter plot with multiple categories, each category represented by a different color and shape.
I remember once I needed to report a complex data analysis result to the company's senior management. Initially, I just presented the data in tables, but found that many people couldn't understand it. Later, I created a series of beautiful visualization charts using seaborn, which not only made the data more intuitive but also successfully attracted everyone's attention. Since then, I've placed more emphasis on data visualization.
Conclusion
Well, that's the end of our Python data analysis journey. We've discussed how to handle large datasets, how to improve code efficiency, how to perform data cleaning, and how to create beautiful visualization charts. These techniques and methods are all things I've figured out in practice, and I hope they'll be helpful to you.
Remember, data analysis is an art that requires continuous learning and practice. Every new challenge is an opportunity to learn. Don't be afraid to try new methods and tools, because you never know which one will be the key to solving a problem.
Finally, I want to say, enjoy the process of data analysis! When you see your code quickly processing large amounts of data, or creating a beautiful visualization chart, that sense of achievement is unparalleled.
So, do you have any data analysis experiences you'd like to share? Or have you encountered any interesting problems in practice? Feel free to discuss with me in the comments! Let's explore together in this ocean of data and discover more treasures!
Next
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts
Next
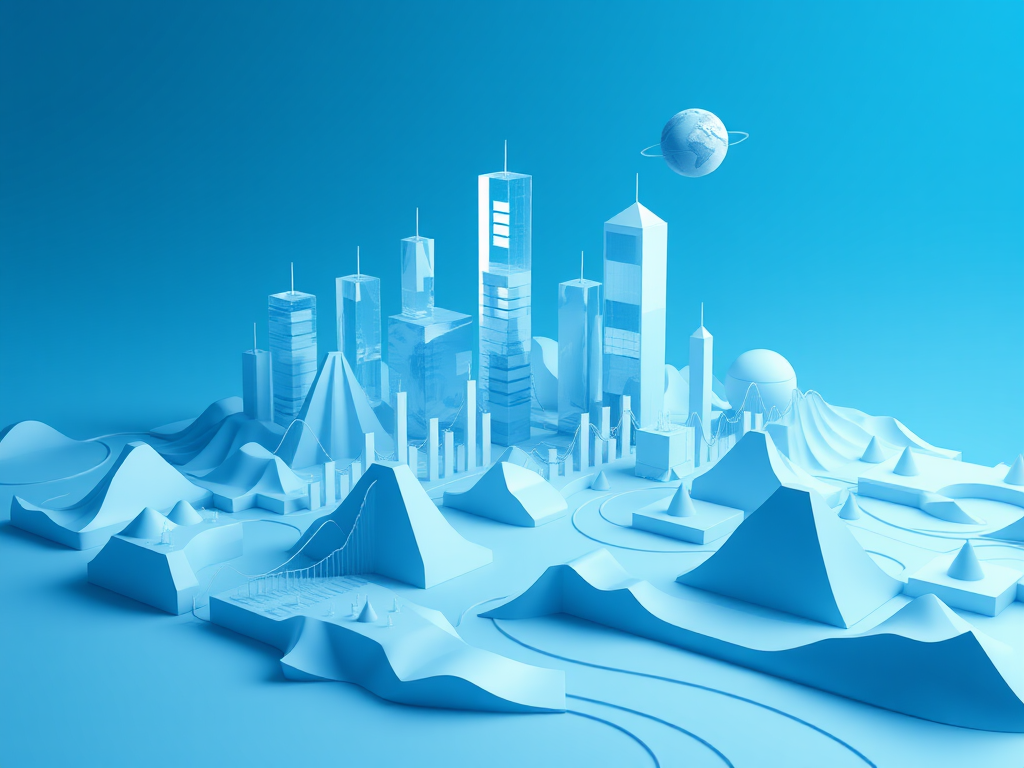
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
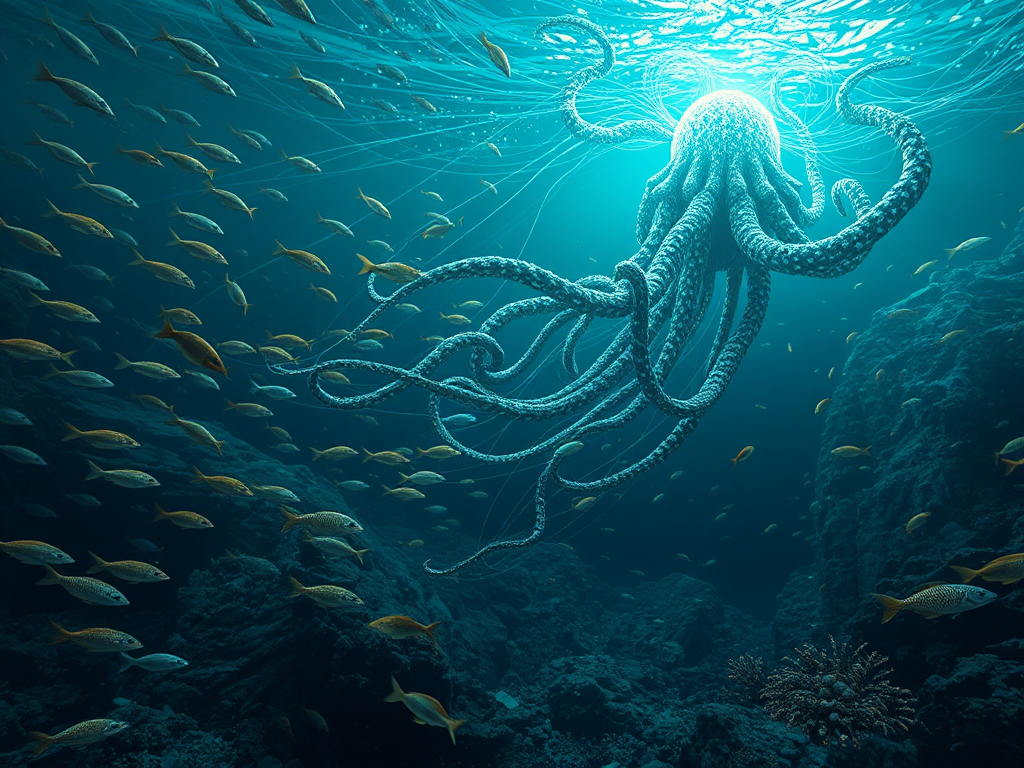
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
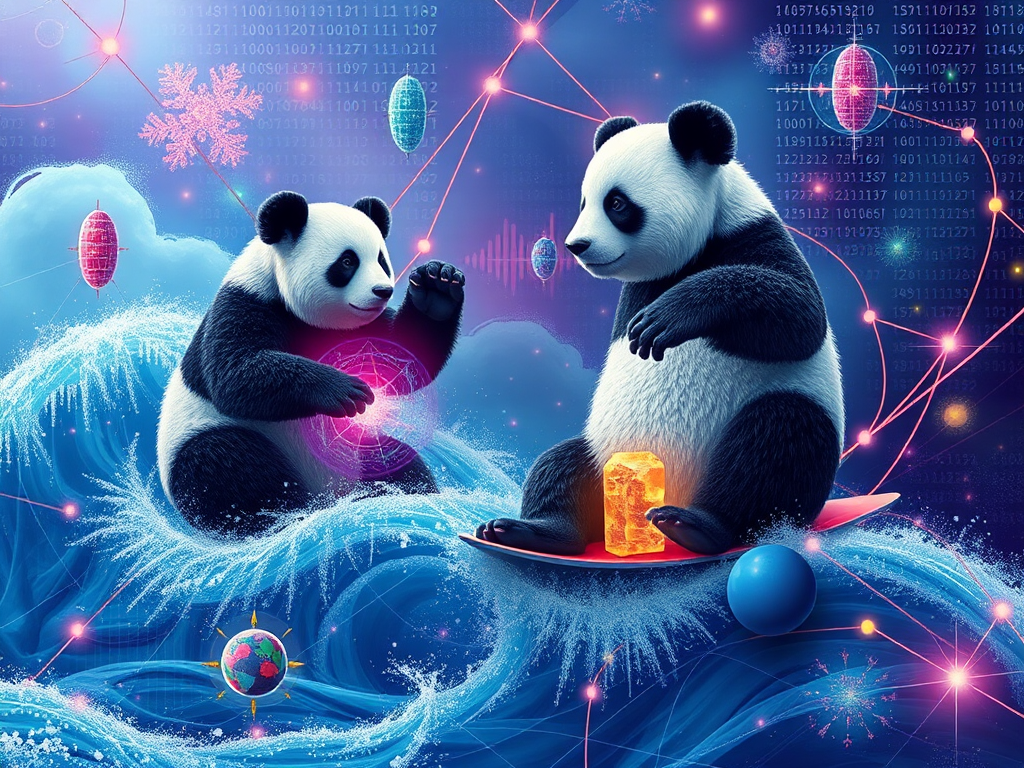
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts