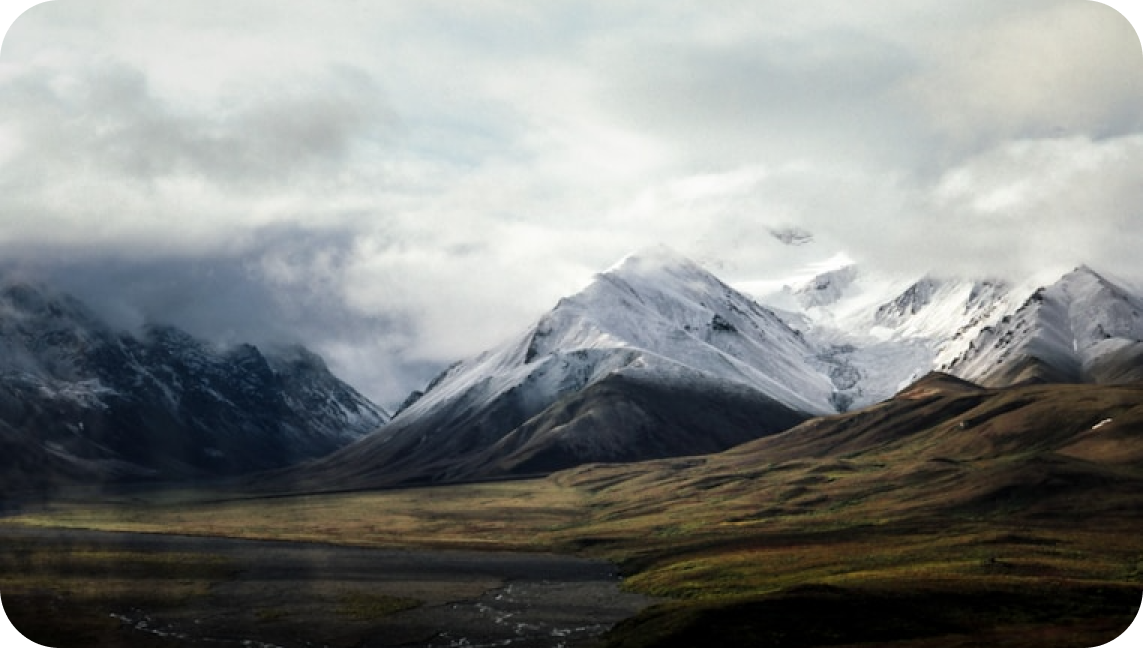
Hi, friends! Today, let's talk about some common techniques and optimization strategies in Python data analysis. Data analysis is not a simple task, especially when dealing with large datasets, where code efficiency and readability become particularly important. So, what are some tips that can help us achieve better results with less effort? Let's take a look together!
Improving Efficiency
Optimizing Code
I believe you, like me, have encountered situations in data analysis projects where the code is lengthy, repetitive, and inefficient. But don't worry, there are some clever approaches that can help us optimize our code, making it more concise and efficient.
For example, you can use the groupby
and agg
functions to combine multiple operations, achieving multiple goals at once. Take a look at this example:
app_category_info = apps.groupby('Category').agg(
Number_of_apps=('Category', 'count'),
Average_price=('Price', 'mean'),
Average_rating=('Rating', 'mean')
).reset_index()
Doesn't it look concise? This line of code can help you calculate the number of apps, average price, and average rating for each app category in one go! It not only reduces the number of code lines but also improves execution efficiency and readability. Isn't that cool?
Big Data Optimization
Well, when it comes to processing large datasets, this can be a big problem. I often encounter performance bottlenecks where code runs extremely inefficiently. However, we have some tricks to solve this problem:
-
Using dask: This is a powerful tool specifically for handling big data. It can perform parallel computations and process datasets larger than memory size. With it in hand, big data is no longer a problem.
-
Optimizing data types: We need to ensure we're using appropriate data types, such as converting
float64
tofloat32
, which can significantly reduce memory usage and improve running efficiency. -
Reading data in chunks: Imagine trying to read an extremely large dataset all at once - that would be a heavy burden. However, using the
chunksize
parameter ofpd.read_csv()
, you can read the data in small chunks and process it piece by piece. This greatly reduces system pressure and improves running speed.
You see, once you master these little tricks, even the largest datasets can't block our path to analysis!
Data Cleaning
Conditional Filtering
In data analysis, we often need to filter out part of the data that meets specific conditions from a large amount of data. This is where boolean indexing comes in handy. Take a look at this example:
filtered_data = df[(df['column_name'] > threshold) & (df['another_column'] == some_value)]
This code can help you extract numbers from the dataframe that meet multiple conditions. Isn't that convenient? You can also use the query()
method to improve code readability, killing two birds with one stone!
Handling Outliers
In real-world data, we always encounter some outliers or missing values, such as data erroneously entered as zero. Don't worry, Python data analysis libraries provide us with multiple ways to handle this.
You can use the replace()
method to replace zero values with NaN
:
df.replace(0, np.nan, inplace=True)
You can also achieve the same effect using the mask()
method. By applying these techniques, you can easily clean your dataset and prepare it well for analysis.
Data Sorting
Multi-column Sorting
When analyzing data, we often need to sort the data based on the values of multiple columns. This is where the sort_values()
method becomes our reliable assistant. Take a look at this example:
sorted_df = df.sort_values(by=['question_type', 'another_column'])
This line of code can help you sort the data based on the values of two columns. Moreover, you can easily adjust the sorting priority by simply changing the order of the column names. Isn't that super convenient?
Overall, Python data analysis libraries provide us with many practical techniques and optimization strategies. By mastering these, you'll be able to handle various data analysis tasks more efficiently. However, this is just the beginning, there's more excitement waiting for us to explore in the future! So, let's keep working hard and continue our journey on the path of data analysis!
Next
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts
Next
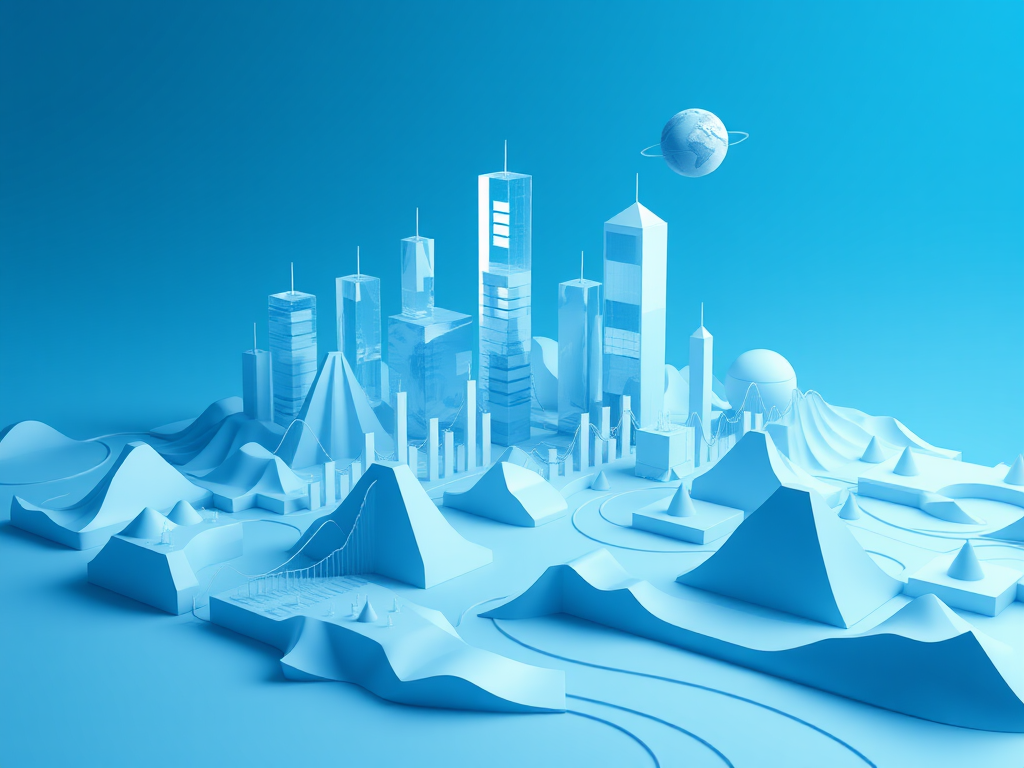
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
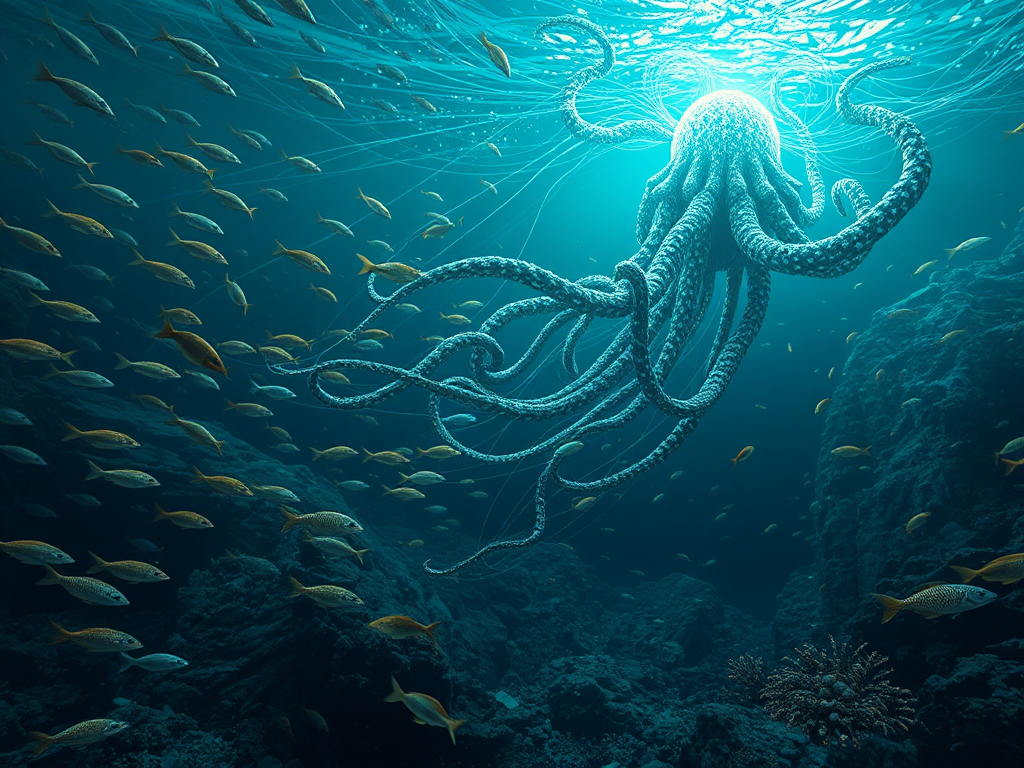
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
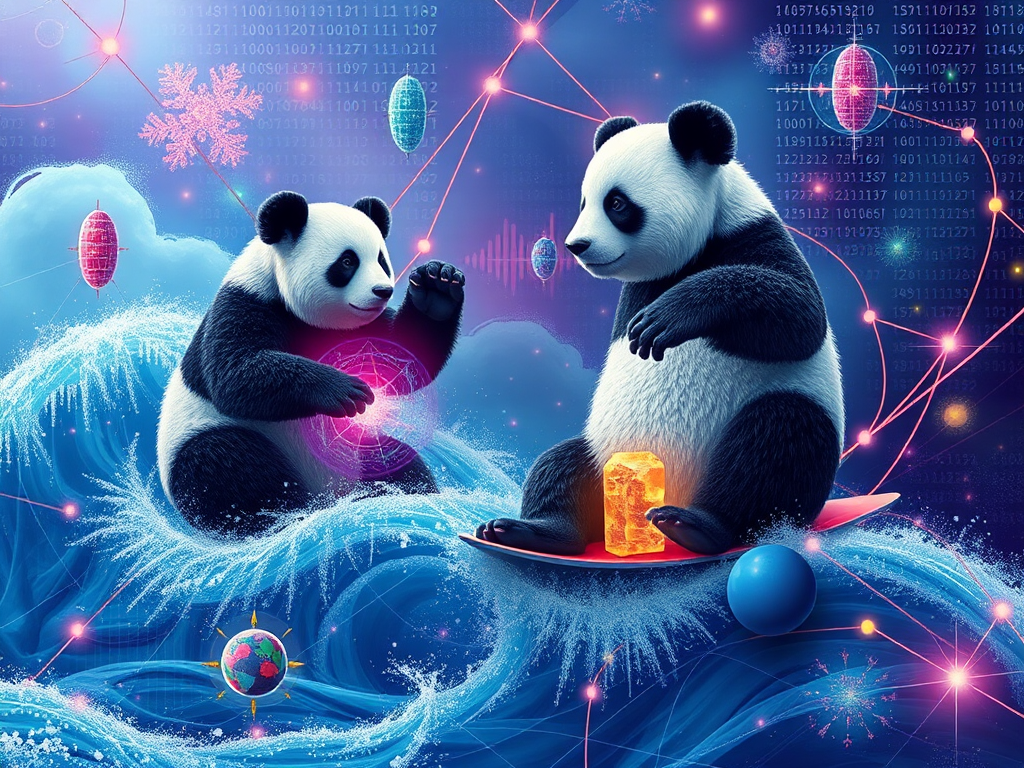
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts