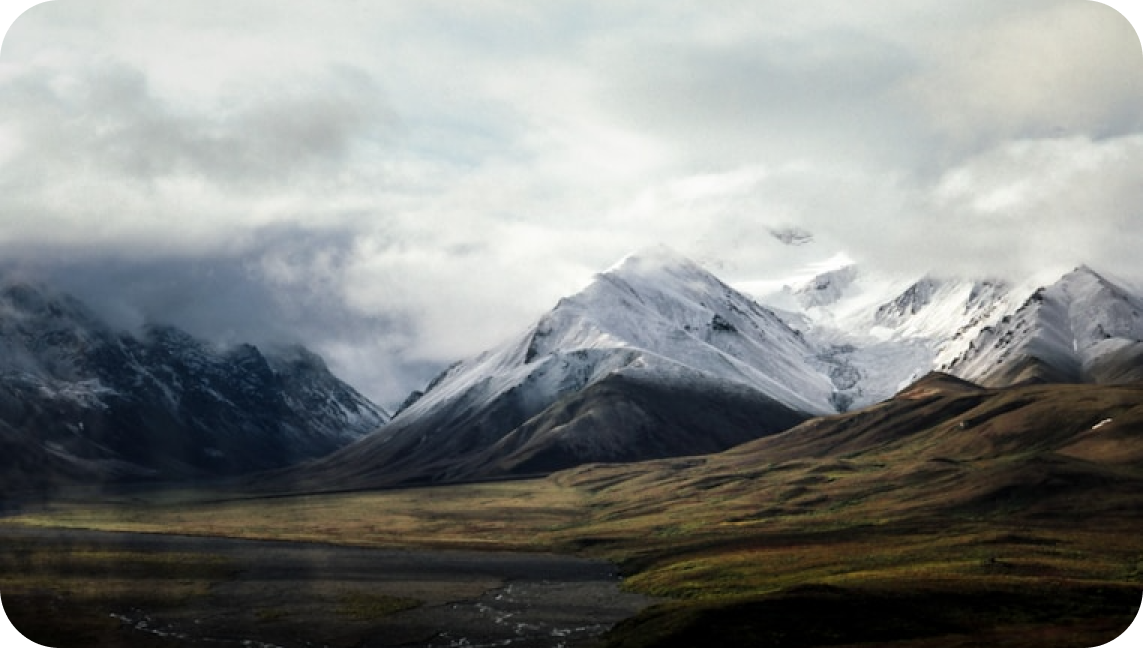
Have you ever felt overwhelmed when dealing with large amounts of data? Or been unsure where to start when faced with complex data analysis tasks? Don't worry, today we'll discuss some practical tips for Python data analysis to make your code more efficient and elegant.
Data Processing
In the data analysis process, data processing is undoubtedly one of the most important steps. How to efficiently process large datasets? How to elegantly handle missing values? These are issues we need to face.
Efficient Processing
When we deal with large datasets, the most common problem is running out of memory. You might think, do I need to upgrade my computer? Actually, no, Python provides us with some clever solutions.
First, we can use the chunksize
parameter of the Pandas read_csv()
function to read data in chunks. This trick is especially useful when your dataset is too large to load into memory all at once. Let's look at an example:
import pandas as pd
chunk_size = 10000
for chunk in pd.read_csv('large_file.csv', chunksize=chunk_size):
# Process each chunk
process_data(chunk)
In this example, we split the large CSV file into multiple small chunks, each containing 10000 rows of data. This way, we can process the data chunk by chunk, without loading everything into memory at once.
Additionally, if you need to process extremely large datasets, you can consider using the Dask library. Dask can help you process data in parallel, greatly improving efficiency. Look at this example:
import dask.dataframe as dd
df = dd.read_csv('large_file.csv')
result = df.groupby('column_name').mean().compute()
In this example, Dask will automatically split the data into multiple partitions, process them in parallel, and then combine the results. This works even for datasets in the terabyte range.
Finally, I want to emphasize one point: in Python, try to use vectorized operations instead of loops whenever possible. Why? Because vectorized operations can significantly improve efficiency. Look at this comparison:
import numpy as np
def slow_sum(arr):
total = 0
for x in arr:
total += x
return total
def fast_sum(arr):
return np.sum(arr)
arr = np.random.rand(1000000)
%timeit slow_sum(arr)
%timeit fast_sum(arr)
You'll find that the vectorized fast_sum
function is several orders of magnitude faster than the loop-based slow_sum
function. That's the magic of vectorized operations.
Elegant Processing
In real-world data analysis, we often encounter missing values. How we handle these missing values can often affect our analysis results. Pandas provides us with some elegant solutions.
First, we can use the fillna()
method to fill missing values:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, None, 4], 'B': [5, None, 7, 8]})
df_filled = df.fillna(0)
df_ffilled = df.fillna(method='ffill')
df_bfilled = df.fillna(method='bfill')
print("Original data:
", df)
print("
Filled with 0:
", df_filled)
print("
Forward-filled:
", df_ffilled)
print("
Backward-filled:
", df_bfilled)
In this example, we demonstrate three ways to fill missing values: filling with a specific value, forward-filling, and backward-filling. Each method is suitable for different scenarios, and you need to choose the appropriate one based on the characteristics of your data.
Sometimes, we might want to directly drop rows or columns containing missing values. In that case, we can use the dropna()
method:
df_dropped_rows = df.dropna()
df_dropped_cols = df.dropna(axis=1, how='all')
print("Dropped rows with missing values:
", df_dropped_rows)
print("
Dropped columns with all missing values:
", df_dropped_cols)
When dealing with missing values, we need to be cautious. Blindly dropping or filling missing values can lead to data bias. Therefore, before handling missing values, it's best to understand the reasons for their occurrence and their distribution in the data.
You can use the following code to examine the distribution of missing values:
missing_count = df.isnull().sum()
missing_percentage = 100 * df.isnull().sum() / len(df)
missing_table = pd.concat([missing_count, missing_percentage], axis=1, keys=['Missing Count', 'Missing Percentage'])
print(missing_table)
This code will give you a clear view of the number and percentage of missing values in each column. This can help you make more informed decisions, such as whether to drop columns with too many missing values.
Data Merging
In real-world data analysis, we often need to work with multiple data sources. How to effectively merge these data sources is a question worth exploring.
Using pd.concat()
The pd.concat()
function is a powerful tool that can help us concatenate multiple objects along a particular axis. Let's look at an example:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'C': ['C0', 'C1', 'C2', 'C3']},
index=[0, 1, 2, 3])
df2 = pd.DataFrame({'A': ['A4', 'A5', 'A6', 'A7'],
'B': ['B4', 'B5', 'B6', 'B7'],
'C': ['C4', 'C5', 'C6', 'C7']},
index=[4, 5, 6, 7])
result = pd.concat([df1, df2])
print(result)
In this example, we create two DataFrames and then use pd.concat()
to concatenate them along the row axis (axis=0). This operation is equivalent to adding df2 to the bottom of df1.
The pd.concat()
function also has some useful parameters, such as ignore_index
and keys
. Let's look at their effects:
result_ignore_index = pd.concat([df1, df2], ignore_index=True)
result_keys = pd.concat([df1, df2], keys=['x', 'y'])
print("Result with ignored index:
", result_ignore_index)
print("
Result with hierarchical index:
", result_keys)
ignore_index=True
will reset the index to start from 0, while the keys
parameter will add a hierarchical index, which is particularly useful when dealing with data from different sources.
Using pd.merge()
Although pd.concat()
is useful for simple data merging scenarios, in more complex situations, we might need to use the pd.merge()
function. pd.merge()
allows us to merge DataFrames based on one or more keys.
Let's look at an example:
import pandas as pd
df1 = pd.DataFrame({'employee': ['John', 'Anna', 'Peter', 'Linda'],
'group': ['Accounting', 'Engineering', 'Engineering', 'HR']})
df2 = pd.DataFrame({'employee': ['John', 'Anna', 'Peter', 'Linda'],
'hire_date': ['2015-01-01', '2016-03-23', '2017-05-25', '2018-07-30']})
result = pd.merge(df1, df2, on='employee')
print(result)
In this example, we merge the two DataFrames based on the 'employee' column. By default, pd.merge()
performs an inner join, which means that only employees present in both DataFrames will appear in the result.
The pd.merge()
function also supports other types of joins, such as left join, right join, and outer join:
left_join = pd.merge(df1, df2, on='employee', how='left')
right_join = pd.merge(df1, df2, on='employee', how='right')
outer_join = pd.merge(df1, df2, on='employee', how='outer')
print("Left join result:
", left_join)
print("
Right join result:
", right_join)
print("
Outer join result:
", outer_join)
These different types of joins allow us to handle various data merging scenarios flexibly. For example, if you want to keep all records from df1, regardless of whether they exist in df2, you can use a left join.
In real applications, we might need to merge data based on multiple columns. pd.merge()
also supports this:
df3 = pd.DataFrame({'employee': ['John', 'Anna', 'Peter', 'Linda'],
'group': ['Accounting', 'Engineering', 'Engineering', 'HR'],
'city': ['New York', 'Boston', 'New York', 'Boston']})
df4 = pd.DataFrame({'employee': ['John', 'Anna', 'Peter', 'Linda'],
'hire_date': ['2015-01-01', '2016-03-23', '2017-05-25', '2018-07-30'],
'city': ['New York', 'Boston', 'New York', 'Boston']})
result_multi = pd.merge(df3, df4, on=['employee', 'city'])
print(result_multi)
In this example, we merge the data based on both the 'employee' and 'city' columns. This ensures that we only merge records where both the employee name and city match.
Data Visualization
Data visualization is a crucial part of data analysis. A good chart can often convey information more intuitively than thousands of rows of data. In Python, we mainly use the Matplotlib and Seaborn libraries for data visualization.
Using Matplotlib
Matplotlib is the most fundamental and commonly used plotting library in Python. It provides a MATLAB-like plotting API and can create various static, dynamic, and interactive plots.
Let's start with a simple line plot:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.figure(figsize=(10, 6))
plt.plot(x, y, label='sin(x)')
plt.title('Sine Wave')
plt.xlabel('x')
plt.ylabel('y')
plt.legend()
plt.grid(True)
plt.show()
This code will generate a sine wave plot. We use plt.figure()
to create a new figure, and then use plt.plot()
to draw the line plot. plt.title()
, plt.xlabel()
, and plt.ylabel()
are used to set the title and axis labels, respectively. plt.legend()
adds a legend, and plt.grid()
adds grid lines.
Matplotlib also supports subplots, which are very useful when we need to compare multiple datasets in a single figure:
fig, axs = plt.subplots(2, 2, figsize=(12, 10))
axs[0, 0].plot(x, np.sin(x))
axs[0, 0].set_title('sin(x)')
axs[0, 1].plot(x, np.cos(x))
axs[0, 1].set_title('cos(x)')
axs[1, 0].plot(x, np.tan(x))
axs[1, 0].set_title('tan(x)')
axs[1, 1].plot(x, np.exp(x))
axs[1, 1].set_title('exp(x)')
plt.tight_layout()
plt.show()
This code creates a 2x2 grid of subplots and plots different functions in each subplot. plt.tight_layout()
is used to automatically adjust the spacing between subplots to avoid overlapping.
Using Seaborn
While Matplotlib is powerful, sometimes we might need more visually appealing and statistically-oriented plots. This is where Seaborn comes into play. Seaborn is a statistical data visualization library built on top of Matplotlib, providing a higher-level interface for creating attractive statistical graphics.
Let's look at an example of using Seaborn to create a violin plot:
import seaborn as sns
import pandas as pd
import numpy as np
np.random.seed(0)
data = pd.DataFrame({
'group': np.repeat(['A', 'B', 'C', 'D'], 250),
'value': np.random.randn(1000)
})
sns.set_style("whitegrid")
plt.figure(figsize=(10, 6))
sns.violinplot(x='group', y='value', data=data)
plt.title('Violin Plot')
plt.show()
This code first creates a DataFrame containing 1000 data points, and then uses Seaborn's violinplot()
function to create a violin plot. The violin plot combines a box plot and a kernel density estimation, providing a more comprehensive view of the data distribution.
Seaborn also provides many other types of statistical plots, such as heatmaps:
corr_matrix = np.random.rand(5, 5)
corr_matrix = (corr_matrix + corr_matrix.T) / 2
np.fill_diagonal(corr_matrix, 1)
plt.figure(figsize=(10, 8))
sns.heatmap(corr_matrix, annot=True, cmap='coolwarm', vmin=-1, vmax=1, center=0)
plt.title('Correlation Heatmap')
plt.show()
This code creates a random correlation matrix and then uses Seaborn's heatmap()
function to create a heatmap. Heatmaps are particularly suitable for visualizing matrix data, especially when showing correlations between variables.
Pivot Tables
Pivot tables are a powerful tool in data analysis, helping us quickly summarize and compare large amounts of data. In Python, we can use the Pandas pivot_table()
function to create pivot tables.
Creating Pivot Tables
Let's look at an example to see how to create and use pivot tables:
import pandas as pd
import numpy as np
np.random.seed(0)
data = pd.DataFrame({
'date': pd.date_range(start='2023-01-01', periods=1000),
'product': np.random.choice(['A', 'B', 'C', 'D'], 1000),
'region': np.random.choice(['North', 'South', 'East', 'West'], 1000),
'sales': np.random.randint(100, 1000, 1000)
})
pivot_table = pd.pivot_table(data,
values='sales',
index=['region'],
columns=['product'],
aggfunc=np.sum,
fill_value=0)
print(pivot_table)
In this example, we first create a DataFrame containing dates, products, regions, and sales amounts. Then, we use the pivot_table()
function to create a pivot table, where:
- The
values
parameter specifies the column to aggregate - The
index
parameter specifies the row index - The
columns
parameter specifies the column index - The
aggfunc
parameter specifies the aggregation function - The
fill_value
parameter specifies how to fill missing values
The result is a table summarizing sales amounts by region and product.
Summarizing and Comparing Data
Pivot tables can be used not only for data summarization but also for data comparison. Let's look at a more complex example:
data['year'] = data['date'].dt.year
data['month'] = data['date'].dt.month
multi_index_pivot = pd.pivot_table(data,
values='sales',
index=['region', 'year'],
columns=['product', 'month'],
aggfunc=np.sum,
fill_value=0)
print(multi_index_pivot)
In this example, we create a multi-index pivot table. This table allows us to simultaneously compare sales across different regions, years, products, and months.
Pivot tables also support marginal totals. We can use the margins
parameter to add row and column totals:
pivot_with_margins = pd.pivot_table(data,
values='sales',
index=['region'],
columns=['product'],
aggfunc=np.sum,
fill_value=0,
margins=True,
margins_name='Total')
print(pivot_with_margins)
This pivot table not only shows the sales amount for each region and product but also displays the total sales for each region and the total sales for each product.
Pivot tables are a highly flexible tool, and you can adjust the index, columns, and aggregation functions according to your needs. For example, if you want to see the average sales amount and the number of sales for each region and product, you can do:
pivot_multi_agg = pd.pivot_table(data,
values='sales',
index=['region'],
columns=['product'],
aggfunc=[np.mean, len],
fill_value=0)
print(pivot_multi_agg)
This pivot table will show the average sales amount and the number of sales for each region and product.
Conclusion
Python data analysis is a vast field, and what we've discussed today is just the tip of the iceberg. However, by mastering these fundamental skills, you'll be able to handle most common data analysis tasks.
Remember, data analysis is not just about techniques; it's also an art. It requires you to continuously practice, develop a sensitivity to data, learn to ask the right questions, and find appropriate methods to answer them.
In your work, you may encounter various data analysis challenges. Don't be afraid of these challenges; they are the best opportunities for you to improve your skills. Stay curious, keep learning new techniques and methods, and you'll undoubtedly become an excellent data analyst.
So, are you ready to embark on your Python data analysis journey? Pick up your keyboard, open your IDE, and let's explore the ocean of data together.
Remember, in the world of data analysis, the most important thing is not what you already know but how eager you are to learn and explore. Maintain this eagerness, and you'll go further in this field.
I wish you smooth sailing on your Python data analysis journey. If you have any questions or thoughts, feel free to share them with me. Let's grow together in this challenging and rewarding field.
Next
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts
Next
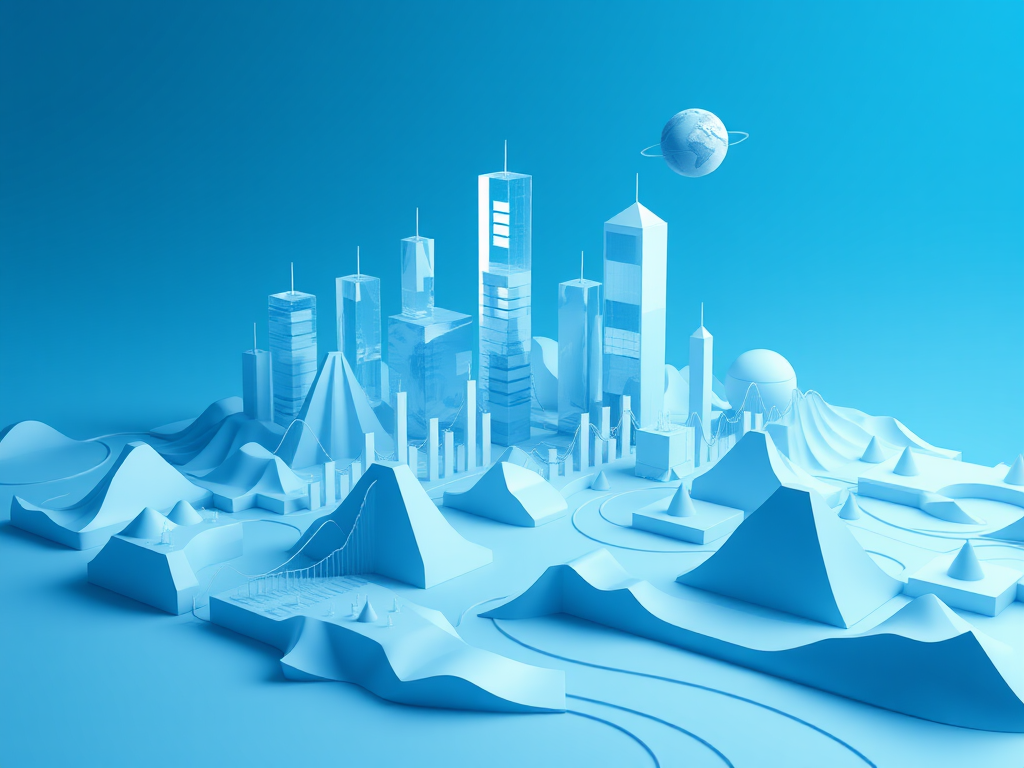
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
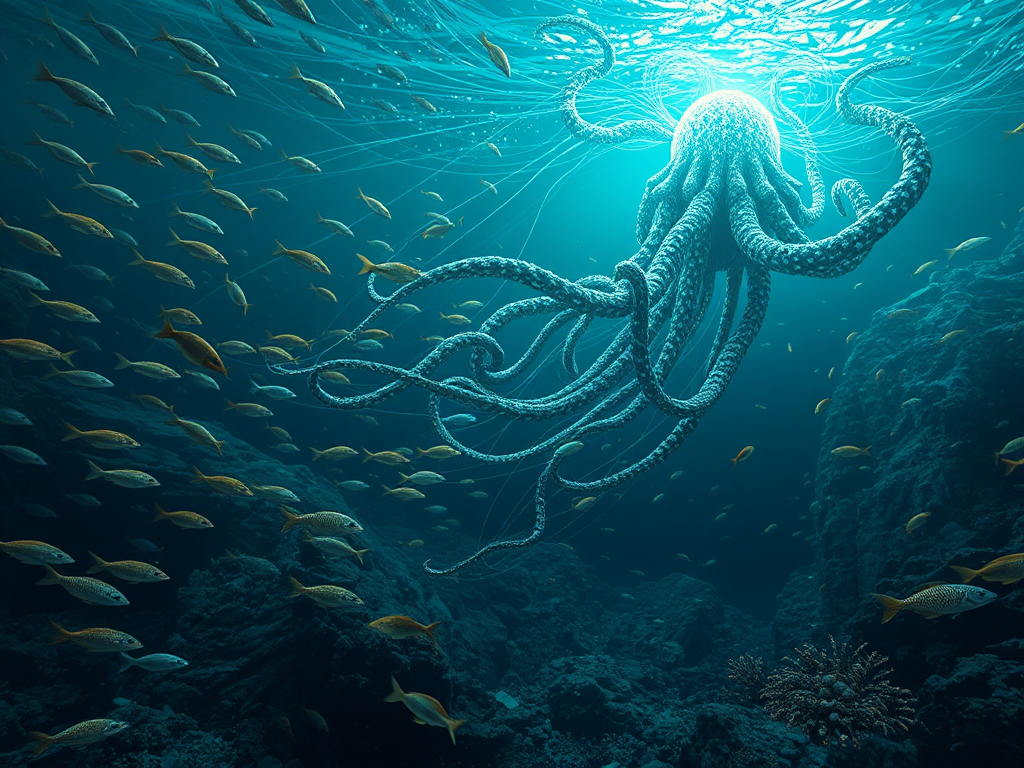
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
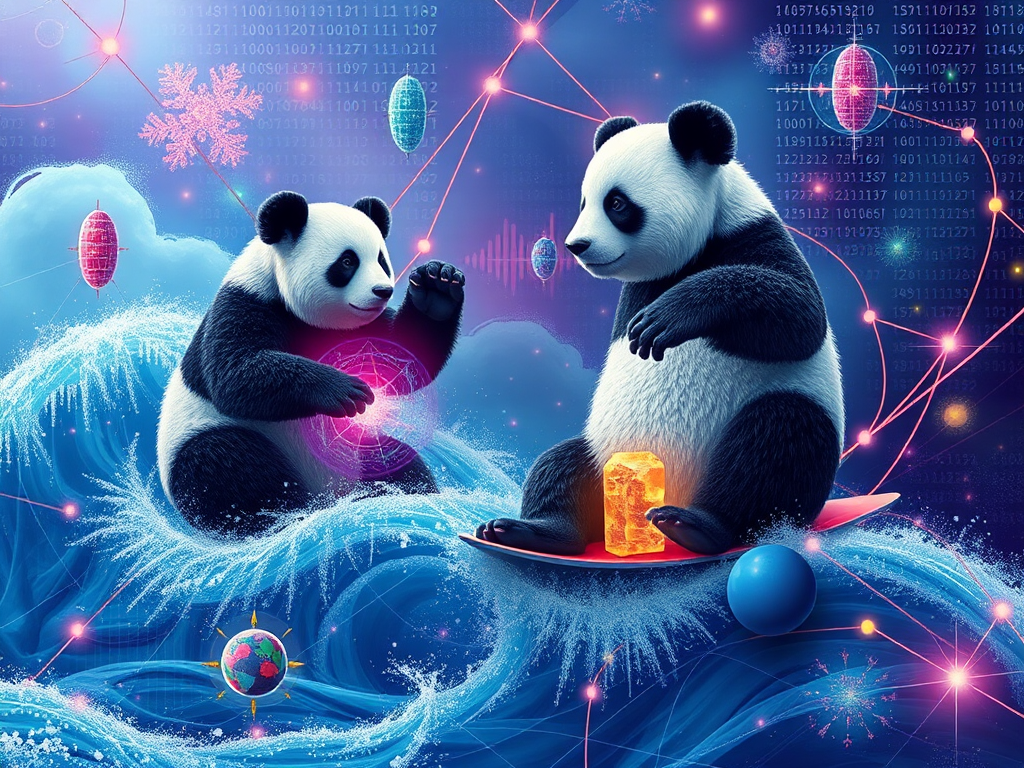
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts