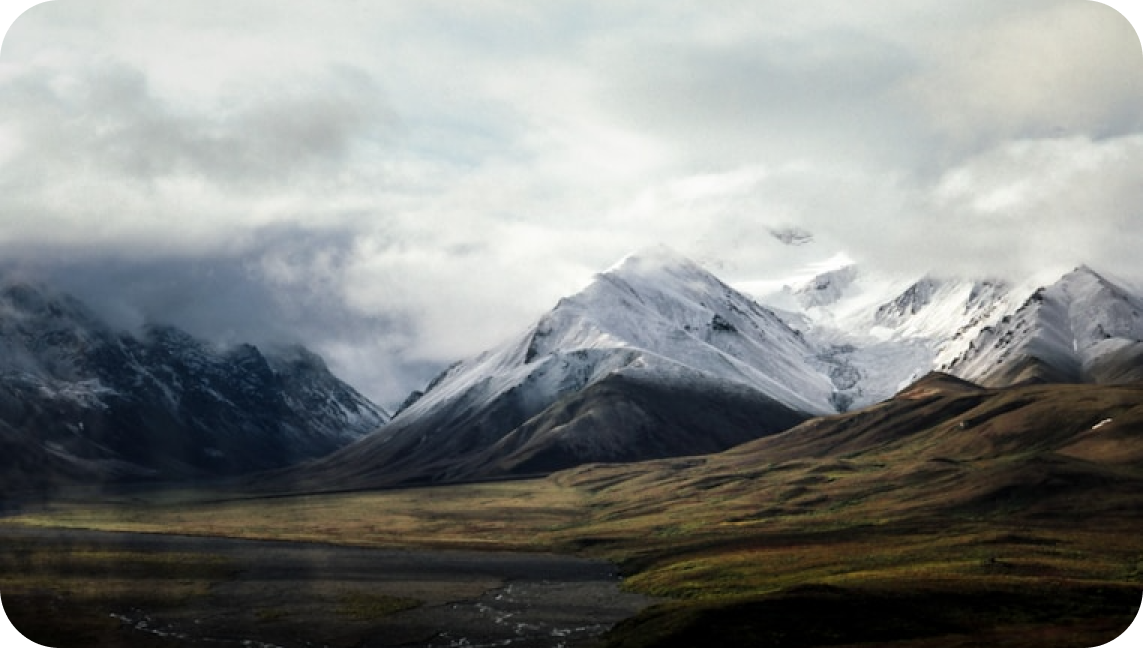
Background
Do you often encounter this problem: every time you handle large datasets, your computer starts making distressing noises? Or perhaps you've written a seemingly simple data processing code, but it takes several minutes to see the results? As a Python data analysis enthusiast, I understand deeply. Today, let me share some practical tips to help you solve these troubles.
Foundation
Before diving into the main topic, we need to understand some key concepts. Do you know why processing large-scale data with regular Python lists is so slow? It all comes down to memory management.
Python lists are dynamic arrays, stored non-contiguously in memory. NumPy arrays, however, are stored contiguously, which is why NumPy operations can be hundreds of times faster than native Python.
import numpy as np
import pandas as pd
normal_list = list(range(1000000))
numpy_array = np.arange(1000000)
Performance Optimization
Speaking of performance optimization, I have a very practical tip to share with you. When handling large DataFrames, have you noticed the importance of dtype?
df = pd.DataFrame({
'id': np.arange(1000000),
'value': np.random.randn(1000000),
'category': ['A'] * 1000000
})
df['id'] = df['id'].astype('int32') # Reduced from default int64 to int32
df['category'] = df['category'].astype('category') # Use category type for repeated strings
This code can help you save nearly 50% of memory usage. I was shocked when I first discovered this trick. Imagine, a dataset that originally needed 1GB of memory now only requires 500MB—this is a lifesaver for handling large-scale data.
Data Cleaning
Data cleaning may be the most time-consuming step, but it also showcases skill. Let me share an efficient way to handle missing values:
def clean_data(df):
# Fill missing values in numerical columns with the median
numeric_columns = df.select_dtypes(include=['float64', 'int64']).columns
for col in numeric_columns:
df[col].fillna(df[col].median(), inplace=True)
# Fill missing values in categorical columns with the mode
categorical_columns = df.select_dtypes(include=['object', 'category']).columns
for col in categorical_columns:
df[col].fillna(df[col].mode()[0], inplace=True)
return df
Efficient Calculation
When it comes to computational efficiency, I must mention vectorized operations. Did you know? Avoiding for loops and using vectorized operations can speed up your code by more than ten times.
def slow_calculation(df):
result = []
for i in range(len(df)):
result.append(df['value'][i] * 2 + df['id'][i])
return result
def fast_calculation(df):
return df['value'] * 2 + df['id']
Visualization
Data visualization is the magic that makes boring data lively. I particularly like using the Seaborn library, which not only draws beautiful charts but also automatically handles statistical relationships.
import seaborn as sns
import matplotlib.pyplot as plt
def plot_distribution(df, column):
plt.figure(figsize=(10, 6))
sns.histplot(data=df, x=column, kde=True)
plt.title(f'Distribution of {column}')
plt.show()
Practical Case
Let's look at a practical case. Suppose we need to analyze a dataset containing one million user shopping data entries:
df = pd.DataFrame({
'user_id': np.random.randint(1, 10000, size=1000000),
'purchase_amount': np.random.normal(100, 20, size=1000000),
'category': np.random.choice(['A', 'B', 'C'], size=1000000)
})
result = df.groupby('user_id').agg({
'purchase_amount': ['mean', 'sum', 'count'],
'category': lambda x: x.value_counts().index[0] # Most frequently purchased category
}).reset_index()
Conclusion and Outlook
After sharing this article, do you have a new understanding of Python data analysis? We discussed the complete process from data cleaning to visualization, emphasizing key points of performance optimization.
Data analysis is a discipline that requires both technical skills and business understanding. In your actual work, besides the tips mentioned in this article, what other points are worth noting? Feel free to share your experiences and thoughts in the comments.
Remember, excellent data analysis is not only about writing efficient code but also about discovering valuable insights from the data. Let's continue to improve on this path.
Which techniques mentioned in the article are you particularly interested in? Or have you encountered any tricky data processing problems in your actual work? Feel free to leave a comment for discussion.
Next
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts
Next
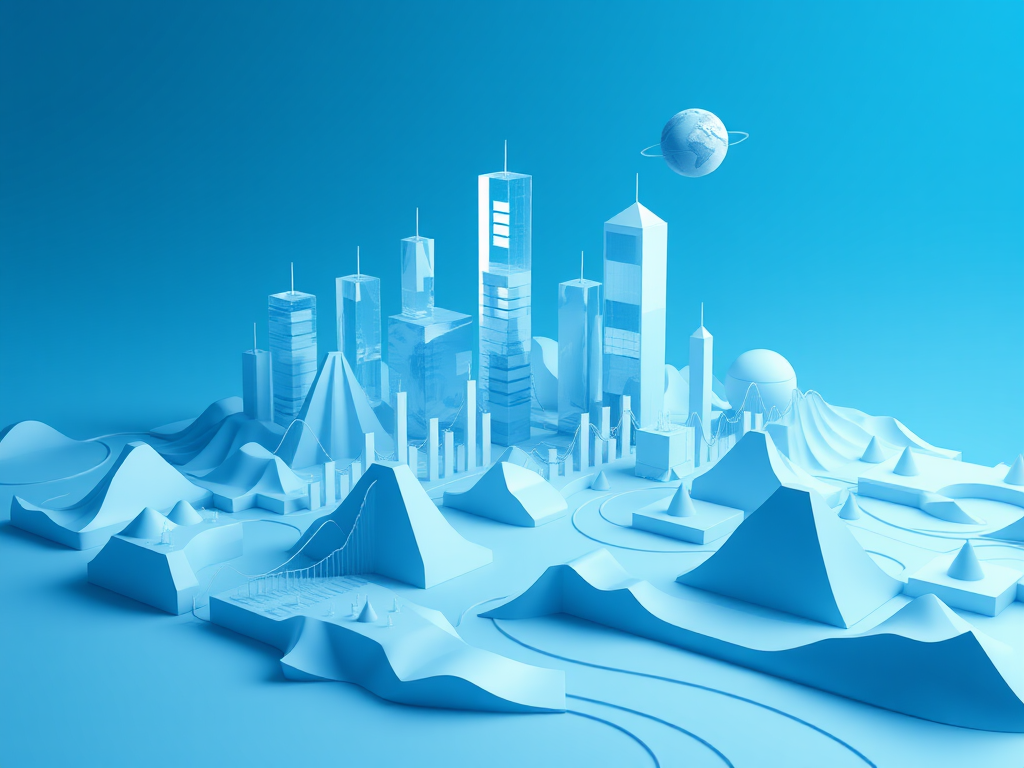
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
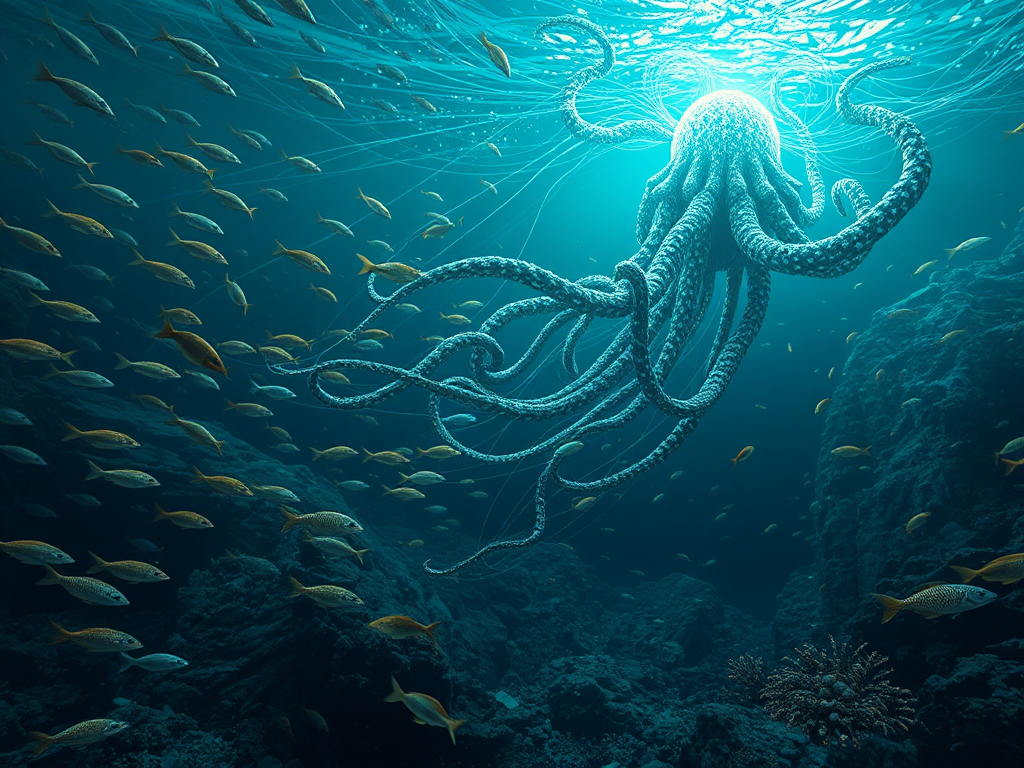
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
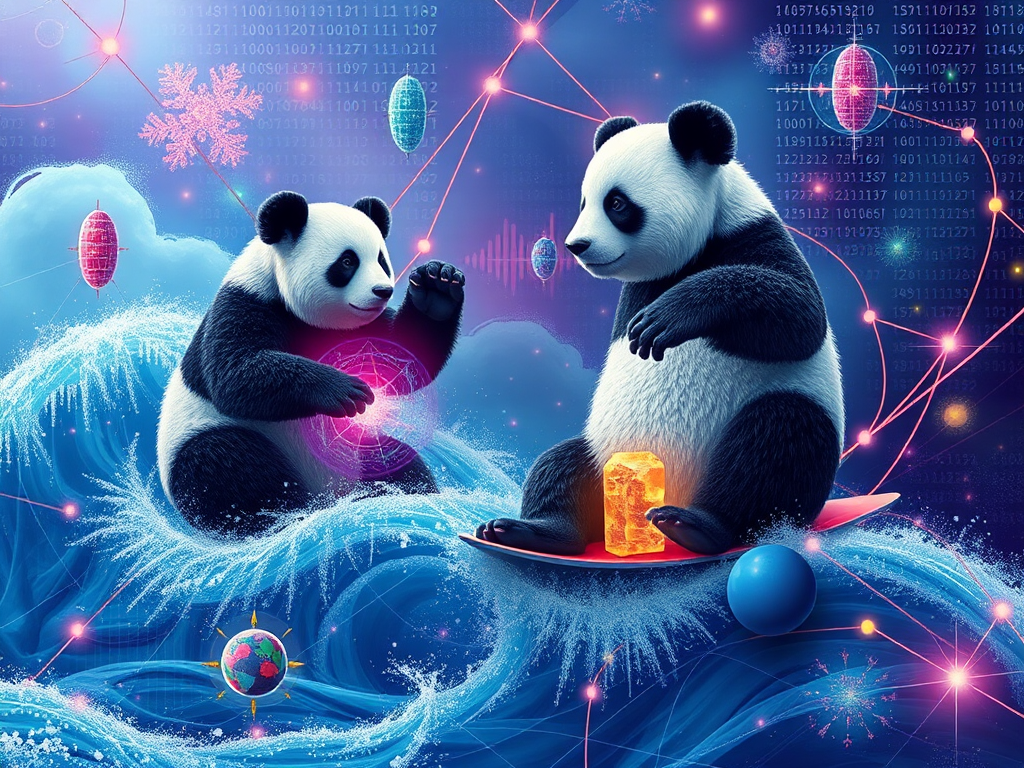
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts