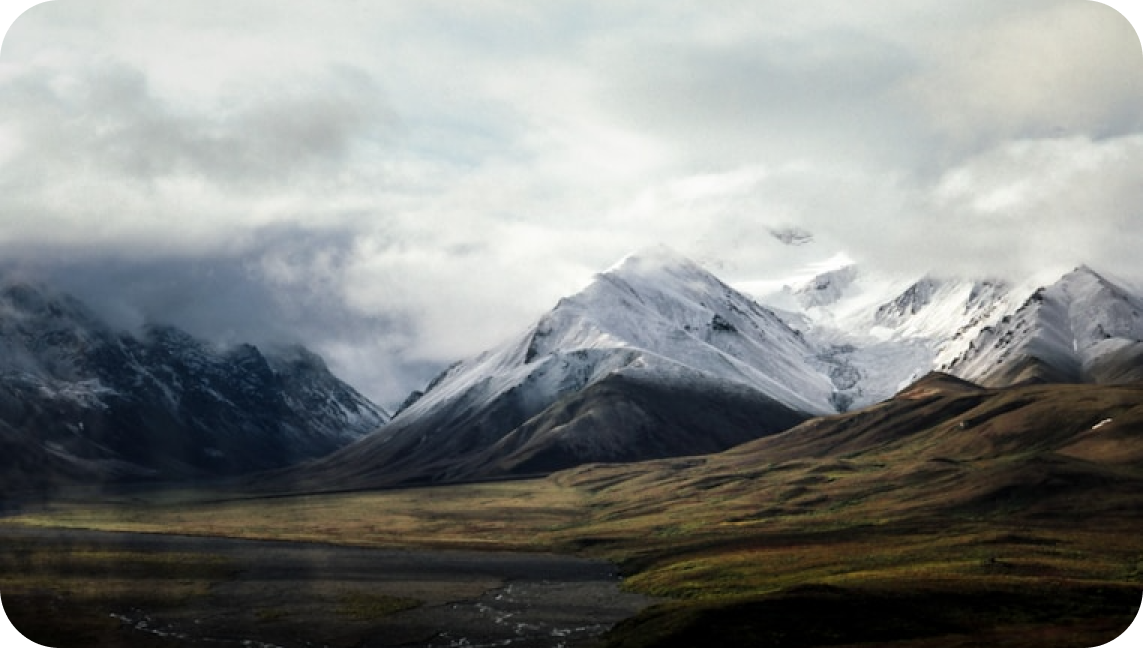
Introduction
Have you ever been frustrated by slow Python program execution when handling large-scale data? As a Python data analysis engineer, I deeply understand the importance of performance optimization. Today, let me share with you how to improve DataFrame operations speed by more than 10 times.
Basic Knowledge
Before diving deep, we need to understand the internal structure of DataFrame. A DataFrame is essentially a two-dimensional table, but it's implemented based on NumPy arrays. This is important because it directly affects how we optimize performance.
Let's look at a simple example:
import pandas as pd
import numpy as np
df = pd.DataFrame({
'A': np.random.rand(1000000),
'B': np.random.randint(0, 100, 1000000),
'C': np.random.choice(['X', 'Y', 'Z'], 1000000)
})
Want to know how this DataFrame is stored in memory? Actually, each column is stored as an independent array. This columnar storage design is crucial for data analysis since we often need to operate on specific columns.
Performance Pitfalls
In my practice, I've discovered some common performance pitfalls that we need to pay special attention to:
- Loop Operations
Many Python programmers are used to using for loops to process data, but this is often a fatal mistake in Pandas. I've seen code like this:
for index, row in df.iterrows():
df.at[index, 'D'] = row['A'] * 2 + row['B']
While this code is intuitive, its performance is extremely poor. Why? Because each iteration creates a Series object, which brings significant overhead. The correct approach is:
df['D'] = df['A'] * 2 + df['B']
- Memory Usage
Memory management is crucial when handling large-scale data. I often see code like this:
df_copy = df.copy()
df_copy['E'] = df_copy['A'] + df_copy['B']
result = df_copy['E'].mean()
This creates a complete DataFrame copy, but we only need to calculate the mean. A better approach is:
result = (df['A'] + df['B']).mean()
Optimization Techniques
Through years of practice, I've summarized some effective optimization techniques:
- Data Type Optimization
Choosing appropriate data types can significantly reduce memory usage. For example:
df['category'] = df['category'].astype('object')
df['category'] = df['category'].astype('category')
After using the category type, memory usage in one of my actual projects decreased by 80%. This is because the category type internally uses integers to store repeated string values.
- Batch Operations
When multiple DataFrame modifications are needed, batch operations are much faster than row-by-row operations:
for value in values:
df = df[df['A'] != value]
df = df[~df['A'].isin(values)]
- Parallel Processing
For large-scale data, utilizing multi-core processors can significantly improve performance:
import pandas as pd
import numpy as np
from multiprocessing import Pool
def process_chunk(chunk):
return chunk['A'].mean()
chunks = np.array_split(df, 4)
with Pool(4) as pool:
results = pool.map(process_chunk, chunks)
final_result = np.mean(results)
Real-world Case Study
Let me share a real optimization case. In a financial data analysis project, we needed to process over 10 million rows of transaction data. The initial version took over an hour to process, but after optimization, it was reduced to 5 minutes.
The main optimization steps included:
- Data Type Optimization:
df['transaction_type'] = df['transaction_type'].astype('category')
df['timestamp'] = pd.to_datetime(df['timestamp'])
df['amount'] = df['amount'].astype('float32') # Downgraded from float64
- Query Optimization:
mask = df['timestamp'].dt.date == target_date
filtered = df[mask]
df.set_index('timestamp', inplace=True)
filtered = df.loc[target_date.strftime('%Y-%m-%d')]
- Aggregation Operation Optimization:
result = df.groupby('customer_id').apply(lambda x: complex_calculation(x))
def complex_calculation(group):
# Pre-calculate commonly used values
mean_amount = group['amount'].mean()
total_transactions = len(group)
return pd.Series({
'avg_amount': mean_amount,
'transaction_count': total_transactions,
'total_value': mean_amount * total_transactions
})
result = df.groupby('customer_id').agg({
'amount': ['mean', 'count', 'sum']
})
Experience Summary
Through years of practice, I've summarized the following experiences:
- Always Pay Attention to Memory Usage
- Use appropriate data types
- Release unnecessary data promptly
-
Avoid unnecessary data copying
-
Fully Utilize Vectorized Operations
- Avoid loops whenever possible
- Use built-in functions instead of custom functions
-
Process data in batches rather than one by one
-
Use Indexes Wisely
- Create indexes for frequently queried columns
- Reset indexes at appropriate times
- Choose appropriate index types based on query patterns
Performance Monitoring
To optimize well, performance monitoring is essential. My commonly used method is:
import time
import memory_profiler
def measure_performance(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"Function {func.__name__} took {end_time - start_time:.2f} seconds")
return result
return wrapper
@measure_performance
def process_data(df):
# Data processing logic
pass
Future Outlook
As data scale continues to grow, performance optimization will become increasingly important. I believe future development trends include:
- More GPU acceleration support
- Smarter memory management
- More powerful parallel processing capabilities
Which of these optimization techniques do you find most helpful for your work? Feel free to share your experiences and thoughts in the comments.
Practical Suggestions
Finally, I want to give you some practical suggestions:
- Identify bottlenecks before starting optimization
- Use performance analysis tools to guide optimization
- Maintain code readability during optimization
- Establish benchmarks to ensure optimization effectiveness
- Document optimization processes to form best practices
Remember, performance optimization is an ongoing process that requires continuous learning and practice. I hope this article helps you go further in your Python data analysis journey.
Do you have any unique optimization techniques to share? Or have you encountered any special problems in practice? Feel free to discuss in the comments.
Next
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts
Next
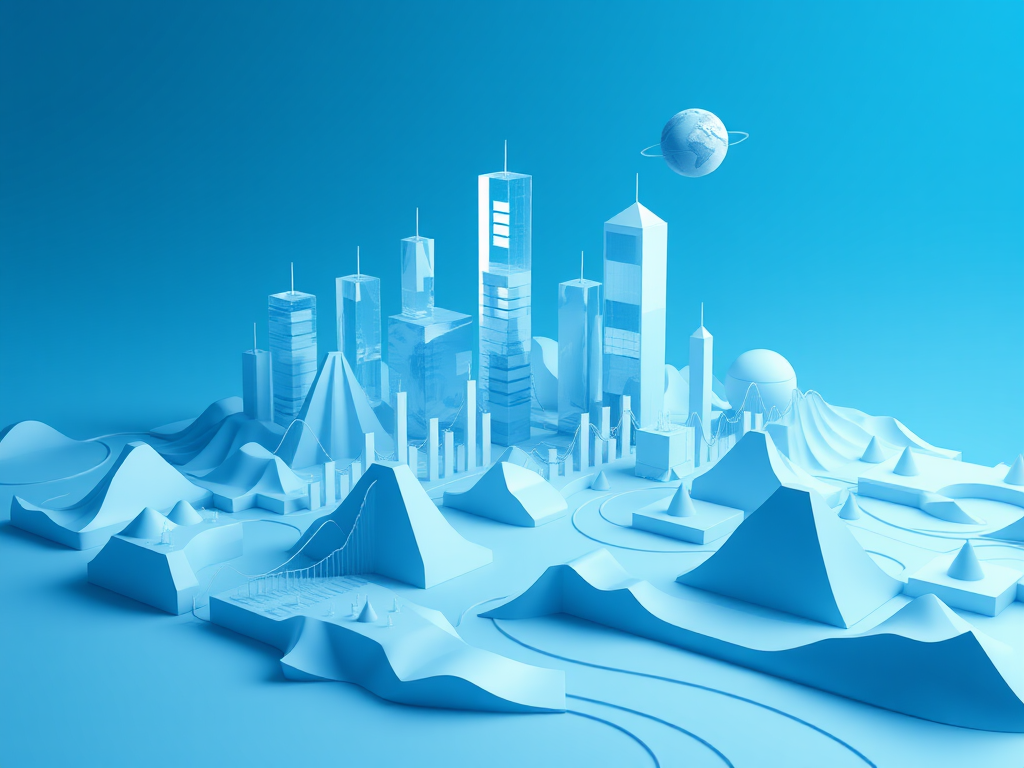
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
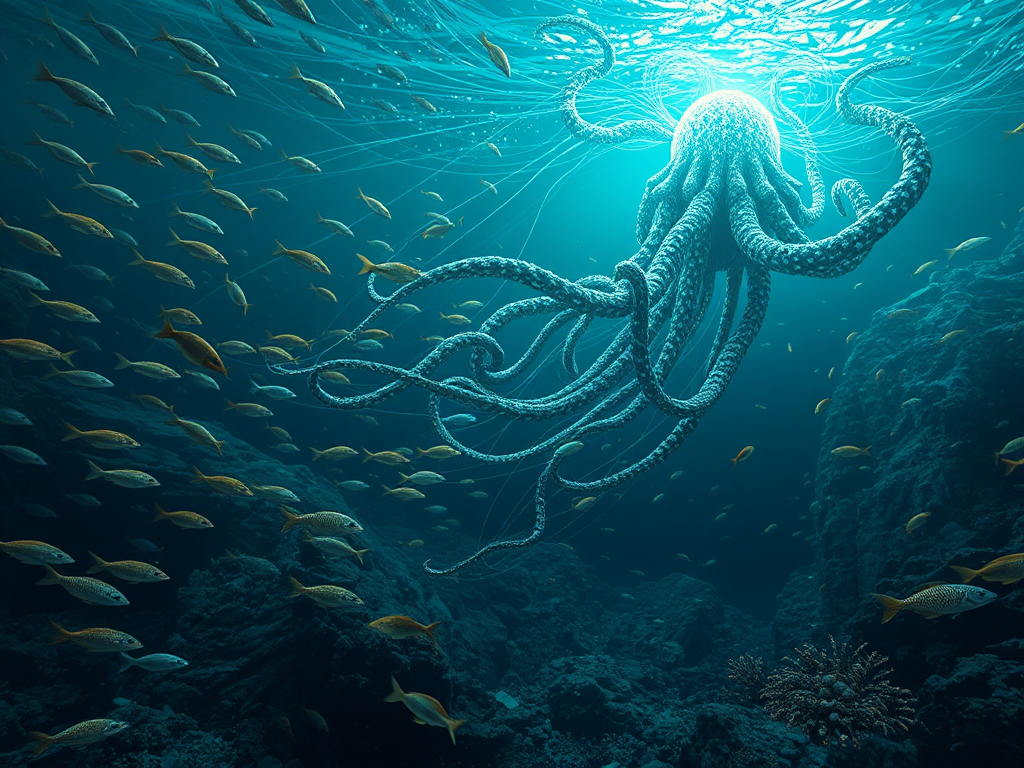
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
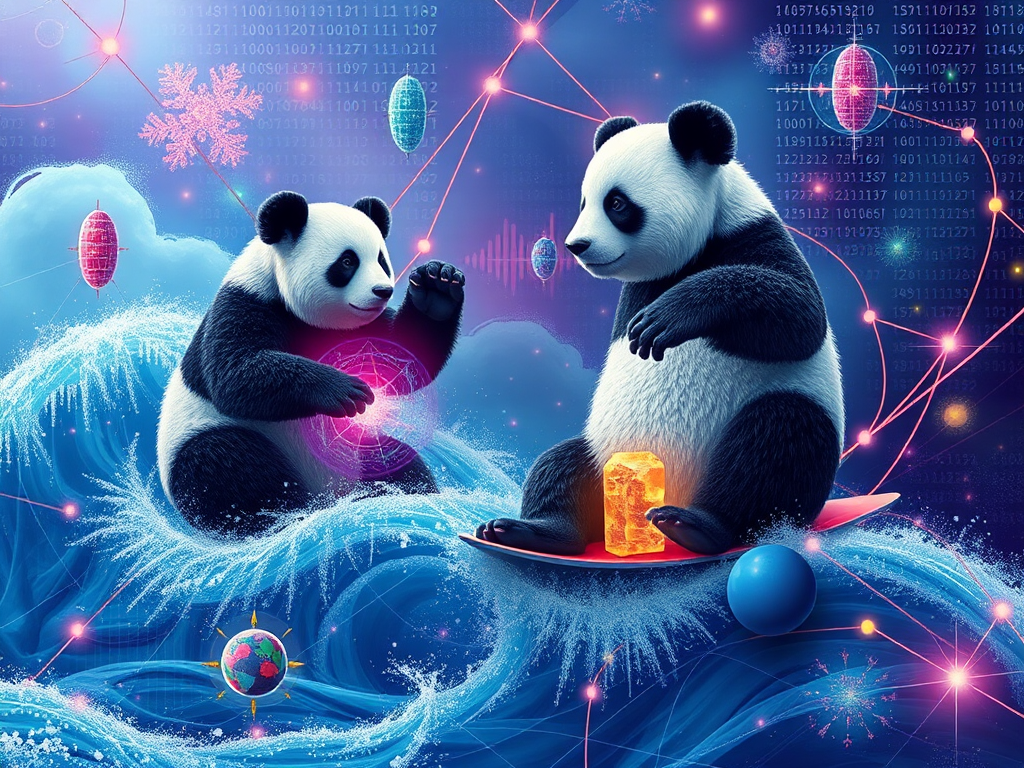
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts