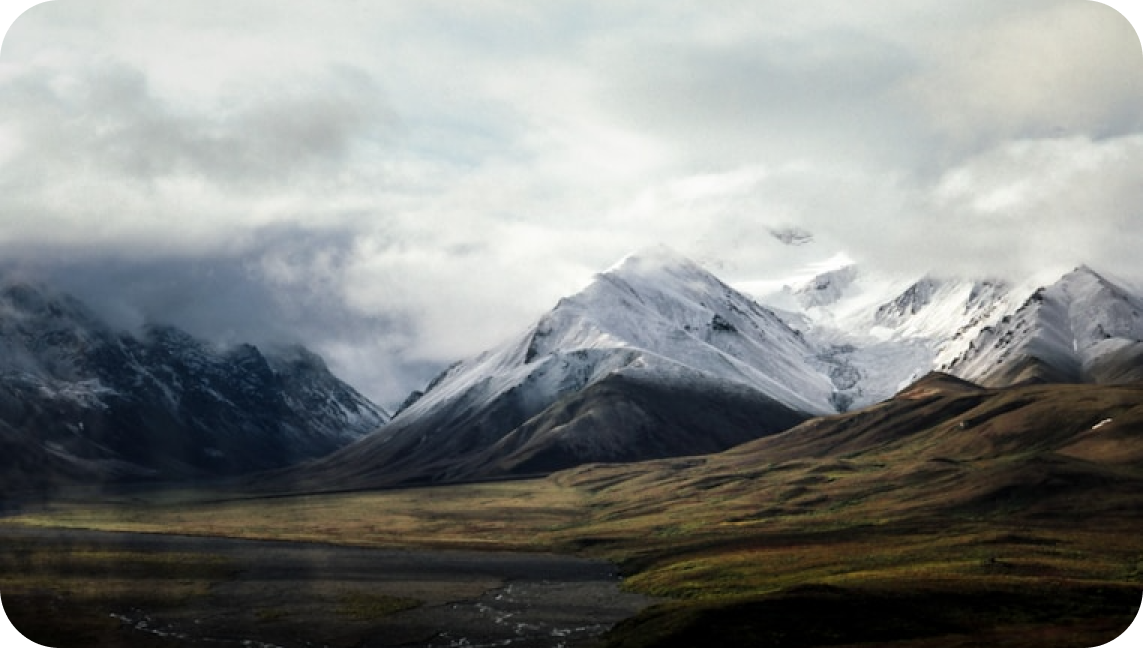
The Charm of Python
Hello everyone, today I'd like to share with you the applications of Python in the field of web development. I believe many of you have heard of Python. As a simple and easy-to-learn language, it has wide applications in many fields. Especially in web development, Python is definitely an invaluable tool.
You might ask, what charm does Python have that makes it so popular in web development? Let me explain one by one.
Simple and Friendly Syntax
The first charm is Python's concise and readable syntax. Its syntax rules are clear and simple, with fewer lines of code and high readability, making it easy for beginners to get started. This is a huge advantage for rapid web application development.
Think about it, if the syntax is too complex and obscure, development efficiency would be greatly reduced. Python, with its concise and clean syntax, allows developers to focus more energy on business logic, thus improving development efficiency. Do you have any experience with this?
Rich and Practical Frameworks
Besides simple syntax, Python's greatest charm is perhaps its rich frameworks. Whether it's the full-stack Django or the micro-framework Flask, they can help you quickly develop powerful web applications.
Take Django for example, it provides many built-in features such as ORM, user authentication, and admin interface, ready to use out of the box, which can greatly improve development efficiency. Flask, on the other hand, is lightweight and flexible, suitable for building small applications and microservices. Whether you're working on large or small projects, there's always a Python framework that suits you.
Active Community and Rich Documentation
Moreover, Python has a strong and active community. No matter what problems you encounter, you can always find solutions in the community. Also, Python's official documentation is very well written, benefiting both beginners and experts.
I myself have benefited a lot from the official documentation and community during my learning process. As long as you have the heart to learn, you can definitely master the Python language. Python's community resources are indeed one of its major advantages.
Cross-platform Portability
Lastly, it's worth mentioning that Python has good cross-platform characteristics. Whether you use Windows, Linux, or Mac systems, Python code can run directly without much modification. This is a great feature for web development, making your applications more portable.
So, Python not only has simple syntax, rich frameworks, and an active community, but also cross-platform advantages. With these charms, it's no wonder it's so popular in the field of web development.
Picking Between Big and Small Frameworks
Although Python has many charms in the field of web development, should you choose a big framework like Django or a small framework like Flask? This needs to be decided based on the actual needs of the project. But before that, let's understand the differences between the two.
Django: The All-rounder
Django can be said to be an all-round Python web framework. It provides a complete solution from development to deployment, with built-in ORM, admin interface, user authentication, caching, and many other features. For large web applications, Django is undoubtedly a good choice.
Developing with Django can make your code structure clearer and more standardized, and development efficiency will be relatively higher. Of course, Django's learning curve is also relatively steep, with a higher entry cost for beginners.
But through official documentation and community resources, I believe everyone can gradually master the use of Django. Personally, I think that although Django has a higher entry difficulty, once you master it, it can show its unique charm when developing large web applications.
Flask: Simple and Flexible
Unlike Django, Flask is a micro Python web framework. Its core code is very concise, containing only the most basic functions, and the rest needs to be added by developers according to their needs.
This design philosophy makes Flask very flexible and suitable for building small web applications and RESTful API services. If your project scale is small, or you focus more on flexibility, then Flask is definitely a good choice.
However, Flask's flexibility also means that developers need to build the entire application architecture themselves, which is somewhat challenging for beginners. But fortunately, the Flask ecosystem also has many excellent extension libraries that can make up for the shortcomings of the framework.
So, if your project scale is small or you have high requirements for flexibility, then choose Flask. Although it's relatively simple to get started, it can still create excellent web applications when used to its fullest.
Advice on Choosing
Whether to choose Django or Flask, my advice is to decide based on the actual needs of the project. If it's a large project and you have high requirements for development efficiency and standardization, then choose Django. If it's a small project, or you value flexibility more, Flask is a better choice.
Of course, if you're new to web development, I suggest starting with Flask as it's relatively easier. After you're familiar with Flask, learning Django will be more manageable.
No matter which framework you choose, remember that practice is very important. Only by constantly practicing in real combat can you truly master the essence of Python web development. Come on, I wish you all can become experts in Python web development!
Django Practical Tips
As an all-round Python web framework, Django naturally has many practical tips worth mastering. Next, let's look at what details we need to pay attention to in Django development.
User Authentication
User authentication is an unavoidable part of any web application. Fortunately, Django provides us with a built-in user authentication system that can greatly simplify the development process. We only need to follow these steps:
-
Use the built-in user model. Django provides a default
User
model that can be used directly without creating a new one. -
Configure authentication backend. Set
AUTHENTICATION_BACKENDS
insettings.py
to specify which authentication backends to use. -
View and form processing. Use forms provided by Django such as
UserCreationForm
andAuthenticationForm
to handle user registration, login, etc. -
Protect views. Use the
@login_required
decorator to protect views that require authentication, unauthenticated users will be redirected to the login page.
Through these simple steps, we can add user authentication functionality to our Django application. Django's built-in authentication system is powerful and can meet most application scenarios. If customization is needed, it can also be extended and modified according to requirements.
Have you used the user authentication system in your own Django project? If you encounter any problems, you can also leave a message in the comments section, and we can discuss together.
Performance Optimization
For any web application, performance optimization is an eternal topic. As a large framework, Django naturally provides us with various means of performance optimization.
Using Cache
First, we need to make good use of Django's caching framework to reduce database queries. Caching some infrequently changing data in memory can greatly improve the response speed of the application. Django supports multiple caching backends, such as Memcached, Redis, etc., which can be selected and configured according to needs.
Optimizing Database Queries
Secondly, we need to pay attention to optimizing database queries. Reasonable use of indexes, effectively avoiding N+1 query problems, reducing unnecessary data loading, etc., are all good optimization methods. Although Django's ORM is convenient to use, it's also easy to cause performance problems, so we need to pay extra attention.
Managing Static Files
Moreover, proper management of static files is also a key point for optimization. Django provides the collectstatic
command to collect static files, which we can deploy with CDN to improve the loading speed of static files.
Asynchronous Task Processing
Finally, for some time-consuming tasks, we can consider using tools like Celery for asynchronous processing. This can avoid blocking the main thread and improve the responsiveness of the application.
Through the above aspects of optimization, I believe everyone can greatly improve the performance of Django applications. However, it should be noted that any optimization method needs to be used according to the scenario, and excessive optimization should be avoided to prevent the code from becoming difficult to maintain. We need to balance between performance and maintainability.
Personal Summary
In summary, Django, as a large web framework, provides us with many conveniences in user authentication and performance optimization. Mastering these skills can help us better develop and optimize Django applications.
Of course, in addition to the few tips mentioned above, Django has many other areas worth exploring, such as admin backend, middleware, signals, and so on. We need to continuously learn and summarize in practice to truly master the essence of Django development. Let's move forward together on this path and become true Django experts!
Flask Development in Practice
Compared to large frameworks like Django, Flask appears more concise and lightweight. But this doesn't mean Flask doesn't have techniques worth mastering. As an excellent micro-framework, Flask has its unique charm. Next, let's explore the essence of Flask development!
Building Basic Applications
As a micro-framework, Flask's core code is very concise. But it's this simple design philosophy that gives Flask great flexibility and extensibility. To build a basic Flask application, you only need a few simple steps:
-
Import the Flask class and create an application instance.
-
Define routes using the
@app.route
decorator. -
Write view functions that return response content.
-
Run the application using
app.run()
to start the server.
It's that simple, and a basic Flask application can be up and running. Of course, in actual development, we still need to add more features, such as databases, template engines, etc.
However, no matter how complex the application is, the basic idea always starts with these simple steps. Flask is such a low-threshold, concise, and efficient framework that is worth mastering.
Form Processing
Any web application can't do without form processing. Although Flask itself doesn't provide a form library, we can use the Flask-WTF extension to quickly handle forms.
-
Install Flask-WTF and initialize it in the application.
-
Define form classes inheriting from
FlaskForm
. -
Add fields using various
Field
subclasses. -
Data validation using built-in or custom validators.
-
View processing to receive and process submitted form data.
-
Template rendering to render the form in the template.
Through these steps, we can seamlessly integrate form processing functionality into Flask applications. The Flask-WTF extension is powerful, not only can it quickly generate forms, but it also supports advanced features such as CSRF protection and file uploads.
Form processing is one of the most common aspects of web development. Mastering the Flask-WTF extension is equivalent to mastering an important skill in Flask development.
Personal Summary
As a micro-framework, Flask's core functionality is very concise. But it's this simple design philosophy that gives Flask great flexibility and extensibility. By choosing appropriate extensions, we can build feature-rich web applications on top of Flask.
In addition to the basic application building and form processing mentioned above, Flask has many other aspects worth learning, such as blueprints, testing, deployment, etc. We need to continuously explore in practice to truly master the essence of Flask development.
Flask may be simple, but it's not simplistic. It has great potential behind it, and as long as we work hard to study, we can definitely go far on the path of Flask development. Let's work hard together and become experts in Flask development!
Web Development Best Practices
Whether using Django or Flask, as web developers, we need to always keep in mind some best practices to ensure the security, maintainability, and scalability of our applications. Next, let's talk about those things in web development.
Security
For any web application, security is the most important aspect. We need to ensure the security of the application from various angles, such as preventing CSRF attacks, XSS attacks, etc.
Both Django and Flask provide corresponding security protection mechanisms. For example, Django enables CSRF middleware by default, which can effectively defend against CSRF attacks; while Flask requires us to manually enable CSRF protection.
In addition, we also need to pay attention to preventing XSS attacks. Both Django and Flask provide corresponding tool functions that can escape user input, thereby avoiding XSS attacks.
In addition to framework-level protection, we also need to pay attention to some security details in coding practices, such as not directly concatenating user input into SQL statements, using parameterized queries, etc. Only by starting from various levels can we truly ensure the security of the application.
Code Organization
Good code organization is the key to ensuring project maintainability. Regardless of which framework is used, we need to follow the principles of modular design and reasonably split the code according to responsibilities.
In addition, the use of version control systems such as Git is also essential. Every time we commit code, we need to add clear comments explaining what changes were made in this commit. The change record of the code is clear at a glance, making later maintenance and rollback more convenient.
Moreover, we also need to unify the code style, such as following the PEP 8 specification. This not only improves the readability of the code but also facilitates multi-person collaborative development. If there are multiple people in the team, it's best to formulate a coding specification and implement it uniformly.
Testing and Debugging
Testing is very important for any application. Whether it's unit testing or integration testing, we need to write test cases for the code to ensure its robustness.
Both Django and Flask provide corresponding test frameworks that allow us to conveniently write and run test cases. We need to continuously test during the development process, discover and fix bugs as early as possible, rather than waiting until the end to test.
In addition, the use of debugging tools is also an indispensable part. Whether it's Django's debug toolbar or Flask's debug mode, they can greatly improve our debugging efficiency. We need to master the use of these debugging tools to speed up problem location and resolution.
Summary
Through the above sharing, I believe everyone has gained a deeper understanding of some best practices in web development. Regardless of what framework is used, we need to always keep in mind the importance of security, code organization, testing and debugging, etc., to ensure the quality of the application.
These are just some basic practices in web development, we have more to learn and improve in the future. However, as long as we continue to learn and practice, we can definitely go further on this path and become true web development experts. Let's work hard together and make progress together!
Next
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit
Next
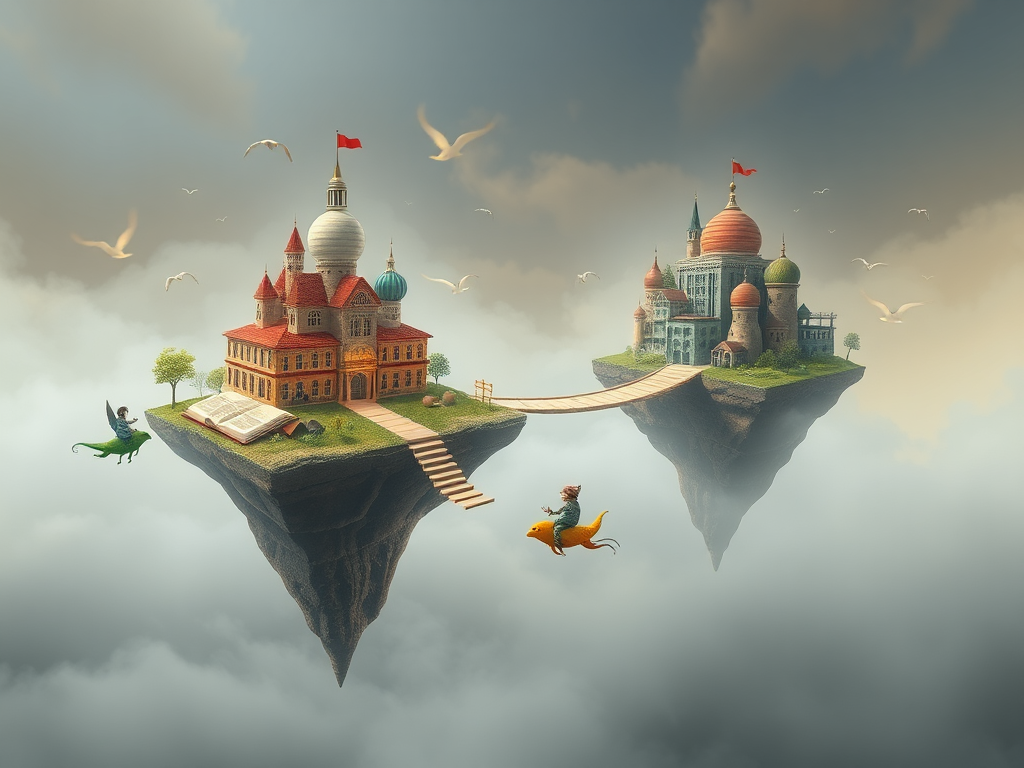
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
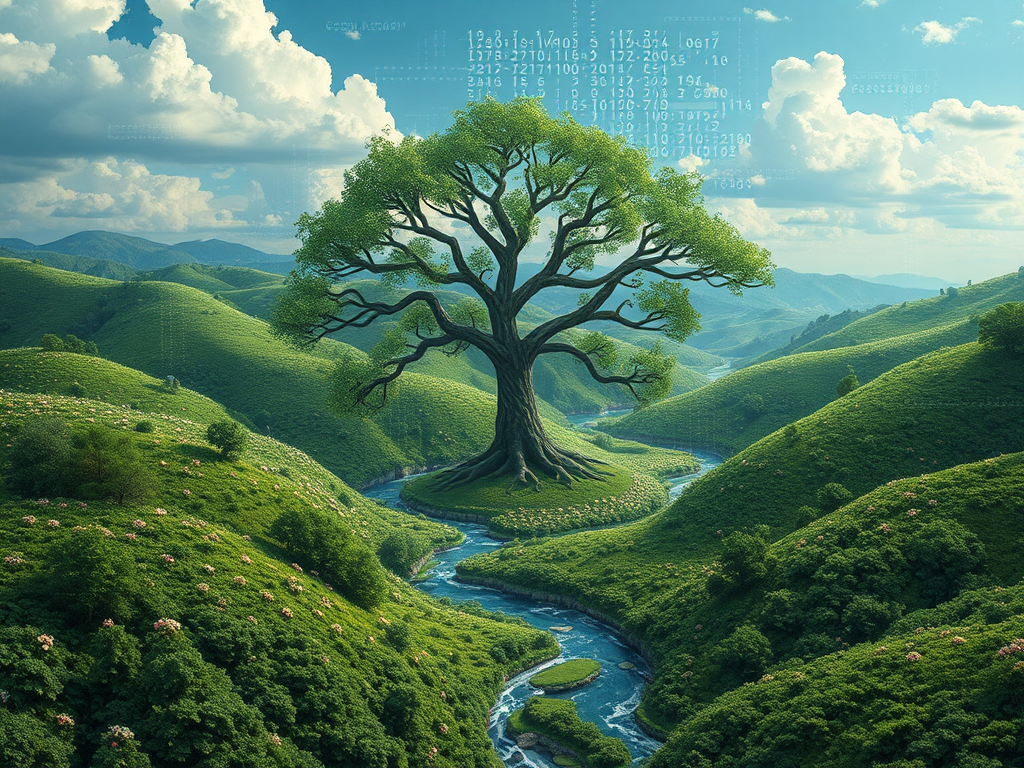
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
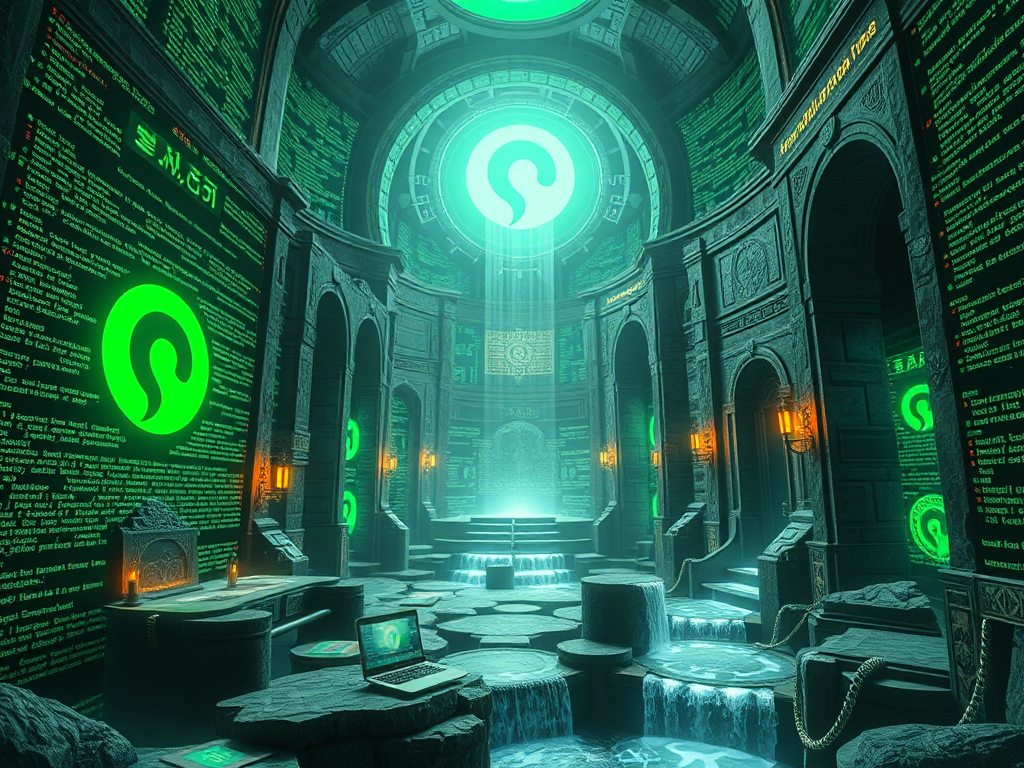
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit