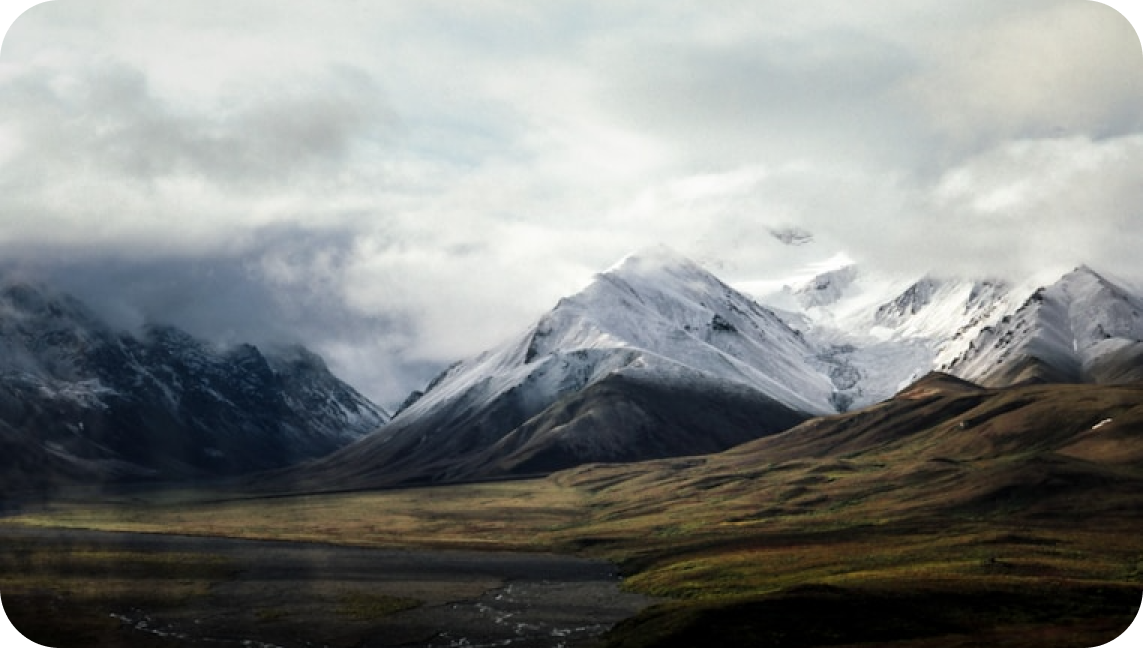
Introduction
Hello, dear friend! Welcome to my Python programming blog. As an experienced Python developer and blogger, I will share programming knowledge here, along with some personal insights and learning experiences. Let's embark on a wonderful journey to explore Python together, seeking fun and a sense of achievement in the world of coding.
Application of Jinja
I'm sure you've encountered this situation: when developing a Web application using the Flask framework, we want to render some dynamic data into HTML templates, but the Jinja code appears as plain text on the webpage. Don't worry, the solution is right in front of you!
First, we need to ensure that Flask and Jinja modules are correctly imported in the app.py file. Then, properly render the Jinja template and correctly reference Jinja variables in the HTML file. See, isn't it simple? Once you've mastered these basics, you can easily display dynamic content on your webpage.
File Operations
In the programming process, we often need to manipulate files and directories. For example, you need to run a Python script located in a different folder to extract files. Don't worry, Python provides us with powerful modules to handle these tasks.
First, use the os.chdir() function to switch to the target folder. Then, use the os.path.join() function to concatenate file paths, ensuring the path is correct. Finally, use the subprocess module to run the Python script, and you can complete the task. See, isn't it simple and practical? Once you've mastered these techniques, you can freely manipulate files and directories in your programming.
Priority Queue
In data structures and algorithms, priority queue is a very important concept. It's often used in various scenarios, such as task scheduling, network routing, etc. Have you ever wondered which priority queue implementation is the fastest when insertion operations are frequent?
Don't rush, I'll tell you the answer. Usually, using the priority queue implementation provided by Python's built-in heapq module is the fastest choice. The heapq module is implemented based on binary heap, which has good performance in both insertion and deletion of the smallest element operations. So, if you need to frequently insert elements, use the heapq module, it will certainly meet your needs.
Nested Dictionaries
In Python, dictionaries are a very powerful and flexible data structure. However, when you need to deal with nested dictionaries with partially overlapping keys, the situation becomes a bit tricky. Don't worry, we have multiple solutions to choose from.
First, you can use collections.ChainMap to merge dictionaries, preserving the overlapping parts. Additionally, you can use recursive functions to traverse nested dictionaries and merge overlapping parts. Finally, you can also use the dict.update() method to update dictionaries, preserving the overlapping parts.
See, aren't there many methods to choose from? Which one to choose depends on your specific needs and preferences. However, no matter which method you choose, it's very important to ensure the readability and maintainability of your code.
Image Brightness Analysis
In the field of image processing, we often need to analyze the brightness level of images. For example, brightness analysis becomes very important in image enhancement or image classification tasks. So, how do we identify the brightness level of an image?
First, you can use the PIL (Python Imaging Library) library to calculate the average brightness value of the image. Additionally, you can use the OpenCV library to calculate the histogram of the image and determine the brightness level based on the histogram distribution. Finally, you can also use the scikit-image library to calculate brightness statistical indicators of the image, such as average brightness, standard deviation, etc.
See, aren't there many methods to choose from? Which one to choose depends on your specific needs and preferences. However, no matter which method you choose, it's very important to ensure the readability and maintainability of your code.
Conclusion
In this blog post, we discussed some common problems and solutions in Python programming. I hope that through these examples, you can better understand and master Python programming techniques.
Remember, programming is not just a technology, but also a source of fun and a sense of achievement. Keep your curiosity, be brave to explore, and you will surely gain a lot of happiness and a sense of achievement on your programming journey.
See you next time, happy coding!
Next
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc
Next
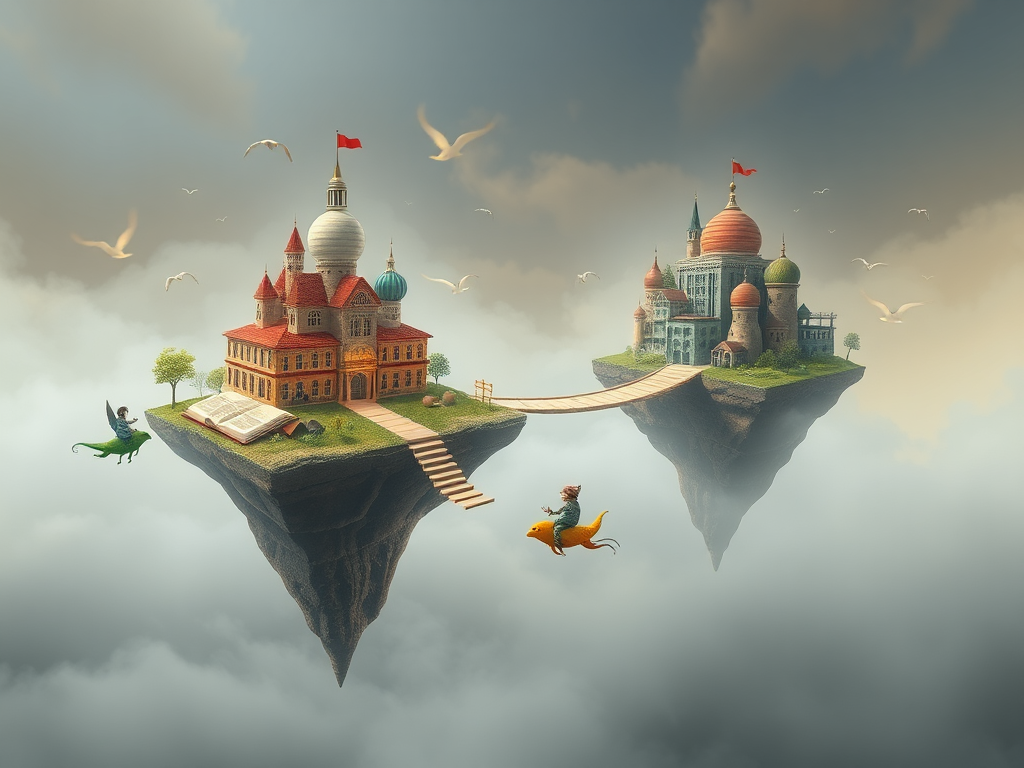
Python Web Development, Simple and Practical
Explore the advantages of Python in web development, including framework selection, form handling, RESTful API implementation, and user authentication. The arti
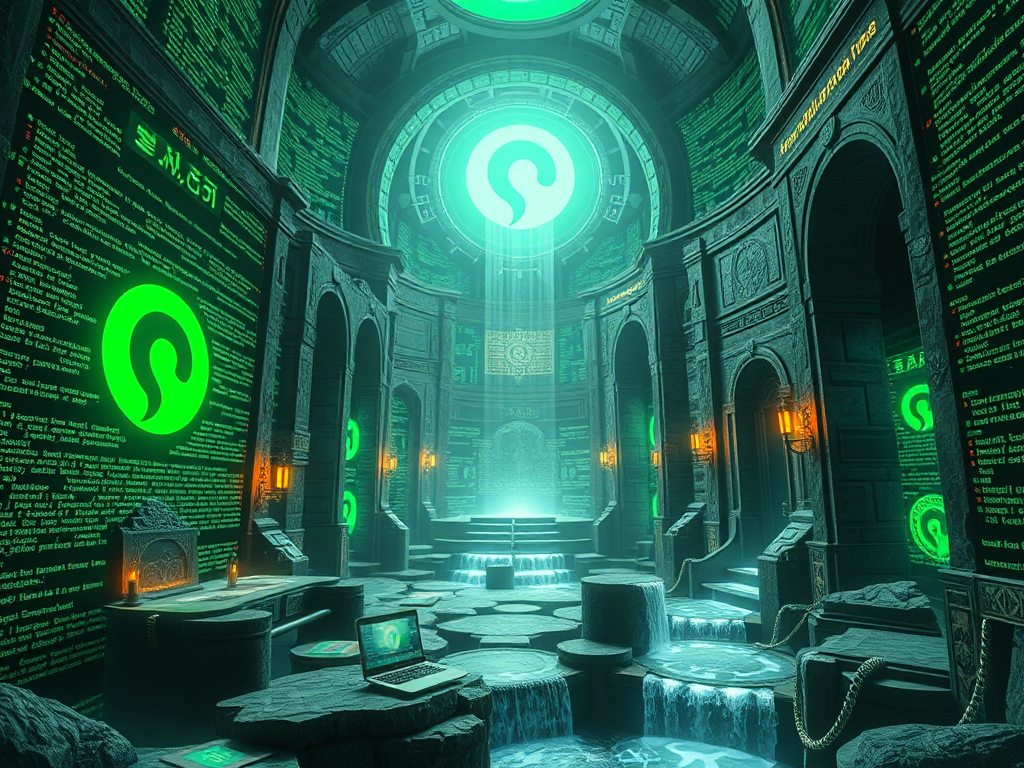
Building Reliable Backend Services for Web Applications with Python - A Complete Guide from Basics to Practice
A comprehensive guide to Python web development, covering framework advantages, Django and Flask features, development environment setup, project implementation, and real-world applications from companies like Netflix and Reddit
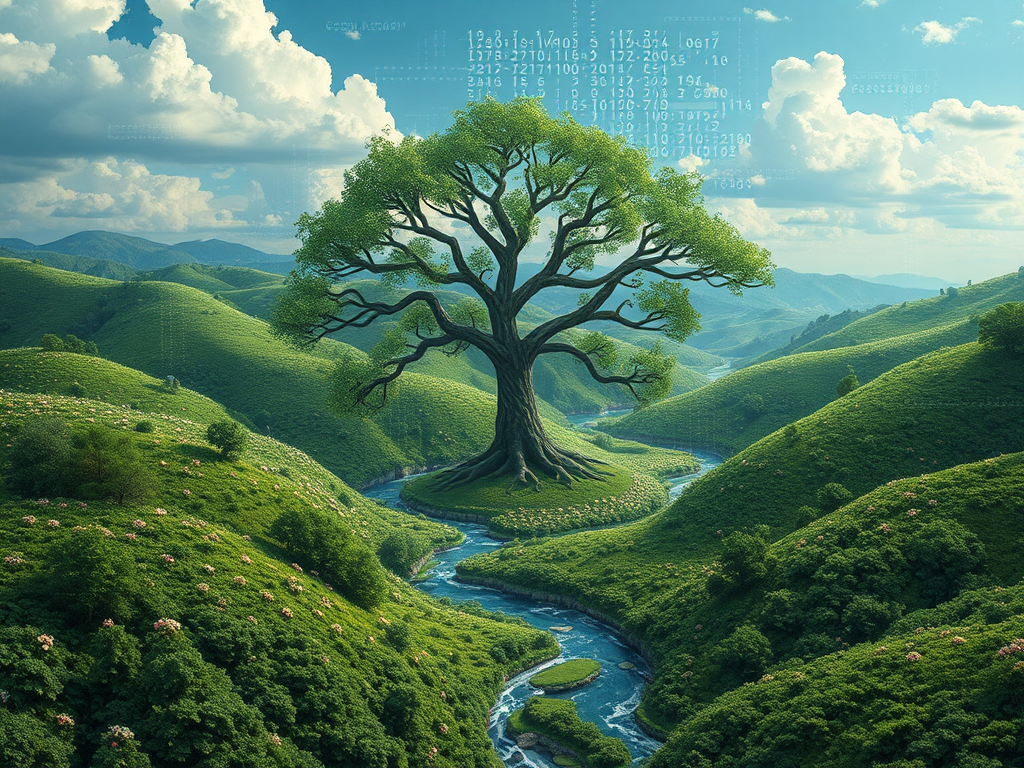
Practical Tips for Python Web Development
This article shares some practical tips for Python Web development, including handling image uploads and storage in FastAPI, executing complex queries in SQLAlc