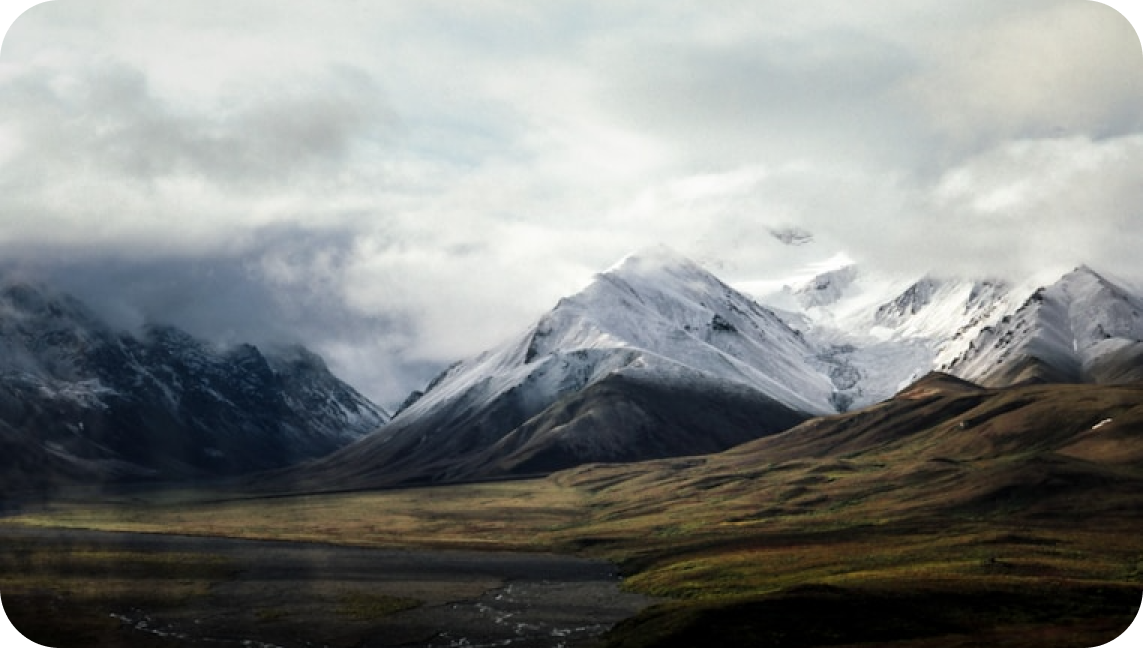
Hey, Python data analysis enthusiasts! Today, let's talk about an interesting and practical topic - text mining! Are you often overwhelmed by large amounts of text data? Don't worry, today I'll teach you how to easily handle text data using Python and extract valuable information from it.
Getting Started
First, we need to understand what text mining is. Simply put, it's extracting useful information and knowledge from large amounts of unstructured text data. Sounds fancy, right? Actually, it's not. With the right tools and techniques, you can easily get started!
In Python, we mainly use two powerful libraries: NLTK and spaCy. NLTK is like a treasure chest, filled with various text processing tools; while spaCy is an efficient natural language processing engine, especially suitable for processing large-scale text.
NLTK: Your Text Processing Toolbox
Let's start with NLTK. Installation is simple, just enter in the command line:
pip install nltk
Once installed, we can start using it. For example, if we want to split a piece of text into words, we can do this:
import nltk
nltk.download('punkt') # Download tokenization model
text = "Python is a powerful and concise programming language."
tokens = nltk.word_tokenize(text)
print(tokens)
When you run this code, you'll see the text split into individual words. Isn't it amazing? That's the magic of tokenization!
NLTK can do even more interesting things. For example, we can use it to find the most common words in a piece of text:
from nltk import FreqDist
text = "Python is a powerful and concise programming language. Python can be used in web development, data analysis, artificial intelligence, and many other fields."
tokens = nltk.word_tokenize(text)
fdist = FreqDist(tokens)
print(fdist.most_common(5)) # Output the 5 most frequently occurring words
See the results? This is word frequency statistics, which is very useful in text analysis.
spaCy: Efficient Text Analysis Tool
After talking about NLTK, let's look at spaCy. spaCy's installation is also simple:
pip install spacy
python -m spacy download en_core_web_sm # Download English model
One of spaCy's main features is its speed, especially suitable for processing large-scale text. Let's look at an example:
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp("Python is a powerful and concise programming language.")
for token in doc:
print(token.text, token.pos_) # Output each word and its part of speech
See that? spaCy can not only tokenize but also automatically determine the part of speech for each word. This is very useful in many natural language processing tasks.
Diving Deeper into Text Mining: Topic Modeling
Alright, now that we've mastered the basic tools, it's time to go a bit deeper. Let's look at topic modeling - a technique for discovering topics from a large number of documents.
In Python, we can use the gensim library for topic modeling. Let's install it first:
pip install gensim
Then, let's look at a simple example:
from gensim import corpora
from gensim.models import LdaModel
from gensim.parsing.preprocessing import STOPWORDS
from gensim.utils import simple_preprocess
documents = [
"Python is a powerful programming language",
"Data analysis is important in business decision-making",
"Machine learning is a branch of artificial intelligence",
"Python can be used for web development and data science"
]
texts = [[word for word in simple_preprocess(doc) if word not in STOPWORDS]
for doc in documents]
dictionary = corpora.Dictionary(texts)
corpus = [dictionary.doc2bow(text) for text in texts]
lda_model = LdaModel(corpus=corpus, id2word=dictionary, num_topics=2)
print(lda_model.print_topics())
When you run this code, you'll see two topics found by the model. Each topic consists of some keywords that reflect the main content of the document collection.
Isn't it amazing? Through topic modeling, we can quickly understand the main content of a large number of documents, which is particularly useful in scenarios such as processing news articles and social media posts.
Sentiment Analysis: Understanding the Emotions Behind the Text
Finally, let's look at another interesting application - sentiment analysis. As the name suggests, it's about analyzing the sentiment contained in the text.
In Python, we can use the TextBlob library for simple sentiment analysis. Let's install it first:
pip install textblob
Then, let's look at an example:
from textblob import TextBlob
text = "I really like Python, it makes programming so simple and fun!"
blob = TextBlob(text)
sentiment = blob.sentiment.polarity
if sentiment > 0:
print("This is a positive review")
elif sentiment < 0:
print("This is a negative review")
else:
print("This is a neutral review")
When you run this code, you'll see the program determines that this is a positive review. Isn't it interesting? Through sentiment analysis, we can automatically judge the sentiment of large amounts of text, which is very useful in scenarios such as analyzing user comments and social media public opinion.
Summary
Alright, today we've learned quite a few text mining techniques, from basic tokenization and word frequency statistics to more advanced topic modeling and sentiment analysis. These techniques are very useful in actual data analysis work.
Have you noticed that text data actually contains rich information? As long as we master the right tools and methods, we can extract valuable insights from it. This is the charm of text mining!
Of course, what we've learned today is just the tip of the iceberg. There are many advanced techniques in text mining, such as named entity recognition, relationship extraction, and so on. If you're interested in these, feel free to continue learning in depth.
Remember, the most important thing in learning programming is to practice a lot. So, quickly open your Python editor and try out these techniques you've learned today! I believe you'll soon become an expert in text mining.
Do you have any thoughts or questions? Feel free to leave a comment, let's discuss and improve together!
Next
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts
Next
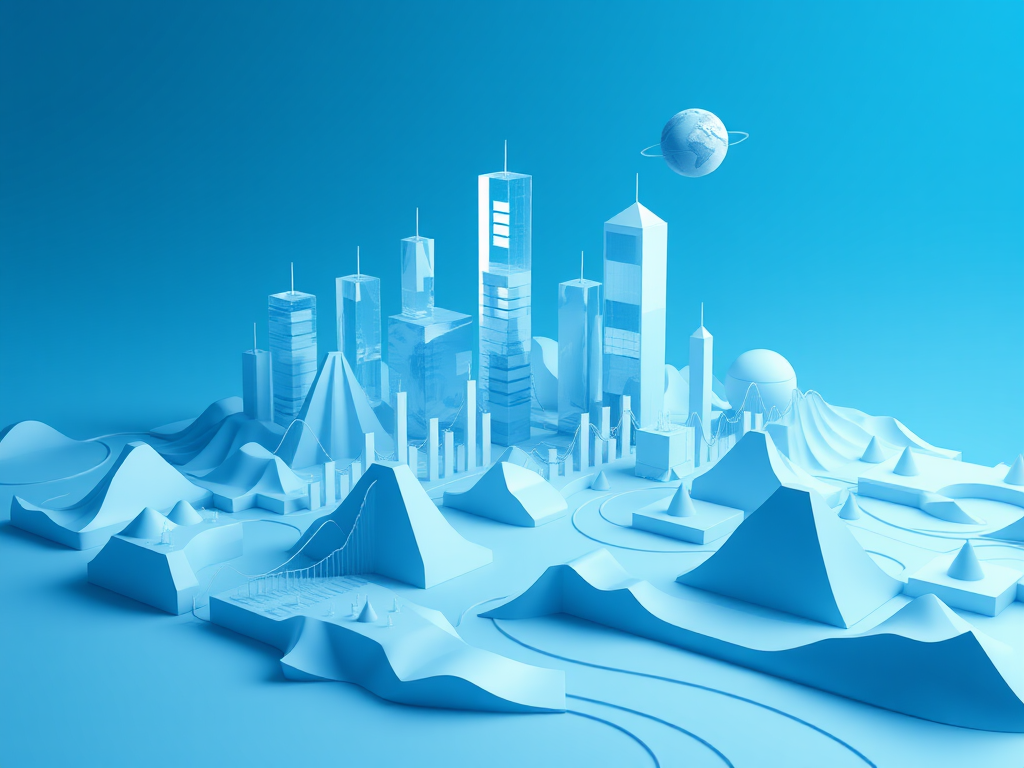
Advanced Python Data Analysis: Elegantly Handling and Visualizing Millions of Data Points
A comprehensive guide to Python data analysis, covering analytical processes, NumPy calculations, Pandas data processing, and Matplotlib visualization techniques, helping readers master practical data analysis tools and methods
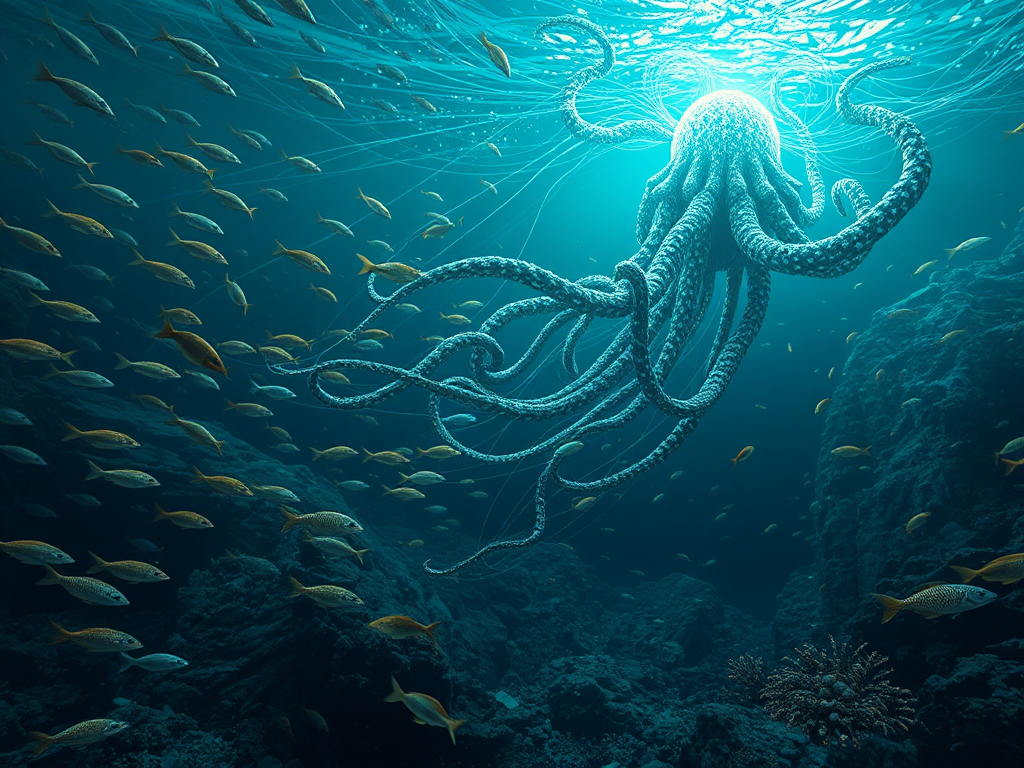
Start here to easily master Python data analysis techniques!
This article introduces common techniques and optimization strategies in Python data analysis, including code optimization, big data processing, data cleaning,
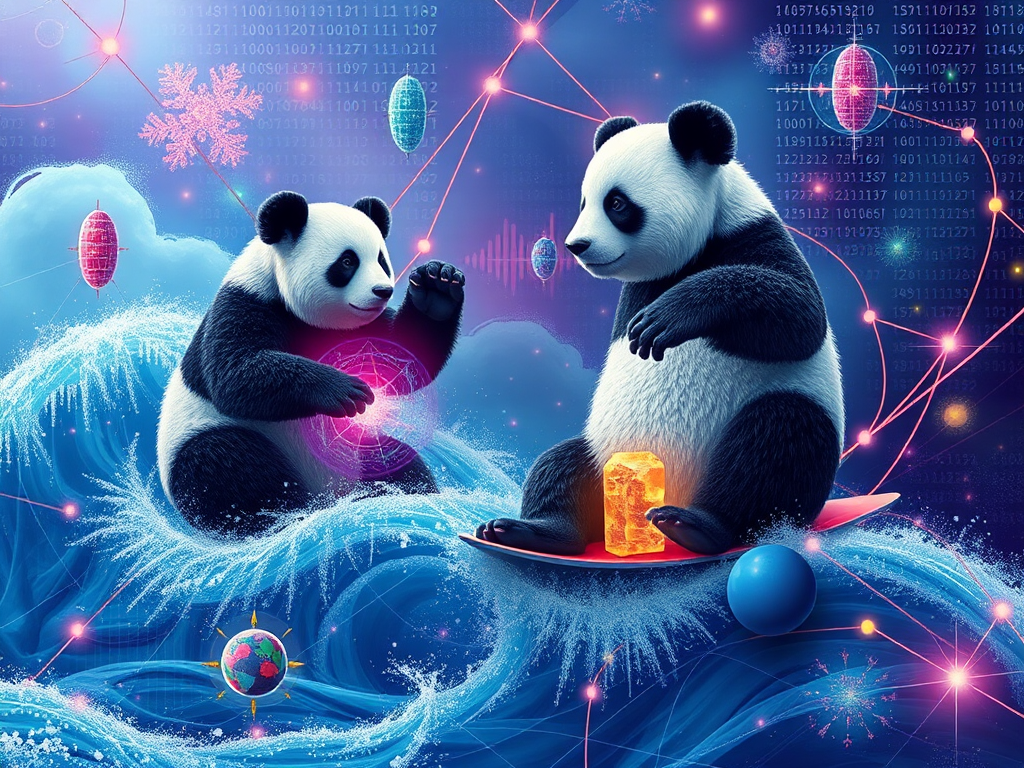
Python Data Analysis: From Basics to Advanced, Unlocking the Magical World of Data Processing
This article delves into Python data analysis techniques, covering the use of libraries like Pandas and NumPy, time series data processing, advanced Pandas operations, and data visualization methods, providing a comprehensive skill guide for data analysts