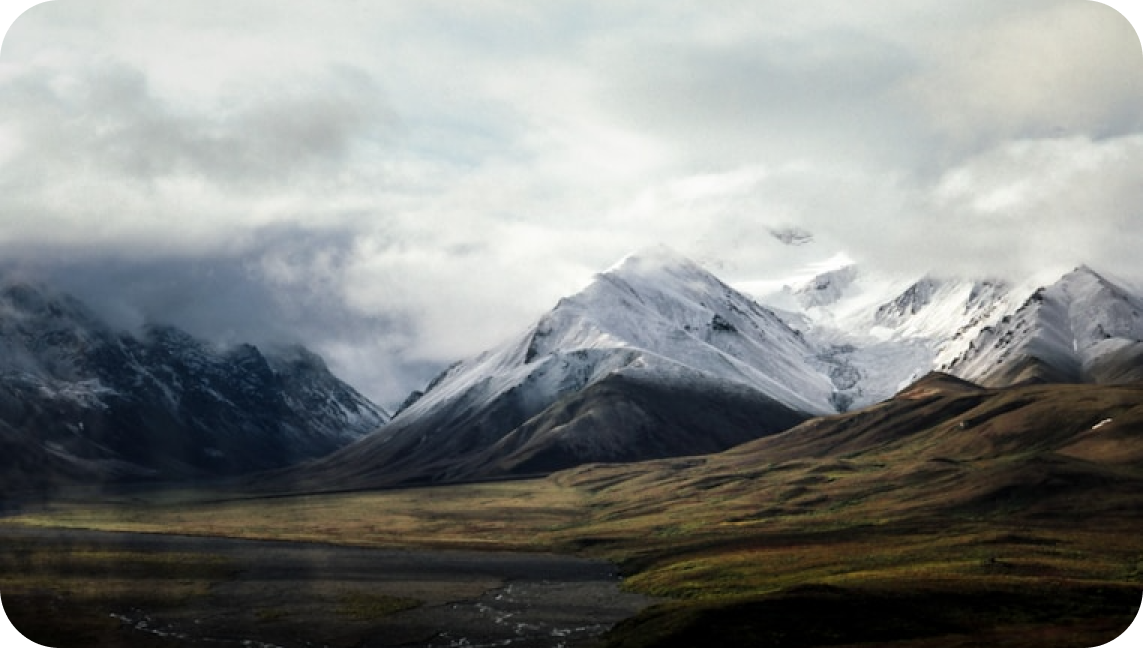
Hello, Python enthusiasts! Today, let's talk about the important and interesting topic of Python package management. As a Python programmer, have you ever been overwhelmed by package management? Don't worry, let's dive into this topic together. From basic concepts to advanced techniques, I'll guide you through the ins and outs of Python package management in simple and understandable language.
The Essence of Packages
First, we need to understand what a package is. Simply put, a package is a collection of related Python modules. But this definition might still be a bit abstract, so let's use a metaphor:
Imagine you're building a house. Modules are like the rooms in the house, and the package is the entire house. Each room (module) has a specific function, like the kitchen is for cooking, and the bedroom is for sleeping. The whole house (package) organizes these functions together to form a complete living space.
In Python, a module is usually a .py file containing definitions of functions, classes, or variables. A package is a directory containing multiple modules, along with a special init.py file to indicate that this directory is a package.
You might ask, why do we need packages? Imagine if your project keeps growing and all the code is in one file. What would that be like? Exactly, like piling all the furniture in one room—messy and hard to find. Packages allow us to organize related functions together, making the code structure clearer and easier to manage and reuse.
Package Management Tools
When it comes to package management, we must mention pip and PyPI. Pip is Python's standard package management tool, while PyPI (Python Package Index) is Python's official third-party library index.
Imagine PyPI as a huge library with hundreds of thousands of books (packages). Pip is your librarian; you tell it which book you need, and it finds and installs it on your computer.
Using pip is very simple. For example, if you want to install the famous requests library, just enter in the command line:
pip install requests
Pip will automatically download and install the requests library and all its dependencies from PyPI. Isn't that convenient?
However, as your Python journey continues, you may encounter some challenges. For instance, different projects may require different versions of the same library, or you might want to try a new library without affecting the existing environment. This is where virtual environments come into play.
Virtual Environments: Your Private Lab
Virtual environments create an independent little world for your Python. In this world, you can freely install, uninstall, and upgrade various packages without affecting other projects or system environments.
Creating and using virtual environments is very simple. Take virtualenv for example:
virtualenv myenv
source myenv/bin/activate # On Linux or macOS
myenv\Scripts\activate.bat # On Windows
deactivate
The benefits of using virtual environments are obvious. It's like preparing a separate workspace for each project, where you can experiment freely without worrying about affecting other projects.
Dependency Management: The Magic of requirements.txt
In team collaboration or project deployment, we often need to ensure everyone uses the same version of dependency packages. This is where the requirements.txt file becomes crucial.
The requirements.txt file lists all the packages and their versions needed for the project. Creating this file is simple:
pip freeze > requirements.txt
This command writes all the installed packages and their versions in the current environment to the requirements.txt file.
When you need to reinstall these dependencies in a new environment, just use:
pip install -r requirements.txt
Pip will automatically install all the packages listed in the file. It's like equipping your project with a "recipe," allowing anyone to accurately replicate the same environment.
Advanced Tools: Pipenv and Poetry
As your projects become more complex, you might find the pip+virtualenv combination somewhat inadequate. At this point, more advanced tools like Pipenv and Poetry can come in handy.
Pipenv combines the functions of pip and virtualenv, providing a simpler workflow. It automatically creates and manages virtual environments for your project, using Pipfile and Pipfile.lock instead of requirements.txt for more precise dependency locking.
Poetry goes a step further, not only managing dependencies but also helping you build and publish your own packages. It uses a pyproject.toml file to manage project configurations and dependencies, offering a more modern package management experience.
These tools make Python package management more powerful and flexible. It's like upgrading from using simple toolboxes to a full set of professional tools, allowing you to better handle complex project requirements.
The Security of Package Management
When it comes to package management, we must mention security. When installing third-party packages, we need to be extra careful, as malicious packages can cause severe harm to our systems.
A few simple principles can help improve security:
- Only install packages from trusted sources (like the official PyPI).
- Before installing, check the package's download count and last update time. Popular and frequently updated packages are usually more reliable.
- Review the package documentation and source code to understand its functionality and authorship.
- Consider using tools like pip-audit to check for known security vulnerabilities in installed packages.
Remember, security is an ongoing process, and we need to remain vigilant.
Resolving Package Conflicts
In actual development, package conflicts are common. This usually happens when different packages depend on different versions of the same library. There are several ways to resolve this issue:
- Use virtual environments: Create separate environments for different projects to avoid global package conflicts.
- Carefully check dependencies: Before installing a new package, check if it's compatible with existing packages.
- Consider using Docker: Docker can provide completely isolated environments for each application, thoroughly avoiding package conflicts.
Remember, prevention is better than cure. Establishing good dependency management habits early in the project can help you avoid many potential conflicts.
The Future of Package Management
As the Python ecosystem continues to evolve, package management tools are also advancing. We can expect to see smarter dependency resolution algorithms, better version control strategies, and stronger security features.
At the same time, with the development of AI and machine learning, we might see AI-assisted package management tools that can automatically analyze project needs and recommend the most suitable packages and versions.
Conclusion
Python package management is a complex yet fascinating topic. From simple pip install to complex dependency resolution, from basic virtual environments to advanced package management tools, each step helps us better organize and manage our Python projects.
As Python developers, mastering package management skills not only makes our development process smoother but also helps us build more robust and maintainable systems. So, don't be afraid to try new tools and technologies, because every learning experience adds new branches to your Python skill tree.
Do you have any experiences or questions about Python package management? Feel free to share your thoughts in the comments! Let's explore and grow together.
Remember, in the world of Python, package management is like building a Lego castle. Each package is a block, and our task is to find the most suitable blocks and perfectly combine them to create our ideal program. Let's create more wonderful works in this world full of possibilities!
Next
The Ultimate Guide to Python Package Management: From Beginner to Expert
An in-depth look at Python package management, covering package definitions, major tools like pip, Pipenv, and Poetry, and the importance of virtual environments. The article also discusses best practices and security considerations in package management.
Python Package Management from Beginner to Practitioner
This article provides a detailed introduction to the basics of Python package management, including everyday use of pip, creating and managing virtual environme
Unveiling Python Package Management: From Beginner to Expert
This article delves into various aspects of Python package management, covering basic pip usage, creating and using virtual environments, working with PyPI, version management and dependency handling, and how to create and distribute your own Python packages. It serves as a comprehensive guide to package management for Python developers.
Next
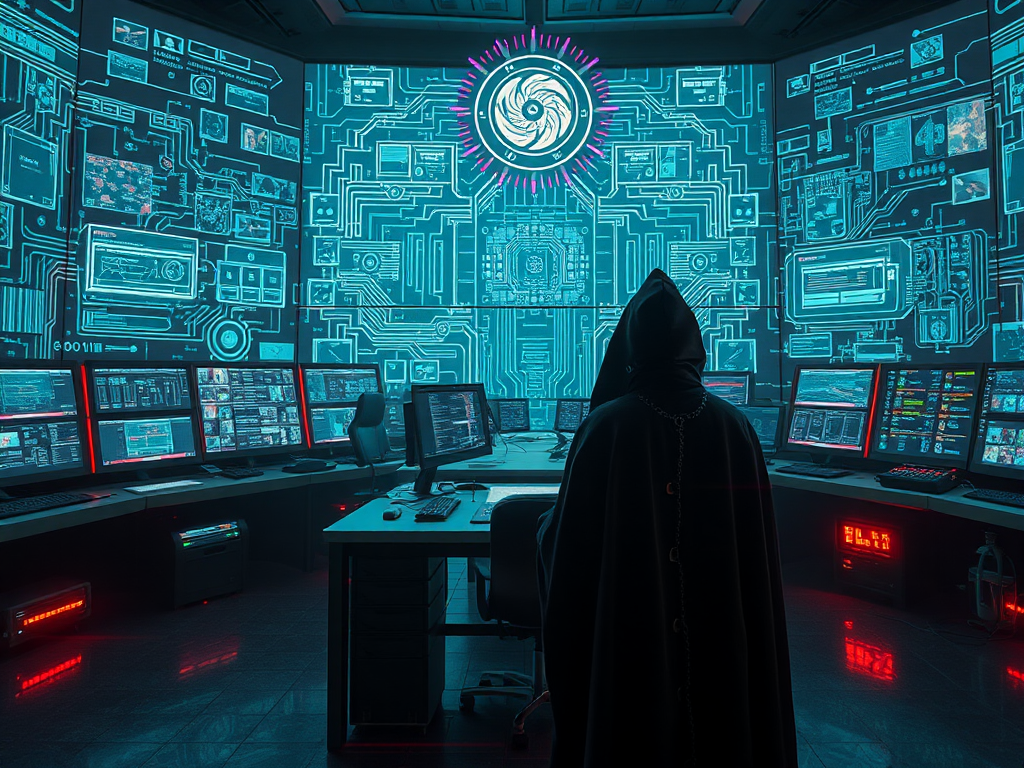
The Ultimate Guide to Python Package Management: From Beginner to Expert
An in-depth look at Python package management, covering package definitions, major tools like pip, Pipenv, and Poetry, and the importance of virtual environments. The article also discusses best practices and security considerations in package management.
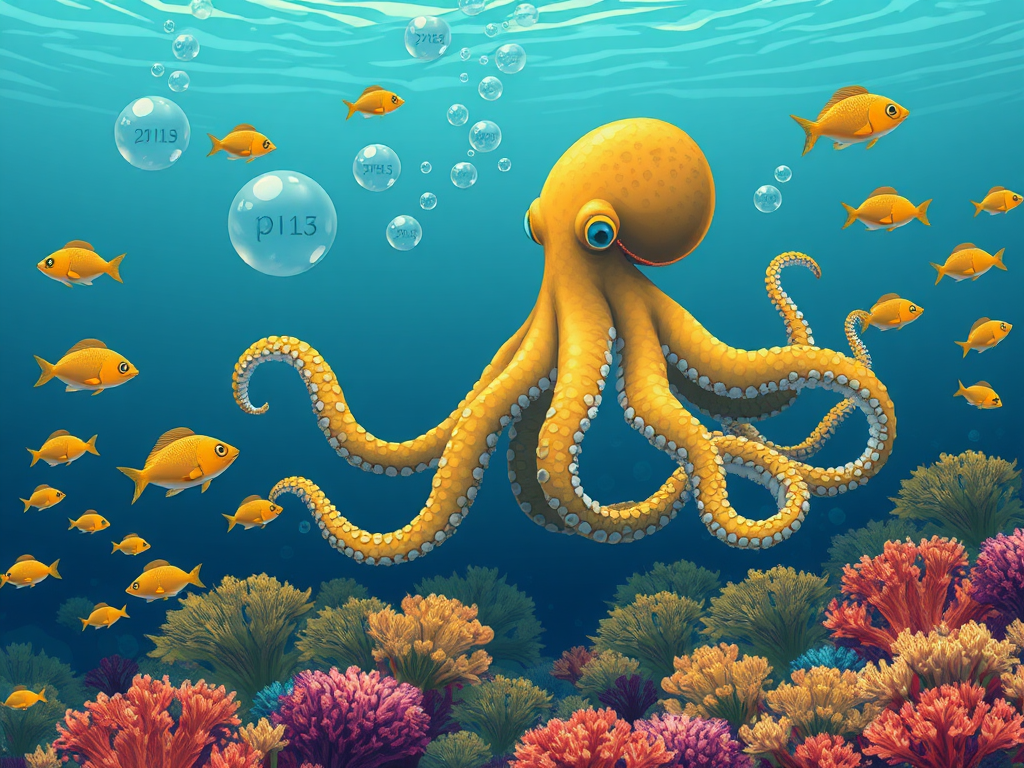
Python Package Management from Beginner to Practitioner
This article provides a detailed introduction to the basics of Python package management, including everyday use of pip, creating and managing virtual environme
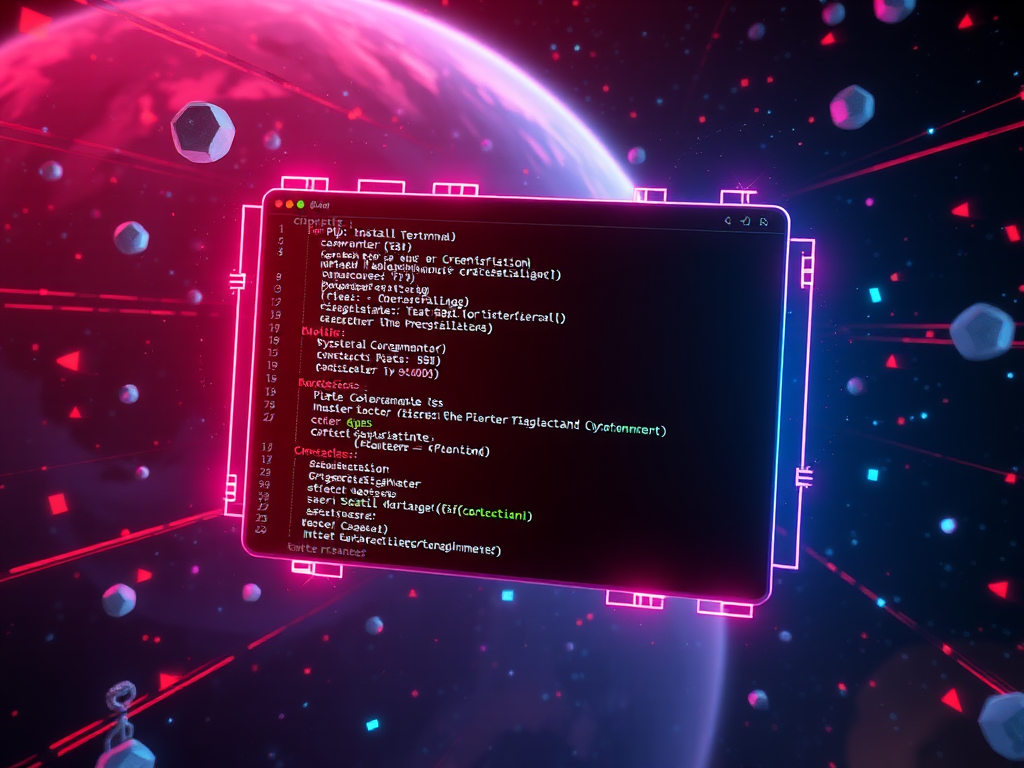
Unveiling Python Package Management: From Beginner to Expert
This article delves into various aspects of Python package management, covering basic pip usage, creating and using virtual environments, working with PyPI, version management and dependency handling, and how to create and distribute your own Python packages. It serves as a comprehensive guide to package management for Python developers.