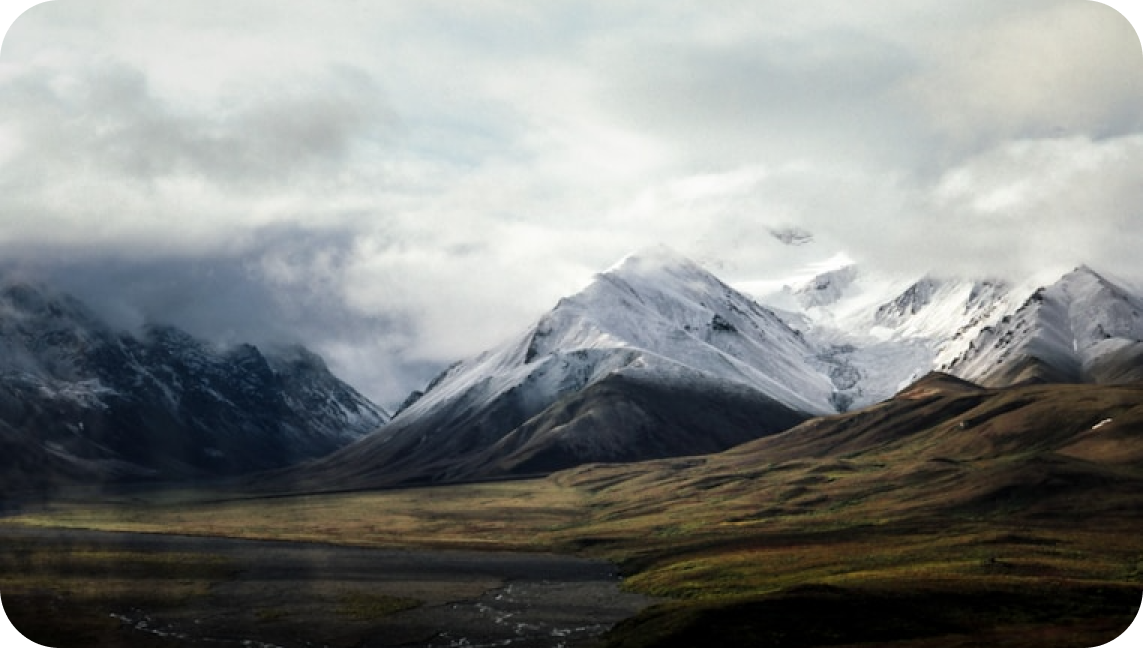
Hello, Python enthusiasts! Today, let's dive into a crucial yet often overlooked topic in the Python world - package management. As a Python developer, you probably deal with various packages daily, but do you really understand the mechanisms behind them? Let's explore this fascinating world together!
The Essence of Packages
First, we need to clarify what a "package" is. Simply put, a package is a collection of related Python modules. But this definition might be a bit abstract, so let's use a real-life example:
Imagine you are packing a suitcase for a trip. Your clothes, shoes, toiletries, etc., are like modules in Python, and the suitcase is a package. This package organizes all related items, making them easy to access whenever needed.
In Python, a package is typically a directory containing an __init__.py
file. This file can be empty, and its presence tells Python that the directory should be treated as a package.
my_package/
__init__.py
module1.py
module2.py
subpackage/
__init__.py
module3.py
This structure allows us to import modules from the package using the dot notation, like:
from my_package import module1
from my_package.subpackage import module3
How do you feel about this organization? Doesn't it make the code structure much clearer?
The Importance of Package Management
So why is package management so important? Imagine what our Python world would be like without it:
- Dependency Hell: Every project would require manually downloading and installing all dependencies, leading to frequent version conflicts.
- Difficulty in Code Reuse: Without a unified sharing and installation mechanism, excellent code would be hard to distribute.
- Maintenance Nightmare: When a library needs updating, all projects using it would need manual updates.
Package management tools solve these problems, allowing us to easily install, update, and delete packages, manage dependencies, and ensure isolation between different projects. It's like having a smart butler managing all your "suitcases," ensuring every "trip" (project) has the right "items" (dependencies).
pip: Python's Swiss Army Knife
When it comes to Python package management, pip is indispensable. Pip is the standard package management tool for Python, and its name is a recursive acronym for "Pip Installs Packages." With pip, you can easily install various packages from the Python Package Index (PyPI).
Let's look at some basic pip commands:
pip install requests
pip install requests==2.25.1
pip install --upgrade requests
pip uninstall requests
pip list
pip show requests
Aren't these commands intuitive? Personally, I particularly like the pip show
command, which lets me quickly find out basic information about a package, including its version, author, and license.
However, pip also has its limitations. For example, it doesn't handle complex dependencies well and can't create isolated environments. This leads us to our next topic: virtual environments.
Virtual Environments: A Private Space for Projects
Virtual environments are like creating an independent "room" for each project, where each "room" has its own Python interpreter and set of packages. This way, different projects won't interfere with each other.
Python 3.3 and later have the built-in venv module, which is very simple to use:
python -m venv myenv
myenv\Scripts\activate
source myenv/bin/activate
deactivate
There are many benefits to using virtual environments:
- Isolated Dependencies: Each project can have its own versions of dependencies without affecting others.
- Easy Reproduction: Easily replicate the project environment on other machines.
- Clean Testing Environment: Quickly create a clean environment to test your packages.
I remember once using a new version of a library in a project, only to find it incompatible with another project. Thanks to virtual environments, I could use different versions of the library in different projects, solving the problem!
Advanced Package Management Tools
While pip and venv can meet most needs, sometimes we might need more powerful tools. Here are a few commonly used advanced package management tools:
Pipenv
Pipenv combines the functionalities of pip and virtualenv, providing a unified command-line interface for managing dependencies and virtual environments.
pip install pipenv
pipenv install requests
pipenv shell
pipenv run python script.py
A great feature of Pipenv is that it uses Pipfile
and Pipfile.lock
to manage dependencies, which is more flexible and precise than the traditional requirements.txt
.
Poetry
Poetry is another powerful dependency management tool that not only manages dependencies but also helps you build and publish packages.
pip install poetry
poetry new my-project
poetry add requests
poetry run python script.py
A highlight of Poetry is its very intelligent dependency resolver, which can automatically handle complex dependencies and version conflicts.
Conda
Conda is not only a package manager but also an environment manager. It was initially designed for data science but is now usable for general Python development.
conda create --name myenv python=3.8
conda activate myenv
conda install numpy pandas
conda deactivate
A unique aspect of Conda is that it can manage non-Python packages, which is especially useful for handling complex scientific computing libraries.
Best Practices for Package Management
From years of Python development experience, I've summarized some best practices for package management that I hope will be helpful to you:
-
Always use virtual environments: This prevents global environment pollution and facilitates project migration and deployment.
-
Keep dependency lists updated: Whether you use
requirements.txt
,Pipfile
, orpyproject.toml
, keep them updated. -
Specify dependency versions: Use exact version numbers or version ranges to avoid unexpected problems due to dependency updates.
-
Regularly update dependencies: But be careful to test and ensure that updates won't break existing functionality.
-
Use
pip freeze
to generate a precise list of dependencies: This ensures that others can exactly replicate your environment. -
Consider using Docker: For complex projects, Docker can provide more thorough environment isolation.
-
Pay attention to security: Regularly check if your dependencies have known security vulnerabilities. Tools like
safety
can help.
pip install safety
safety check
- Follow license regulations: When using open-source packages, be sure to comply with their license requirements.
Have you ever encountered problems due to not following these best practices? Feel free to share your experiences in the comments!
Conclusion
The Python package management ecosystem is so rich and diverse that we've only scratched the surface today. Each tool has its unique advantages, and the choice depends on your specific needs and project characteristics.
Remember, good package management not only improves your development efficiency but also makes your projects more robust and maintainable. It's like establishing a well-organized library in your Python world, allowing you to easily find and use every "book" you need.
So, how do you manage your Python packages? Do you have any unique tips or experiences? Feel free to share your thoughts in the comments!
Let's explore the mysteries of Python package management together and become better Python developers!
Next
The Ultimate Guide to Python Package Management: From Beginner to Expert
An in-depth look at Python package management, covering package definitions, major tools like pip, Pipenv, and Poetry, and the importance of virtual environments. The article also discusses best practices and security considerations in package management.
Python Package Management from Beginner to Practitioner
This article provides a detailed introduction to the basics of Python package management, including everyday use of pip, creating and managing virtual environme
Unveiling Python Package Management: From Beginner to Expert
This article delves into various aspects of Python package management, covering basic pip usage, creating and using virtual environments, working with PyPI, version management and dependency handling, and how to create and distribute your own Python packages. It serves as a comprehensive guide to package management for Python developers.
Next
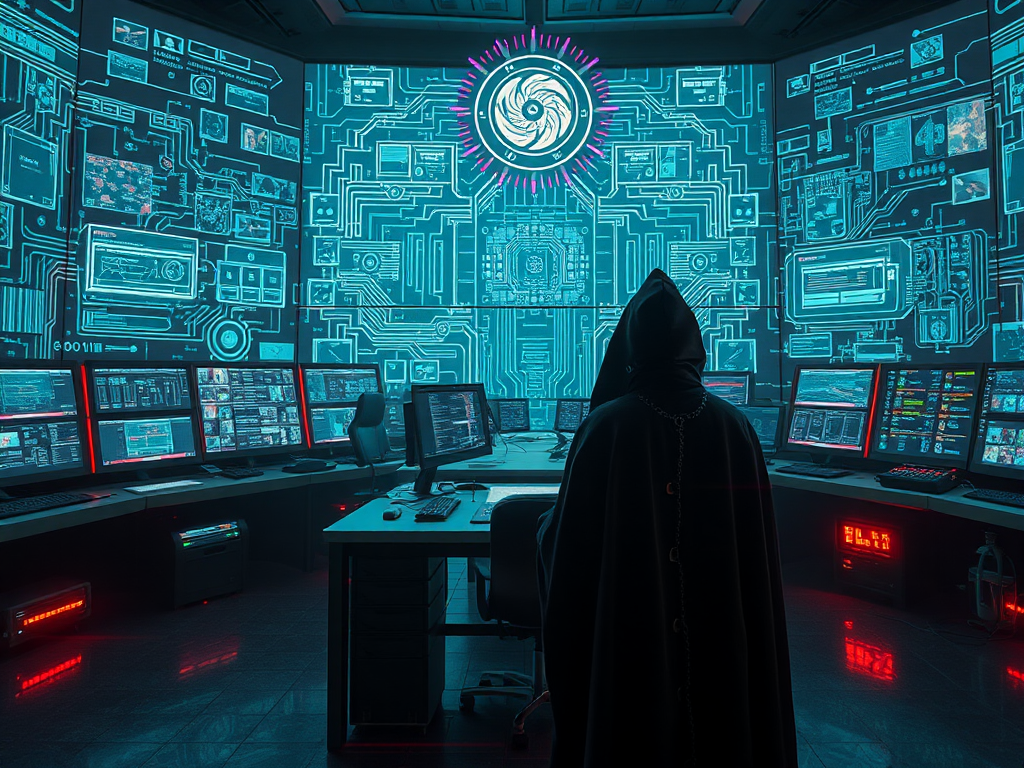
The Ultimate Guide to Python Package Management: From Beginner to Expert
An in-depth look at Python package management, covering package definitions, major tools like pip, Pipenv, and Poetry, and the importance of virtual environments. The article also discusses best practices and security considerations in package management.
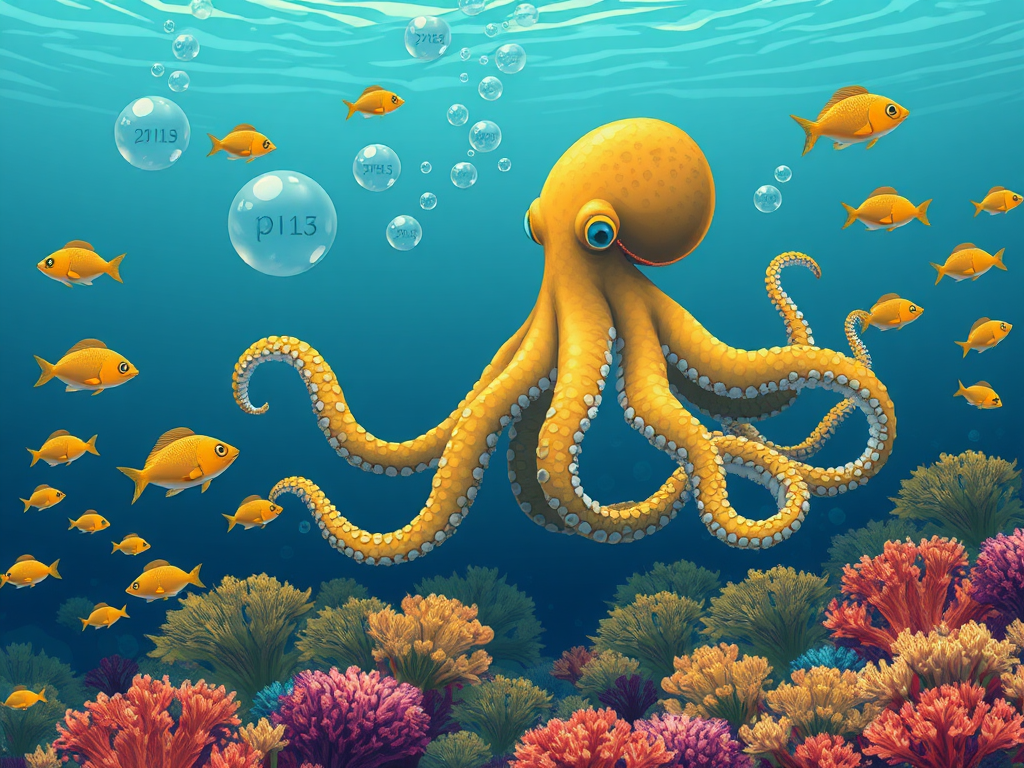
Python Package Management from Beginner to Practitioner
This article provides a detailed introduction to the basics of Python package management, including everyday use of pip, creating and managing virtual environme
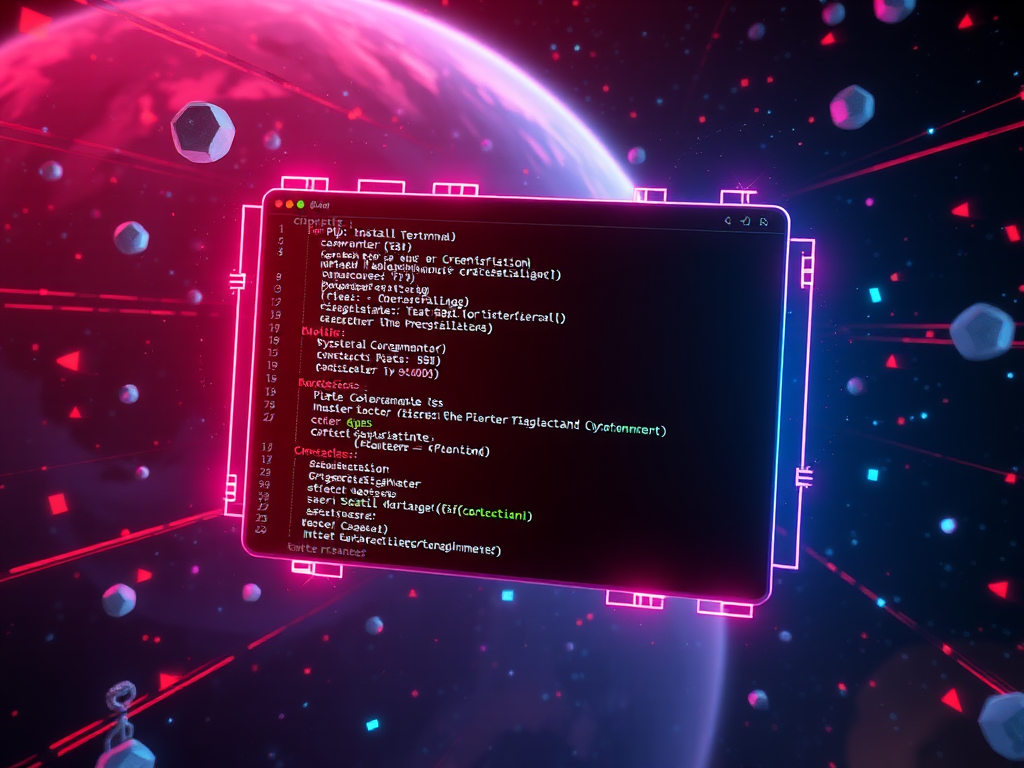
Unveiling Python Package Management: From Beginner to Expert
This article delves into various aspects of Python package management, covering basic pip usage, creating and using virtual environments, working with PyPI, version management and dependency handling, and how to create and distribute your own Python packages. It serves as a comprehensive guide to package management for Python developers.